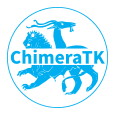 |
ChimeraTK-ApplicationCore
04.01.00
|
Go to the documentation of this file.
7 #include <boost/core/demangle.hpp>
19 : _name(std::move(name)), _description(std::move(description)), _tags(std::move(tags)) {}
24 : _name(
"**INVALID**"), _description(
"Invalid EntityOwner created by default constructor just as a place holder") {}
29 _name = std::move(other._name);
30 _description = std::move(other._description);
31 _accessorList = std::move(other._accessorList);
32 _moduleList = std::move(other._moduleList);
33 _tags = std::move(other._tags);
34 for(
auto* mod : _moduleList) {
37 for(
auto& node : _accessorList) {
38 node.setOwningModule(
this);
41 other._name =
"**INVALID**";
42 other._description =
"This EntityOwner was moved from.";
43 assert(other._accessorList.empty());
44 assert(other._moduleList.empty());
45 assert(other._tags.empty());
54 for(
const auto& tag :
_tags) {
75 auto sublist = submodule->getAccessorListRecursive();
76 list.insert(list.end(), sublist.begin(), sublist.end());
89 auto sublist = submodule->getSubmoduleListRecursive();
90 list.insert(list.end(), sublist.begin(), sublist.end());
98 for(
const auto& tag :
_tags) {
110 stream <<
"==== Hierarchy dump of module '" <<
_name <<
"':" << std::endl;
114 stream << prefix <<
"+ ";
119 stream << prefix <<
"| " << submodule->getName() << std::endl;
120 submodule->dump(prefix +
"| ", stream);
131 submodule->addTag(tag);
148 if(!tag.empty() && tag[0] ==
'!') {
149 return tag.substr(1);
157 return getQualifiedName() +
"<" + boost::core::demangle(
typeid(*this).name()) +
">";
std::list< VariableNetworkNode > getAccessorListRecursive() const
Obtain the list of accessors/variables associated with this instance and any submodules.
Class describing a node of a variable network.
bool hasReachedTestableMode()
Check whether this module has declared that it reached the testable mode.
std::string getQualifiedNameWithType() const
Get the fully qualified name of the module instance, followed by the C++ data type (in pointy bracket...
void dump(const std::string &prefix="", std::ostream &stream=std::cout) const
Print the full hierarchy to given stream.
Base class for owners of other EntityOwners (e.g.
std::string negateTag(const std::string &tag)
negate tag using prefix '!'
EntityOwner()
Default constructor just for late initialisation.
std::list< Module * > _moduleList
List of modules owned by this instance.
std::unordered_set< std::string > _tags
List of tags to be added to all accessors and modules inside this module.
std::list< Module * > getSubmoduleList() const
Obtain the list of submodules associated with this instance.
void registerModule(Module *module, bool addTags=true)
Register another module as a sub-module.
void registerAccessor(VariableNetworkNode accessor)
Called inside the constructor of Accessor: adds the accessor to the list.
void addTag(const std::string &tag)
Add a tag to all Application-type nodes inside this group.
void addTag(const std::string &tag) const
Add a tag.
std::list< VariableNetworkNode > getAccessorList() const
Obtain the list of accessors/variables directly associated with this instance.
virtual std::string getQualifiedName() const =0
Get the fully qualified name of the module instance, i.e.
EntityOwner & operator=(EntityOwner &&other) noexcept
Move assignment operator.
std::list< VariableNetworkNode > _accessorList
List of accessors owned by this instance.
std::string _name
The name of this instance.
virtual void unregisterModule(Module *module)
Unregister another module as a sub-module.
InvalidityTracer application module.
std::list< Module * > getSubmoduleListRecursive() const
Obtain the list of submodules associated with this instance and any submodules.
std::atomic< bool > _testableModeReached
Flag used by the testable mode to identify whether a thread within the EntityOwner has reached the po...
Base class for ApplicationModule and DeviceModule, to have a common interface for these module types.