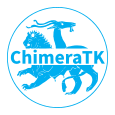 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
9 #include <boost/make_shared.hpp>
16 const LNMBackendRegisterInfo& info,
size_t pluginIndex,
const std::map<std::string, std::string>& parameters)
19 if(parameters.find(
"factor") == parameters.end()) {
22 _factor = std::stod(parameters.at(
"factor"));
34 template<
typename UserType>
54 template<
typename UserType>
56 _target->postRead(type, hasNewData);
57 if(!hasNewData)
return;
58 for(
size_t i = 0; i < _target->getNumberOfChannels(); ++i) {
59 for(
size_t k = 0; k < _target->getNumberOfSamples(); ++k) {
60 buffer_2D[i][k] = numericToUserType<UserType>(_target->accessData(i, k) * _factor);
63 this->_versionNumber = _target->getVersionNumber();
64 this->_dataValidity = _target->dataValidity();
67 template<
typename UserType>
69 for(
size_t i = 0; i < _target->getNumberOfChannels(); ++i) {
70 for(
size_t k = 0; k < _target->getNumberOfSamples(); ++k) {
71 _target->accessData(i, k) = userTypeToNumeric<double>(buffer_2D[i][k]) * _factor;
74 _target->setDataValidity(this->_dataValidity);
75 _target->preWrite(type, versionNumber);
80 template<
typename UserType,
typename TargetType>
84 if constexpr(std::is_same<TargetType, double>::value) {
85 return boost::make_shared<MultiplierPluginDecorator<UserType>>(target,
_factor);
@ raw
Raw access: disable any possible conversion from the original hardware data type into the given UserT...
void doPreWrite(TransferType type, VersionNumber) override
Base class for plugins that modify the behaviour of accessors in the logical name mapping backend.
void doRegisterInfoUpdate() override
Implementation of the plugin specific register information update.
void remove(AccessMode flag)
Remove the given flag from the set.
MultiplierPluginDecorator(const boost::shared_ptr< ChimeraTK::NDRegisterAccessor< double >> &target, double factor)
Helper struct to hold extra parameters needed by some plugins, used in decorateAccessor()
Class describing the actual payload data format of a register in an abstract manner.
void doPostWrite(TransferType type, VersionNumber dataLost) override
RegisterInfo structure for the LogicalNameMappingBackend.
MultiplierPlugin(const LNMBackendRegisterInfo &info, size_t pluginIndex, const std::map< std::string, std::string > ¶meters)
LNMBackendRegisterInfo _info
RegisterInfo describing the the target register for which this plugin instance should work.
void doPostRead(TransferType type, bool hasNewData) override
TransferType
Used to indicate the applicable operation on a Transferelement.
A class to describe which of the supported data types is used.
Base class for decorators of the NDRegisterAccessor.
DataDescriptor _dataDescriptor
void doPreRead(TransferType type) override
AccessModeFlags supportedFlags
Supported AccessMode flags.
boost::shared_ptr< NDRegisterAccessor< UserType > > decorateAccessor(boost::shared_ptr< LogicalNameMappingBackend > &backend, boost::shared_ptr< NDRegisterAccessor< TargetType >> &target, const UndecoratedParams &accessorParams)
Class for generating and holding version numbers without exposing a numeric representation.
N-dimensional register accessor.
Exception thrown when a logic error has occured.