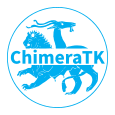 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
7 #include <boost/make_shared.hpp>
19 if(parameters.find(
"milliseconds") == parameters.end()) {
21 "LogicalNameMappingBackend MonostableTriggerPluginPlugin: Missing parameter 'milliseconds'.");
24 if(parameters.find(
"active") != parameters.end()) {
25 _active = std::stoul(parameters.at(
"active"));
27 if(parameters.find(
"inactive") != parameters.end()) {
28 _inactive = std::stoul(parameters.at(
"inactive"));
47 template<
typename UserType>
52 double milliseconds, uint32_t active, uint32_t inactive)
56 if(!_target->isWriteable()) {
58 "LogicalNameMappingBackend MonostableTriggerPluginPlugin: Cannot target non-writeable register.");
60 if(_target->getNumberOfChannels() > 1 || _target->getNumberOfSamples() > 1) {
62 "LogicalNameMappingBackend MonostableTriggerPluginPlugin: Cannot target non-scalar registers.");
66 [[nodiscard]]
bool isReadable()
const override {
return false; }
69 throw ChimeraTK::logic_error(
"LogicalNameMappingBackend MonostableTriggerPluginPlugin: Reading is not allowed.");
75 _target->accessData(0, 0) =
_active;
76 _target->setDataValidity(this->_dataValidity);
87 std::chrono::duration<double, std::milli>
_delay;
95 template<
typename UserType>
101 bool a = _target->writeTransfer(versionNumber);
104 std::this_thread::sleep_for(_delay);
106 _target->accessData(0, 0) = _inactive;
108 dataLossInInactivate = _target->writeTransfer(versionNumber);
109 return a || dataLossInInactivate;
112 template<
typename UserType>
115 return doWriteTransfer(versionNumber);
120 template<
typename UserType,
typename TargetType>
124 if constexpr(std::is_same<TargetType, uint32_t>::value) {
void doPreWrite(TransferType, VersionNumber versionNumber) override
@ raw
Raw access: disable any possible conversion from the original hardware data type into the given UserT...
bool doWriteTransfer(ChimeraTK::VersionNumber versionNumber) override
boost::shared_ptr< NDRegisterAccessor< UserType > > decorateAccessor(boost::shared_ptr< LogicalNameMappingBackend > &backend, boost::shared_ptr< NDRegisterAccessor< TargetType >> &target, const UndecoratedParams &accessorParams)
Base class for plugins that modify the behaviour of accessors in the logical name mapping backend.
void remove(AccessMode flag)
Remove the given flag from the set.
void doRegisterInfoUpdate() override
Implementation of the plugin specific register information update.
bool isReadable() const override
MonostableTriggerPlugin(LNMBackendRegisterInfo info, size_t pluginIndex, const std::map< std::string, std::string > ¶meters)
void doPostWrite(TransferType, VersionNumber versionNumber) override
Helper struct to hold extra parameters needed by some plugins, used in decorateAccessor()
Class describing the actual payload data format of a register in an abstract manner.
bool dataLossInInactivate
std::chrono::duration< double, std::milli > _delay
RegisterInfo structure for the LogicalNameMappingBackend.
void doPostRead(TransferType, bool) override
LNMBackendRegisterInfo _info
RegisterInfo describing the the target register for which this plugin instance should work.
TransferType
Used to indicate the applicable operation on a Transferelement.
MonostableTriggerPluginDecorator(const boost::shared_ptr< ChimeraTK::NDRegisterAccessor< uint32_t >> &target, double milliseconds, uint32_t active, uint32_t inactive)
bool doWriteTransferDestructively(ChimeraTK::VersionNumber versionNumber) override
Base class for decorators of the NDRegisterAccessor.
DataDescriptor _dataDescriptor
AccessModeFlags supportedFlags
Supported AccessMode flags.
Class for generating and holding version numbers without exposing a numeric representation.
void doPreRead(TransferType) override
N-dimensional register accessor.
bool readable
Flag if the register is readable.
Exception thrown when a logic error has occured.