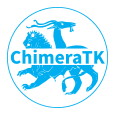 |
ChimeraTK-ApplicationCore
04.01.00
|
Go to the documentation of this file.
12 #include <ChimeraTK/BackendFactory.h>
14 #include <boost/fusion/container/map.hpp>
33 if(_applicationName.empty()) {
35 throw ChimeraTK::logic_error(
"Error: An instance of Application must have its applicationName set.");
40 throw ChimeraTK::logic_error(
41 "Error: The application name may only contain alphanumeric characters and underscores.");
44 #pragma GCC diagnostic push
45 #pragma GCC diagnostic ignored "-Wdeprecated"
46 _configReader = std::make_shared<ConfigReader>(
this,
"/", name +
"-config.xml");
47 #pragma GCC diagnostic pop
51 #ifdef CHIMERATK_APPLICATION_CORE_WITH_PYTHON
53 _pythonModuleManager.createModules(*
this);
55 catch(ChimeraTK::logic_error&) {
57 std::rethrow_exception(std::current_exception());
68 ApplicationBase::shutdown();
99 counter, 0, std::memory_order_release, std::memory_order_relaxed)) {
108 throw ChimeraTK::logic_error(
"Application::initialise() was already called before.");
111 if(!getPVManager()) {
112 throw ChimeraTK::logic_error(
"Application::initialise() was called without an instance of ChimeraTK::PVManager.");
125 throw ChimeraTK::logic_error(
126 "Application::initialise() must be called before Application::optimiseUnmappedVariables().");
135 assert(!_applicationName.empty());
139 throw ChimeraTK::logic_error(
140 "Testable mode enabled but Application::run() called directly. Call TestFacility::runApplication() instead.");
145 throw ChimeraTK::logic_error(
"Application::run() has already been called before.");
170 internalModule->activate();
184 while(!
module->hasReachedTestableMode()) {
193 waitForTestableMode(internalModule.get());
197 waitForTestableMode(
module);
221 internalModule->deactivate();
227 pair.second->terminate();
237 ApplicationBase::shutdown();
245 assert(!_applicationName.empty());
249 generator.save(_applicationName +
".xml");
255 assert(!_applicationName.empty());
262 return dynamic_cast<Application&
>(ApplicationBase::getInstance());
void enableTestableMode()
Enable the testable mode.
std::map< std::string, boost::shared_ptr< DeviceManager > > _deviceManagerMap
Map of DeviceManagers.
ConfigReader * _defaultConfigReader
static void registerThread(const std::string &name)
Register the thread in the application system and give it a name.
@ run
Actual run phase with full multi threading.
void optimiseUnmappedVariables(const std::set< std::string > &names) override
bool _initialiseCalled
Flag whether initialise() has been called already, to make sure it doesn't get called twice.
void shutdown() override
This will remove the global pointer to the instance and allows creating another instance afterwards.
std::atomic< size_t > _dataLossCounter
Counter for how many write() operations have overwritten unread data.
bool _debugDataLoss
Flag whether to debug data loss (as counted with the data loss counter).
std::atomic< LifeCycleState > _lifeCycleState
Life-cycle state of the application.
@ shutdown
The application is in the process of shutting down.
Model::RootProxy _model
The model of the application.
Model::RootProxy getModel()
Return the root of the application model.
static void incrementDataLossCounter(const std::string &name)
Increment counter for how many write() operations have overwritten unread data.
void generateXML()
Instead of running the application, just initialise it and output the published variables to an XML f...
Base class for owners of other EntityOwners (e.g.
std::list< boost::shared_ptr< InternalModule > > _internalModuleList
List of InternalModules.
void writeGraphViz(const std::string &filename, Args... args) const
Implementations of RootProxy.
void initialise() override
Generate XML representation of variables.
void run() override
Execute the module.
bool checkName(const std::string &name, bool allowDotsAndSlashes)
Check given name for characters which are not allowed in variable or module names.
detail::CircularDependencyDetector _circularDependencyDetector
static Application & getInstance()
Obtain instance of the application.
Logger::StreamProxy logger(Logger::Severity severity, std::string context)
Convenience function to obtain the logger stream.
void generateDOT()
Instead of running the application, just initialise it and output the published variables to a DOT fi...
boost::shared_ptr< DeviceManager > getDeviceManager(const std::string &aliasOrCDD)
Return the DeviceManager for the given alias name or CDD.
void finalise()
Finalise the model and register all PVs with the control system adapter.
std::shared_ptr< ConfigReader > _configReader
Manager for Python-based ApplicationModules.
void connect()
Realise connections.
detail::TestableMode _testableMode
bool _testFacilityRunApplicationCalled
Flag which is set by the TestFacility in runApplication() at the beginning.
@ initialisation
Initialisation phase including ApplicationModule::prepare().
bool _runCalled
Flag whether run() has been called already, to make sure it doesn't get called twice.
ConnectionMaker _cm
Helper class to create connections.
bool _enableDebugMakeConnections
Flag if debug output is enabled for creation of the variable connections.
Proxy representing the root of the application model.
void optimiseUnmappedVariables(const std::set< std::string > &names)
Execute the optimisation request from the control system adapter (remove unused variables)
static size_t getAndResetDataLossCounter()
Return the current value of the data loss counter and (atomically) reset it to 0.
VersionNumber getStartVersion() const
Return the start version.
void setDebugConnections(bool enable)
InvalidityTracer application module.
std::list< Module * > getSubmoduleListRecursive() const
Obtain the list of submodules associated with this instance and any submodules.
detail::TestableMode & getTestableMode()
Get the TestableMode control object of this application.