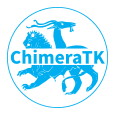 |
ChimeraTK-ApplicationCore
04.01.00
|
Go to the documentation of this file.
71 #include <unordered_map>
73 #include <ChimeraTK/SupportedUserTypes.h>
81 struct ArrayFunctorFill;
82 struct FunctorSetValues;
83 struct FunctorSetValuesArray;
115 const std::unordered_set<std::string>& tags = {});
127 const T&
get(
const std::string& variableName)
const;
134 const T&
get(
const std::string& variableName,
const T& defaultValue)
const;
140 std::list<std::string>
getModules(
const std::string& path = {})
const;
144 void construct(
const std::string& fileName);
159 :
accessor(owner, name,
"unknown",
"Configuration variable"),
value(std::move(theValue)) {}
170 Array(
Module* owner,
const std::string& name,
const std::vector<T>& theValue)
171 :
accessor(owner, name,
"unknown", theValue.size(),
"Configuration array"),
value(theValue) {}
181 void createVar(
const std::string& name,
const std::string& value);
185 void createArray(
const std::string& name,
const std::map<size_t, std::string>& values);
189 void checkVariable(std::string
const& name, std::string
const& type)
const;
193 void checkArray(std::string
const& name, std::string
const& type)
const;
198 using MapOfVar = std::unordered_map<std::string, Var<T>>;
206 using MapOfArray = std::unordered_map<std::string, Array<T>>;
209 ChimeraTK::TemplateUserTypeMapNoVoid<MapOfArray>
_arrayMap;
212 ChimeraTK::SingleTypeUserTypeMapNoVoid<const char*>
_typeMap{
"int8",
"uint8",
"int16",
"uint16",
"int32",
"uint32",
213 "int64",
"uint64",
"float",
"double",
"string",
"boolean"};
219 const T&
getImpl(
const std::string& variableName, T*)
const;
221 const std::vector<T>&
getImpl(
const std::string& variableName, std::vector<T>*)
const;
237 return getImpl(variableName,
static_cast<T*
>(
nullptr));
239 catch(ChimeraTK::logic_error&) {
249 return getImpl(variableName,
static_cast<T*
>(
nullptr));
258 return boost::fusion::at_key<T>(
_variableMap.table).at(variableName).value;
267 return boost::fusion::at_key<T>(
_arrayMap.table).at(variableName).value;
std::unique_ptr< ModuleTree > _moduleTree
List to hold VariableNodes corresponding to xml modules.
Functor to fill variableMap.
void parsingError(const std::string &message)
throw a parsing error with more information
Generic module to read an XML config file and provide the defined values as constant variables.
const T & get(const std::string &variableName) const
Get value for given configuration variable.
ScalarOutput< T > accessor
void createVar(const std::string &name, const std::string &value)
Create an instance of Var<T> and place it on the variableMap.
ConfigReader(ModuleGroup *owner, const std::string &name, const std::string &fileName, const std::unordered_set< std::string > &tags={})
void checkVariable(std::string const &name, std::string const &type) const
Check if variable exists in the config and if type of var name in the config file matches the given t...
void createArray(const std::string &name, const std::map< size_t, std::string > &values)
Create an instance of Array<T> and place it on the arrayMap.
ChimeraTK::TemplateUserTypeMapNoVoid< MapOfArray > _arrayMap
Type-depending map of vectors of arrays.
Functor to fill variableMap for arrays.
Functor to set values to the scalar accessors.
Class holding the value and the accessor for one configuration variable.
void mainLoop() override
To be implemented by the user: function called in a separate thread executing the main loop of the mo...
ArrayOutput< T > accessor
void prepare() override
Prepare the execution of the module.
Array(Module *owner, const std::string &name, const std::vector< T > &theValue)
std::list< std::string > getModules(const std::string &path={}) const
Returns a list of names of modules which are direct children of path.
std::string _fileName
File name.
const T & getImpl(const std::string &variableName, T *) const
Implementation of get() which can be overloaded for scalars and vectors.
ChimeraTK::SingleTypeUserTypeMapNoVoid< const char * > _typeMap
Map assigning string type identifyers to C++ types.
ChimeraTK::TemplateUserTypeMapNoVoid< MapOfVar > _variableMap
Type-depending map of vectors of variables.
std::unordered_map< std::string, Array< T > > MapOfArray
Define type for map of std::string to Array, so we can put it into the TemplateUserTypeMap.
Var(Module *owner, const std::string &name, T theValue)
Class holding the values and the accessor for one configuration array.
void construct(const std::string &fileName)
Helper function to avoid code duplication in constructors.
Functor to set values to the array accessors.
InvalidityTracer application module.
void checkArray(std::string const &name, std::string const &type) const
Check if array exists in the config and if type of array name in the config file matches the given ty...
Base class for ApplicationModule and DeviceModule, to have a common interface for these module types.
std::unordered_map< std::string, Var< T > > MapOfVar
Define type for map of std::string to Var, so we can put it into the TemplateUserTypeMap.