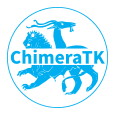 |
ChimeraTK-ApplicationCore
04.01.00
|
Go to the documentation of this file.
8 #include <unordered_set>
27 using TAGS =
const std::unordered_set<std::string>;
30 std::string
negateTag(
const std::string& tag);
42 EntityOwner(std::string name, std::string description, std::unordered_set<std::string> tags = {});
112 void addTag(
const std::string& tag);
115 void dump(
const std::string& prefix =
"", std::ostream& stream = std::cout)
const;
184 std::unordered_set<std::string>
_tags;
202 static std::atomic<uint64_t> uid(0);
203 return std::string(
namePrefixConstant) +
"/" + DataType(
typeid(T)).getAsString() +
"/" + std::to_string(uid++) +
std::list< VariableNetworkNode > getAccessorListRecursive() const
Obtain the list of accessors/variables associated with this instance and any submodules.
Class describing a node of a variable network.
bool hasReachedTestableMode()
Check whether this module has declared that it reached the testable mode.
EntityOwner(EntityOwner &&other) noexcept
Move constructor.
std::string getQualifiedNameWithType() const
Get the fully qualified name of the module instance, followed by the C++ data type (in pointy bracket...
virtual size_t getCircularNetworkHash() const =0
Get the ID of the circular dependency network (0 if none).
void dump(const std::string &prefix="", std::ostream &stream=std::cout) const
Print the full hierarchy to given stream.
const std::unordered_set< std::string > TAGS
Convenience type definition which can optionally be used as a shortcut for the type which defines a l...
static constexpr std::string_view namePrefixConstant
Prefix for constants created by constant().
Base class for owners of other EntityOwners (e.g.
std::string negateTag(const std::string &tag)
negate tag using prefix '!'
EntityOwner()
Default constructor just for late initialisation.
std::string constant(T value)
Create a variable name which will be automatically connected with a constant value.
std::list< Module * > _moduleList
List of modules owned by this instance.
virtual std::list< EntityOwner * > getInputModulesRecursively(std::list< EntityOwner * > startList)=0
Use pointer to the module as unique identifier.
std::unordered_set< std::string > _tags
List of tags to be added to all accessors and modules inside this module.
std::string _description
The description of this instance.
const std::string & getDescription() const
Get the description of the module instance.
std::list< Module * > getSubmoduleList() const
Obtain the list of submodules associated with this instance.
void registerModule(Module *module, bool addTags=true)
Register another module as a sub-module.
void registerAccessor(VariableNetworkNode accessor)
Called inside the constructor of Accessor: adds the accessor to the list.
virtual VersionNumber getCurrentVersionNumber() const =0
Return the current version number which has been received with the last push-type read operation.
std::string escapeName(const std::string &name, bool allowDotsAndSlashes)
Convert all characters which are not allowed in variable or module names into underscores followed by...
virtual void decrementDataFaultCounter()=0
Decrement the fault counter and set the data validity flag to ok if the counter has reached 0.
void addTag(const std::string &tag)
Add a tag to all Application-type nodes inside this group.
virtual DataValidity getDataValidity() const =0
Return the data validity flag.
std::list< VariableNetworkNode > getAccessorList() const
Obtain the list of accessors/variables directly associated with this instance.
virtual void setCurrentVersionNumber(VersionNumber versionNumber)=0
Set the current version number.
virtual void incrementDataFaultCounter()=0
Set the data validity flag to fault and increment the fault counter.
virtual std::string getQualifiedName() const =0
Get the fully qualified name of the module instance, i.e.
EntityOwner & operator=(EntityOwner &&other) noexcept
Move assignment operator.
virtual ~EntityOwner()=default
Virtual destructor to make the type polymorphic.
std::list< VariableNetworkNode > _accessorList
List of accessors owned by this instance.
virtual ModuleType getModuleType() const =0
Return the module type of this module, or in case of a VirtualModule the module type this VirtualModu...
std::string _name
The name of this instance.
virtual void unregisterModule(Module *module)
Unregister another module as a sub-module.
InvalidityTracer application module.
const std::string & getName() const
Get the name of the module instance.
std::list< Module * > getSubmoduleListRecursive() const
Obtain the list of submodules associated with this instance and any submodules.
virtual std::string getFullDescription() const =0
Obtain the full description including the full description of the owner.
std::atomic< bool > _testableModeReached
Flag used by the testable mode to identify whether a thread within the EntityOwner has reached the po...
void unregisterAccessor(const VariableNetworkNode &accessor)
Called inside the destructor of Accessor: removes the accessor from the list.
Base class for ApplicationModule and DeviceModule, to have a common interface for these module types.