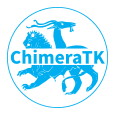 |
ChimeraTK-ApplicationCore
04.01.00
|
Go to the documentation of this file.
14 const std::unordered_set<std::string>& tags)
32 if(_owner !=
nullptr) {
35 if(other._owner !=
nullptr) {
36 other._owner->unregisterModule(&other);
38 _owner = other._owner;
39 other._owner =
nullptr;
41 if(_owner !=
nullptr) {
42 _owner->registerModule(
this,
false);
58 ChimeraTK::ReadAnyGroup group;
59 for(
auto& accessor : recursiveAccessorList) {
60 if(accessor.getDirection() ==
VariableDirection{VariableDirection::feeding, false}) {
63 group.add(accessor.getAppAccessorNoType());
75 for(
auto& accessor : recursiveAccessorList) {
79 if(includeReturnChannels) {
80 if(accessor.getDirection() ==
VariableDirection{VariableDirection::feeding, false}) {
89 accessor.getAppAccessorNoType().read();
92 for(
auto& accessor : recursiveAccessorList) {
100 accessor.getAppAccessorNoType().readLatest();
108 for(
auto& accessor : recursiveAccessorList) {
112 if(includeReturnChannels) {
113 if(accessor.getDirection() ==
VariableDirection{VariableDirection::feeding, false}) {
122 accessor.getAppAccessorNoType().readNonBlocking();
124 for(
auto& accessor : recursiveAccessorList) {
132 accessor.getAppAccessorNoType().readLatest();
140 for(
auto& accessor : recursiveAccessorList) {
141 if(includeReturnChannels) {
142 if(accessor.getDirection() ==
VariableDirection{VariableDirection::feeding, false}) {
151 accessor.getAppAccessorNoType().readLatest();
160 for(
auto& accessor : recursiveAccessorList) {
161 if(includeReturnChannels) {
162 if(accessor.getDirection() ==
VariableDirection{VariableDirection::consuming, false}) {
171 accessor.getAppAccessorNoType().write(versionNumber);
180 for(
auto& accessor : recursiveAccessorList) {
181 if(includeReturnChannels) {
182 if(accessor.getDirection() ==
VariableDirection{VariableDirection::consuming, false}) {
191 accessor.getAppAccessorNoType().writeDestructively(versionNumber);
200 assert(ret !=
nullptr);
205 assert(ret !=
nullptr);
210 assert(owningModule !=
nullptr);
211 return owningModule->findApplicationModule();
213 throw ChimeraTK::logic_error(
214 "EntityOwner::findApplicationModule() called on neither an ApplicationModule nor a VariableGroup.");
230 if(ownerDescription.empty()) {
234 return ownerDescription;
259 auto m = acc.getModel();
std::list< VariableNetworkNode > getAccessorListRecursive() const
Obtain the list of accessors/variables associated with this instance and any submodules.
Module * findApplicationModule()
Find ApplicationModule owner.
Struct to define the direction of variables.
Generic module to read an XML config file and provide the defined values as constant variables.
static ConfigReader & appConfig()
Obtain the ConfigReader instance of the application.
virtual void run()
Execute the module.
EntityOwner * getOwner() const
~Module() override
Destructor.
std::string getFullDescription() const override
Obtain the full description including the full description of the owner.
Base class for owners of other EntityOwners (e.g.
void readAllNonBlocking(bool includeReturnChannels=false)
Just call readNonBlocking() on all readable variables in the group.
virtual std::list< EntityOwner * > getInputModulesRecursively(std::list< EntityOwner * > startList)=0
Use pointer to the module as unique identifier.
std::string _description
The description of this instance.
std::list< Module * > getSubmoduleList() const
Obtain the list of submodules associated with this instance.
void registerModule(Module *module, bool addTags=true)
Register another module as a sub-module.
static Application & getInstance()
Obtain instance of the application.
ConfigReader & getConfigReader()
ChimeraTK::ReadAnyGroup readAnyGroup()
Create a ChimeraTK::ReadAnyGroup for all readable variables in this Module.
std::list< EntityOwner * > getInputModulesRecursively(std::list< EntityOwner * > startList) override
Use pointer to the module as unique identifier.
void writeAllDestructively(bool includeReturnChannels=false)
Just call writeDestructively() on all writable variables in the group.
std::string raiseIftrailingSlash(const std::string &name, bool isModule)
Raises logic error if name ends in a slash, or if it contains consecutive slashes.
std::list< VariableNetworkNode > getAccessorList() const
Obtain the list of accessors/variables directly associated with this instance.
virtual std::string getQualifiedName() const =0
Get the fully qualified name of the module instance, i.e.
EntityOwner * _owner
Owner of this instance.
void readAll(bool includeReturnChannels=false)
Read all readable variables in the group.
void writeAll(bool includeReturnChannels=false)
Just call write() on all writable variables in the group.
EntityOwner & operator=(EntityOwner &&other) noexcept
Move assignment operator.
Module()=default
Default constructor: Allows late initialisation of modules (e.g.
VersionNumber getCurrentVersionNumber() const override
Return the current version number which has been received with the last push-type read operation.
std::string getQualifiedName() const override
Get the fully qualified name of the module instance, i.e.
virtual ModuleType getModuleType() const =0
Return the module type of this module, or in case of a VirtualModule the module type this VirtualModu...
std::string _name
The name of this instance.
void readAllLatest(bool includeReturnChannels=false)
Just call readLatest() on all readable variables in the group.
virtual void unregisterModule(Module *module)
Unregister another module as a sub-module.
Module & operator=(Module &&other) noexcept
Move assignment operator.
InvalidityTracer application module.
virtual std::string getFullDescription() const =0
Obtain the full description including the full description of the owner.
void disable()
Disable the module such that it is not part of the Application.
std::atomic< bool > _testableModeReached
Flag used by the testable mode to identify whether a thread within the EntityOwner has reached the po...
Base class for ApplicationModule and DeviceModule, to have a common interface for these module types.