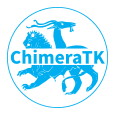 |
ChimeraTK-ApplicationCore
04.01.00
|
Go to the documentation of this file.
14 #ifdef CHIMERATK_APPLICATION_CORE_WITH_PYTHON
18 #include <ChimeraTK/ControlSystemAdapter/ApplicationBase.h>
19 #include <ChimeraTK/DeviceBackend.h>
30 class VariableNetwork;
33 class ApplicationModule;
35 template<
typename UserType>
37 template<
typename UserType>
39 template<
typename UserType>
58 using ApplicationBase::getName;
164 boost::shared_ptr<DeviceManager>
getDeviceManager(
const std::string& aliasOrCDD);
183 #ifdef CHIMERATK_APPLICATION_CORE_WITH_PYTHON
185 return _pythonModuleManager;
205 template<
typename UserType>
208 template<
typename UserType>
276 std::shared_ptr<Logger>
_logger{Logger::getSharedPtr()};
281 #ifdef CHIMERATK_APPLICATION_CORE_WITH_PYTHON
291 template<
typename UserType>
293 template<
typename UserType>
300 throw ChimeraTK::logic_error(
"getCurrentVersionNumber() called on the application. This is probably "
301 "caused by incorrect ownership of variables/accessors or VariableGroups.");
304 throw ChimeraTK::logic_error(
"setCurrentVersionNumber() called on the application. This is probably "
305 "caused by incorrect ownership of variables/accessors or VariableGroups.");
308 throw ChimeraTK::logic_error(
"getDataValidity() called on the application. This is probably "
309 "caused by incorrect ownership of variables/accessors or VariableGroups.");
312 throw ChimeraTK::logic_error(
"incrementDataFaultCounter() called on the application. This is probably "
313 "caused by incorrect ownership of variables/accessors or VariableGroups.");
316 throw ChimeraTK::logic_error(
"decrementDataFaultCounter() called on the application. This is probably "
317 "caused by incorrect ownership of variables/accessors or VariableGroups.");
320 throw ChimeraTK::logic_error(
"getInputModulesRecursively() called on the application. This is probably "
321 "caused by incorrect ownership of variables/accessors or VariableGroups.");
324 throw ChimeraTK::logic_error(
"getCircularNetworkHash() called on the application. This is probably "
325 "caused by incorrect ownership of variables/accessors or VariableGroups.");
void enableTestableMode()
Enable the testable mode.
Class describing a node of a variable network.
std::map< std::string, boost::shared_ptr< DeviceManager > > _deviceManagerMap
Map of DeviceManagers.
ConfigReader * _defaultConfigReader
static void registerThread(const std::string &name)
Register the thread in the application system and give it a name.
Generic module to read an XML config file and provide the defined values as constant variables.
void optimiseUnmappedVariables(const std::set< std::string > &names) override
This class loads and unloads the Python modules as specified in the ConfigReader XML file,...
bool _initialiseCalled
Flag whether initialise() has been called already, to make sure it doesn't get called twice.
The StatusAggregator collects results of multiple StatusMonitor instances and aggregates them into a ...
void shutdown() override
This will remove the global pointer to the instance and allows creating another instance afterwards.
void setCurrentVersionNumber(VersionNumber) override
Set the current version number.
std::atomic< size_t > _dataLossCounter
Counter for how many write() operations have overwritten unread data.
bool _debugDataLoss
Flag whether to debug data loss (as counted with the data loss counter).
VersionNumber _startVersion
Version number used at application start, e.g.
std::atomic< LifeCycleState > _lifeCycleState
Life-cycle state of the application.
Model::RootProxy _model
The model of the application.
void debugMakeConnections()
Enable debug output for the ConnectionMaker.
Model::RootProxy getModel()
Return the root of the application model.
friend struct detail::TestableMode
static void incrementDataLossCounter(const std::string &name)
Increment counter for how many write() operations have overwritten unread data.
void generateXML()
Instead of running the application, just initialise it and output the published variables to an XML f...
std::shared_ptr< Logger > _logger
Shared Pointer to central logger instance.
std::string getQualifiedName() const override
Get the fully qualified name of the module instance, i.e.
std::list< EntityOwner * > getInputModulesRecursively([[maybe_unused]] std::list< EntityOwner * > startList) override
ApplicationModule * getVersionInfoProvider()
std::list< boost::shared_ptr< InternalModule > > _internalModuleList
List of InternalModules.
friend class XMLGeneratorVisitor
FanOut implementation which acts as a read-only (i.e.
void enableDebugDataLoss()
Enable debug output for lost data.
void initialise() override
std::map< size_t, std::list< EntityOwner * > > _circularDependencyNetworks
The networks of circular dependencies, reachable by their hash, which serves as unique ID.
size_t getCircularNetworkHash() const override
Get the ID of the circular dependency network (0 if none).
std::string getFullDescription() const override
Obtain the full description including the full description of the owner.
void run() override
Execute the module.
size_t getCircularNetworkInvalidityCounter(size_t circularNetworkHash) const
detail::CircularDependencyDetector _circularDependencyDetector
friend class VariableNetwork
static Application & getInstance()
Obtain instance of the application.
void generateDOT()
Instead of running the application, just initialise it and output the published variables to a DOT fi...
void setVersionInfoProvider(ApplicationModule *provider)
ConfigReader & getConfigReader()
boost::shared_ptr< DeviceManager > getDeviceManager(const std::string &aliasOrCDD)
Return the DeviceManager for the given alias name or CDD.
void incrementDataFaultCounter() override
Set the data validity flag to fault and increment the fault counter.
friend class VariableNetworkModuleGraphDumpingVisitor
Decorator of the NDRegisterAccessor which facilitates tests of the application.
std::shared_ptr< ConfigReader > _configReader
Manager for Python-based ApplicationModules.
const void * getUniqueId() const
Return the unique ID of this node (will change every time the application is started).
LifeCycleState
Enum to define the life-cycle states of an Application.
std::map< size_t, size_t > _pvIdMap
Map from ProcessArray uniqueId to the variable ID for control system variables.
Decorator of the NDRegisterAccessor which facilitates tests of the application.
friend struct detail::CircularDependencyDetector
std::unordered_set< const void * > _debugMode_variableList
List of variables for which debug output was requested via enableVariableDebugging().
Helper class to facilitate tests of applications based on ApplicationCore.
static bool hasInstance()
Check whether an instance of Application currently exists.
detail::TestableMode _testableMode
bool _testFacilityRunApplicationCalled
Flag which is set by the TestFacility in runApplication() at the beginning.
VersionNumber getCurrentVersionNumber() const override
Return the current version number which has been received with the last push-type read operation.
@ initialisation
Initialisation phase including ApplicationModule::prepare().
bool _runCalled
Flag whether run() has been called already, to make sure it doesn't get called twice.
ConnectionMaker _cm
Helper class to create connections.
void enableVariableDebugging(const VariableNetworkNode &node)
Enable debug output for a given variable.
bool _enableDebugMakeConnections
Flag if debug output is enabled for creation of the variable connections.
Proxy representing the root of the application model.
Base class for several implementations which distribute values from one feeder to multiple consumers.
friend class VariableNetworkGraphDumpingVisitor
DataValidity getDataValidity() const override
Return the data validity flag.
static size_t getAndResetDataLossCounter()
Return the current value of the data loss counter and (atomically) reset it to 0.
std::map< size_t, std::atomic< uint64_t > > _circularNetworkInvalidityCounters
Map of atomic invalidity counters for each circular dependency network.
VersionNumber getStartVersion() const
Return the start version.
std::string _name
The name of this instance.
LifeCycleState getLifeCycleState() const
Get the current LifeCycleState of the application.
ModuleType getModuleType() const override
Return the module type of this module, or in case of a VirtualModule the module type this VirtualModu...
void decrementDataFaultCounter() override
Decrement the fault counter and set the data validity flag to ok if the counter has reached 0.
InvalidityTracer application module.
detail::TestableMode & getTestableMode()
Get the TestableMode control object of this application.
ApplicationModule * _versionInfoProvider
Base class for ApplicationModule and DeviceModule, to have a common interface for these module types.