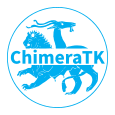 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
9 #include <boost/make_shared.hpp>
28 template<
typename UserType>
35 if(!target->isReadable()) {
37 "LogicalNameMappingBackend ForceReadOnlyPlugin: Cannot target non-readable register.");
41 [[nodiscard]]
bool isWriteable()
const override {
return false; }
54 template<
typename UserType,
typename TargetType>
58 if constexpr(std::is_same<UserType, TargetType>::value) {
59 return boost::make_shared<ForceReadOnlyPluginDecorator<UserType>>(target);
void doRegisterInfoUpdate() override
Implementation of the plugin specific register information update.
Base class for plugins that modify the behaviour of accessors in the logical name mapping backend.
Helper struct to hold extra parameters needed by some plugins, used in decorateAccessor()
void doPreWrite(TransferType, VersionNumber) override
bool writeable
Flag if the register is writeable.
boost::shared_ptr< NDRegisterAccessor< UserType > > decorateAccessor(boost::shared_ptr< LogicalNameMappingBackend > &backend, boost::shared_ptr< NDRegisterAccessor< TargetType >> &target, const UndecoratedParams &accessorParams)
RegisterInfo structure for the LogicalNameMappingBackend.
LNMBackendRegisterInfo _info
RegisterInfo describing the the target register for which this plugin instance should work.
TransferType
Used to indicate the applicable operation on a Transferelement.
ForceReadOnlyPluginDecorator(const boost::shared_ptr< ChimeraTK::NDRegisterAccessor< UserType >> &target)
Base class for decorators of the NDRegisterAccessor.
bool isWriteable() const override
Class for generating and holding version numbers without exposing a numeric representation.
ForceReadOnlyPlugin(const LNMBackendRegisterInfo &info, size_t pluginIndex, const std::map< std::string, std::string > ¶meters)
N-dimensional register accessor.
Exception thrown when a logic error has occured.
void doPostWrite(TransferType, VersionNumber) override