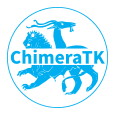 |
ChimeraTK-ApplicationCore
04.01.00
|
Go to the documentation of this file.
7 #include <ChimeraTK/Device.h>
8 #include <ChimeraTK/ForwardDeclarations.h>
9 #include <ChimeraTK/RegisterPath.h>
11 #include <boost/thread/latch.hpp>
45 std::function<void(ChimeraTK::Device&)> initialisationHandler =
nullptr,
const std::string& pathInDevice =
"/");
78 boost::weak_ptr<DeviceManager>
_dm;
void addInitialisationHandler(std::function< void(ChimeraTK::Device &)> initialisationHandler)
Implementation class for the model.
void reportException(std::string errMsg)
Use this function to report an exception.
SetDMapFilePath(const std::string &dmapFilePath)
ModuleGroup()=default
Default constructor to allow late initialisation of module groups.
std::string _pathInDevice
boost::weak_ptr< DeviceManager > _dm
The corresponding DeviceManager.
Implements access to a ChimeraTK::Device.
DeviceManager & getDeviceManager()
Return the corresponding DeviceManager.
Helper class to set the DMAP file path.
Model::DeviceModuleProxy _model
DeviceModule & operator=(DeviceModule &&other) noexcept
Move assignment.
const std::string & getDeviceAliasOrURI() const
friend class DeviceModule
Model::DeviceModuleProxy getModel()
DeviceModule(DeviceModule &&other) noexcept
Move operation with the move constructor.
InvalidityTracer application module.
const std::string & getTriggerPath() const