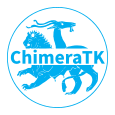 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
16 _flags(std::move(flags)) {}
31 template<
typename UserType>
32 boost::shared_ptr<NDRegisterAccessor<UserType>>
getAccessor(boost::shared_ptr<LogicalNameMappingBackend> backend,
33 size_t numberOfWords,
size_t wordOffsetInRegister,
AccessModeFlags flags,
size_t pluginIndex) {
35 getAccessor_impl, UserType, backend, numberOfWords, wordOffsetInRegister, flags, pluginIndex);
39 boost::shared_ptr<LogicalNameMappingBackend>,
size_t,
size_t,
AccessModeFlags,
size_t));
72 virtual void openHook(
const boost::shared_ptr<LogicalNameMappingBackend>& backend) { std::ignore = backend; }
78 virtual void postParsingHook([[maybe_unused]]
const boost::shared_ptr<const LogicalNameMappingBackend>& backend) {}
103 template<
typename Derived>
141 template<
typename UserType,
typename TargetType>
143 boost::shared_ptr<LogicalNameMappingBackend>& backend,
147 template<
typename UserType>
149 boost::shared_ptr<LogicalNameMappingBackend>& backend,
size_t numberOfWords,
size_t wordOffsetInRegister,
162 const std::string& name,
const std::map<std::string, std::string>& parameters);
172 const LNMBackendRegisterInfo& info,
size_t pluginIndex,
const std::map<std::string, std::string>& parameters);
177 template<
typename UserType,
typename TargetType>
179 boost::shared_ptr<LogicalNameMappingBackend>& backend,
196 template<
typename UserType,
typename TargetType>
198 boost::shared_ptr<LogicalNameMappingBackend>& backend,
210 const LNMBackendRegisterInfo& info,
size_t pluginIndex,
const std::map<std::string, std::string>& parameters);
214 template<
typename UserType,
typename TargetType>
216 boost::shared_ptr<LogicalNameMappingBackend>& backend,
224 const LNMBackendRegisterInfo& info,
size_t pluginIndex,
const std::map<std::string, std::string>& parameters);
228 template<
typename UserType,
typename TargetType>
230 boost::shared_ptr<LogicalNameMappingBackend>& backend,
238 const LNMBackendRegisterInfo& info,
size_t pluginIndex,
const std::map<std::string, std::string>& parameters);
249 const LNMBackendRegisterInfo& info,
size_t pluginIndex,
const std::map<std::string, std::string>& parameters);
251 template<
typename UserType,
typename TargetType>
253 boost::shared_ptr<LogicalNameMappingBackend>& backend,
269 template<
typename Derived>
272 :
AccessorPluginBase(info), _needSharedTarget{shareTargetAccessors}, _pluginIndex(pluginIndex) {
278 template<
typename Derived>
279 template<
typename UserType,
typename TargetType>
283 if constexpr(std::is_same<UserType, TargetType>::value) {
295 template<
typename Derived>
296 template<
typename UserType>
298 boost::shared_ptr<LogicalNameMappingBackend>& backend,
size_t numberOfWords,
size_t wordOffsetInRegister,
300 boost::shared_ptr<NDRegisterAccessor<UserType>> decorated;
302 assert(_pluginIndex == pluginIndex);
305 auto type = getTargetDataType(
typeid(UserType));
306 if((_info._dataDescriptor.rawDataType() == DataType::none) && flags.
has(AccessMode::raw)) {
308 "Access mode 'raw' is not supported for register '" + std::string(_info.getRegisterName()) +
"'");
314 if(_needSharedTarget) {
315 auto& map = boost::fusion::at_key<decltype(T)>(backend->sharedAccessorMap.table);
320 auto it = map.find(key);
321 if(it == map.end() || (target = it->second.accessor.lock()) ==
nullptr) {
323 target = backend->getRegisterAccessor_impl<decltype(T)>(
324 _info.getRegisterName(), numberOfWords, wordOffsetInRegister, flags, pluginIndex + 1);
325 map[key].accessor = target;
329 target = backend->getRegisterAccessor_impl<decltype(T)>(
330 _info.getRegisterName(), numberOfWords, wordOffsetInRegister, flags, pluginIndex + 1);
334 UndecoratedParams accessorParams(_info.registerName, numberOfWords, wordOffsetInRegister, flags);
335 decorated =
static_cast<Derived*
>(
this)->
template decorateAccessor<UserType>(backend, target, accessorParams);
338 decorated->setExceptionBackend(backend);
boost::shared_ptr< AccessorPluginBase > makePlugin(LNMBackendRegisterInfo info, size_t pluginIndex, const std::string &name, const std::map< std::string, std::string > ¶meters)
Factory function for accessor plugins.
std::pair< DeviceBackend *, RegisterPath > AccessorKey
Map of target accessors which are potentially shared across our accessors.
boost::shared_ptr< NDRegisterAccessor< UserType > > decorateAccessor(boost::shared_ptr< LogicalNameMappingBackend > &backend, boost::shared_ptr< NDRegisterAccessor< TargetType >> &target, const UndecoratedParams &accessorParams)
DataType getTargetDataType(DataType) const override
Return the data type for which the target accessor shall be obtained.
boost::shared_ptr< NDRegisterAccessor< UserType > > getAccessor_impl(boost::shared_ptr< LogicalNameMappingBackend > &backend, size_t numberOfWords, size_t wordOffsetInRegister, AccessModeFlags flags, size_t pluginIndex)
This function is called by the backend.
UndecoratedParams(const std::string &name, size_t numberOfWords, size_t wordOffsetInRegister, AccessModeFlags flags)
void doRegisterInfoUpdate() override
Implementation of the plugin specific register information update.
boost::shared_ptr< NDRegisterAccessor< UserType > > decorateAccessor(boost::shared_ptr< LogicalNameMappingBackend > &backend, boost::shared_ptr< NDRegisterAccessor< TargetType >> &target, const UndecoratedParams &accessorParams)
@ float64
Double precision float.
virtual DataType getTargetDataType(DataType userType) const
Return the data type for which the target accessor shall be obtained.
ForcePollingRead Plugin: Forces a register to not allow setting the AccessMode::wait_for_new_data fla...
Base class for plugins that modify the behaviour of accessors in the logical name mapping backend.
BitRangeAccessPlugin(const LNMBackendRegisterInfo &info, size_t pluginIndex, const std::map< std::string, std::string > ¶meters)
virtual void postParsingHook([[maybe_unused]] const boost::shared_ptr< const LogicalNameMappingBackend > &backend)
Hook called after the parsing of logical name map.
virtual ~AccessorPluginBase()=default
boost::shared_ptr< NDRegisterAccessor< UserType > > decorateAccessor(boost::shared_ptr< LogicalNameMappingBackend > &backend, boost::shared_ptr< NDRegisterAccessor< TargetType >> &target, const UndecoratedParams &accessorParams)
This function should be overridden by the plugin (yes, this is possible due to the CRTP).
void doRegisterInfoUpdate() override
Implementation of the plugin specific register information update.
uint32_t dataInterpretationFractionalBits
size_t _wordOffsetInRegister
TypeHintModifier Plugin: Change the catalogue type of the mapped register.
Base class for AccessorPlugins used by the LogicalNameMapping backend to store backends in lists.
ForceReadOnly Plugin: Forces a register to be read only.
void doRegisterInfoUpdate() override
Implementation of the plugin specific register information update.
virtual void openHook(const boost::shared_ptr< LogicalNameMappingBackend > &backend)
Hook called when the backend is opened, at the end of the open() function after all backend work has ...
#define FILL_VIRTUAL_FUNCTION_TEMPLATE_VTABLE(functionName)
Fill the vtable of a virtual function template defined with DEFINE_VIRTUAL_FUNCTION_TEMPLATE.
MonostableTriggerPlugin(LNMBackendRegisterInfo info, size_t pluginIndex, const std::map< std::string, std::string > ¶meters)
void setAltSeparator(const std::string &altSeparator)
set alternative separator.
boost::shared_ptr< NDRegisterAccessor< UserType > > getAccessor(boost::shared_ptr< LogicalNameMappingBackend > backend, size_t numberOfWords, size_t wordOffsetInRegister, AccessModeFlags flags, size_t pluginIndex)
Called by the backend when obtaining a register accessor.
DEFINE_VIRTUAL_FUNCTION_TEMPLATE_VTABLE(getAccessor_impl, boost::shared_ptr< NDRegisterAccessor< T >>(boost::shared_ptr< LogicalNameMappingBackend >, size_t, size_t, AccessModeFlags, size_t))
void updateRegisterInfo(BackendRegisterCatalogue< LNMBackendRegisterInfo > &)
Update the register info inside the catalogue if needed.
AccessorPluginBase(const LNMBackendRegisterInfo &info)
Helper struct to hold extra parameters needed by some plugins, used in decorateAccessor()
Interface for backends to the register catalogue.
bool has(AccessMode flag) const
Check if a certain flag is in the set.
@ uint32
Unsigned 32 bit integer.
boost::shared_ptr< NDRegisterAccessor< UserType > > decorateAccessor(boost::shared_ptr< LogicalNameMappingBackend > &backend, boost::shared_ptr< NDRegisterAccessor< TargetType >> &target, const UndecoratedParams &accessorParams)
virtual void closeHook()
Hook called when the backend is closed, at the beginning of the close() function when the device is s...
RegisterInfo structure for the LogicalNameMappingBackend.
MultiplierPlugin(const LNMBackendRegisterInfo &info, size_t pluginIndex, const std::map< std::string, std::string > ¶meters)
@ none
The data type/concept does not exist, e.g. there is no raw transfer (do not confuse with Void)
void doRegisterInfoUpdate() override
Implementation of the plugin specific register information update.
LNMBackendRegisterInfo _info
RegisterInfo describing the the target register for which this plugin instance should work.
virtual void doRegisterInfoUpdate()=0
Implementation of the plugin specific register information update.
Multiplier Plugin: Multiply register's data with a constant factor.
A class to describe which of the supported data types is used.
void doRegisterInfoUpdate() override
Implementation of the plugin specific register information update.
DataType getTargetDataType(DataType) const override
Return the data type for which the target accessor shall be obtained.
bool dataInterpretationIsSigned
boost::shared_ptr< NDRegisterAccessor< UserType > > decorateAccessor(boost::shared_ptr< LogicalNameMappingBackend > &backend, boost::shared_ptr< NDRegisterAccessor< TargetType >> &target, const UndecoratedParams &accessorParams)
DataType getTargetDataType(DataType) const override
Return the data type for which the target accessor shall be obtained.
void doRegisterInfoUpdate() override
Implementation of the plugin specific register information update.
ForcePollingReadPlugin(const LNMBackendRegisterInfo &info, size_t pluginIndex, const std::map< std::string, std::string > ¶meters)
TypeHintModifierPlugin(const LNMBackendRegisterInfo &info, size_t pluginIndex, const std::map< std::string, std::string > ¶meters)
void callForType(const std::type_info &type, LAMBDATYPE lambda)
Helper function for running code which uses some compile-time type that is specified at runtime as a ...
virtual void exceptionHook()
Hook called when an exception is reported to the the backend via setException(), after the backend ha...
Class to store a register path name.
AccessorPlugin(const LNMBackendRegisterInfo &info, size_t pluginIndex, bool shareTargetAccessors=false)
The constructor of the plugin should also accept a 3rd argument: const std::map<std::string,...
boost::shared_ptr< NDRegisterAccessor< UserType > > decorateAccessor(boost::shared_ptr< LogicalNameMappingBackend > &backend, boost::shared_ptr< NDRegisterAccessor< TargetType >> &target, const UndecoratedParams &accessorParams)
const bool _needSharedTarget
Deriving plugins should set this to true if they want to use interlocked access to the same target ac...
Monostable Trigger Plugin: Write value to target which falls back to another value after defined time...
ForceReadOnlyPlugin(const LNMBackendRegisterInfo &info, size_t pluginIndex, const std::map< std::string, std::string > ¶meters)
#define CALL_VIRTUAL_FUNCTION_TEMPLATE(functionName, templateArgument,...)
Execute the virtual function template call using the vtable defined with the DEFINE_VIRTUAL_FUNCTION_...
size_t _pluginIndex
Index of the plugin instance within the stack of plugins on a particular register.
Set of AccessMode flags with additional functionality for an easier handling.
@ uint64
Unsigned 64 bit integer.
Exception thrown when a logic error has occured.