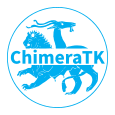 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
7 #include <boost/make_shared.hpp>
11 template<
typename BackendRegisterInfo>
14 template<
typename BackendRegisterInfo>
51 [[nodiscard]]
virtual std::unique_ptr<const_RegisterCatalogueImplIterator>
getConstIteratorBegin()
const = 0;
54 [[nodiscard]]
virtual std::unique_ptr<const_RegisterCatalogueImplIterator>
getConstIteratorEnd()
const = 0;
57 [[nodiscard]]
virtual std::unique_ptr<BackendRegisterCatalogueBase>
clone()
const = 0;
69 template<
typename BackendRegisterInfo>
83 [[nodiscard]] std::unique_ptr<const_RegisterCatalogueImplIterator>
getConstIteratorBegin()
const override;
85 [[nodiscard]] std::unique_ptr<const_RegisterCatalogueImplIterator>
getConstIteratorEnd()
const override;
87 [[nodiscard]] std::unique_ptr<BackendRegisterCatalogueBase>
clone()
const override;
93 void addRegister(
const BackendRegisterInfo& registerInfo);
150 std::map<RegisterPath, BackendRegisterInfo> catalogue;
151 std::vector<BackendRegisterInfo*> insertionOrderedCatalogue;
176 template<
typename BackendRegisterInfo>
177 class const_BackendRegisterCatalogueImplIterator :
public const_RegisterCatalogueImplIterator {
185 const BackendRegisterInfoBase*
get()
override;
188 const std::unique_ptr<const_RegisterCatalogueImplIterator>& rightHandSide)
const override;
190 [[nodiscard]] std::unique_ptr<const_RegisterCatalogueImplIterator>
clone()
const override;
200 const BackendRegisterInfo&
operator*()
const;
202 const BackendRegisterInfo*
operator->()
const;
204 explicit operator RegisterCatalogue::const_iterator()
const;
211 typename std::vector<BackendRegisterInfo*>::const_iterator
theIterator;
217 template<
typename BackendRegisterInfo>
253 template<
typename BackendRegisterInfo>
255 return RegisterInfo(std::make_unique<BackendRegisterInfo>(getBackendRegister(name)));
260 template<
typename BackendRegisterInfo>
264 return catalogue.at(name);
266 catch(std::out_of_range&) {
267 throw ChimeraTK::logic_error(
"BackendRegisterCatalogue::getRegister(): Register '" + name +
"' does not exist.");
273 template<
typename BackendRegisterInfo>
275 return catalogue.find(registerPathName) != catalogue.end();
280 template<
typename BackendRegisterInfo>
282 return catalogue.size();
287 template<
typename BackendRegisterInfo>
289 BackendRegisterInfo>::getConstIteratorBegin()
const {
290 auto it = std::make_unique<const_BackendRegisterCatalogueImplIterator<BackendRegisterInfo>>(
291 insertionOrderedCatalogue.cbegin());
297 template<
typename BackendRegisterInfo>
299 BackendRegisterInfo>::getConstIteratorEnd()
const {
300 auto it = std::make_unique<const_BackendRegisterCatalogueImplIterator<BackendRegisterInfo>>(
301 insertionOrderedCatalogue.cend());
307 template<
typename BackendRegisterInfo>
316 std::unique_ptr<BackendRegisterCatalogueBase> c = std::make_unique<BackendRegisterCatalogue<BackendRegisterInfo>>();
327 template<
typename BackendRegisterInfo>
331 for(
auto& p : catalogue) {
332 target->catalogue[p.first] = getBackendRegister(p.first);
334 for(
auto& ptr : insertionOrderedCatalogue) {
335 target->insertionOrderedCatalogue.push_back(&target->catalogue[ptr->getRegisterName()]);
341 template<
typename BackendRegisterInfo>
343 if(hasRegister(registerInfo.getRegisterName())) {
345 registerInfo.getRegisterName() +
" already exists!");
347 catalogue[registerInfo.getRegisterName()] = registerInfo;
348 insertionOrderedCatalogue.push_back(&catalogue[registerInfo.getRegisterName()]);
353 template<
typename BackendRegisterInfo>
356 if(!hasRegister(name)) {
358 "BackendRegisterCatalogue::removeRegister(): Register '" + name +
"' does not exist.");
362 auto it = std::find_if(insertionOrderedCatalogue.begin(), insertionOrderedCatalogue.end(),
363 [&](
auto reg) { return reg->getRegisterName() == name; });
364 assert(it != insertionOrderedCatalogue.end());
365 insertionOrderedCatalogue.erase(it);
368 auto removed = catalogue.erase(name);
369 assert(removed == 1);
374 template<
typename BackendRegisterInfo>
376 if(!hasRegister(registerInfo.getRegisterName())) {
378 registerInfo.getRegisterName() +
"' cannot be modified because it does not exist!");
380 catalogue[registerInfo.getRegisterName()] = registerInfo;
388 template<
typename BackendRegisterInfo>
390 typename std::vector<BackendRegisterInfo*>::const_iterator it)
395 template<
typename BackendRegisterInfo>
402 template<
typename BackendRegisterInfo>
409 template<
typename BackendRegisterInfo>
416 template<
typename BackendRegisterInfo>
418 const std::unique_ptr<const_RegisterCatalogueImplIterator>& rightHandSide)
const {
420 return rhs_casted && rhs_casted->
theIterator == theIterator;
425 template<
typename BackendRegisterInfo>
427 BackendRegisterInfo>::clone()
const {
428 return {std::make_unique<const_BackendRegisterCatalogueImplIterator>(*
this)};
433 template<
typename BackendRegisterInfo>
435 BackendRegisterInfo>::operator++() {
442 template<
typename BackendRegisterInfo>
444 BackendRegisterInfo>::operator++(int) {
452 template<
typename BackendRegisterInfo>
454 BackendRegisterInfo>::operator--() {
461 template<
typename BackendRegisterInfo>
463 BackendRegisterInfo>::operator--(int) {
471 template<
typename BackendRegisterInfo>
473 return *(*theIterator);
478 template<
typename BackendRegisterInfo>
485 template<
typename BackendRegisterInfo>
488 return rightHandSide.theIterator == theIterator;
493 template<
typename BackendRegisterInfo>
496 return rightHandSide.theIterator != theIterator;
502 template<
typename BackendRegisterInfo>
504 typename std::vector<BackendRegisterInfo*>::iterator theIterator_)
505 : theIterator(theIterator_) {}
509 template<
typename BackendRegisterInfo>
511 BackendRegisterInfo>::operator++() {
518 template<
typename BackendRegisterInfo>
520 BackendRegisterInfo>::operator++(int) {
528 template<
typename BackendRegisterInfo>
530 BackendRegisterInfo>::operator--() {
537 template<
typename BackendRegisterInfo>
539 BackendRegisterInfo>::operator--(int) {
547 template<
typename BackendRegisterInfo>
549 return *(*theIterator);
554 template<
typename BackendRegisterInfo>
561 template<
typename BackendRegisterInfo>
564 boost::make_shared<const_BackendRegisterCatalogueImplIterator<BackendRegisterInfo>>(theIterator)};
569 template<
typename BackendRegisterInfo>
577 template<
typename BackendRegisterInfo>
BackendRegisterCatalogueBase & operator=(BackendRegisterCatalogueBase &&other)=default
Const iterator for iterating through the registers in the catalogue.
virtual std::unique_ptr< const_RegisterCatalogueImplIterator > getConstIteratorEnd() const =0
Return end iterator for iterating through the registers in the catalogue.
virtual size_t getNumberOfRegisters() const =0
Get number of registers in the catalogue.
const_BackendRegisterCatalogueImplIterator< BackendRegisterInfo > end() const
Return const end iterators for iterating through the registers in the catalogue.
std::vector< BackendRegisterInfo * >::const_iterator theIterator
const BackendRegisterInfo * operator->() const
BackendRegisterCatalogueImplIterator & operator--()
const_BackendRegisterCatalogueImplIterator< BackendRegisterInfo > cbegin() const
Return const begin iterators for iterating through the registers in the catalogue.
BackendRegisterCatalogueImplIterator< BackendRegisterInfo > end()
Return end iterator for iterating through the registers in the catalogue.
void modifyRegister(const BackendRegisterInfo ®isterInfo)
Replaces the register information for the matching register.
const BackendRegisterInfo & operator*() const
bool operator==(const BackendRegisterCatalogueImplIterator &rightHandSide) const
std::unique_ptr< BackendRegisterCatalogueBase > clone() const override
Create deep copy of the catalogue.
void decrement() override
BackendRegisterInfo & operator*() const
const_BackendRegisterCatalogueImplIterator(typename std::vector< BackendRegisterInfo * >::const_iterator it)
BackendRegisterCatalogueBase()=default
bool operator==(const const_BackendRegisterCatalogueImplIterator &rightHandSide) const
virtual RegisterInfo getRegister(const RegisterPath ®isterPathName) const =0
Get register information for a given full path name.
std::vector< BackendRegisterInfo * >::iterator theIterator
Interface for backends to the register catalogue.
BackendRegisterInfo * operator->() const
virtual bool hasRegister(const RegisterPath ®isterPathName) const =0
Check if register with the given path name exists.
std::unique_ptr< const_RegisterCatalogueImplIterator > getConstIteratorEnd() const override
Return end iterator for iterating through the registers in the catalogue.
BackendRegisterCatalogueImplIterator()=default
bool operator!=(const const_BackendRegisterCatalogueImplIterator &rightHandSide) const
const_BackendRegisterCatalogueImplIterator & operator++()
void addRegister(const BackendRegisterInfo ®isterInfo)
Add register information to the catalogue.
Non-const iterator for iterating through the registers in the catalogue, used by backend implementati...
RegisterInfo getRegister(const RegisterPath ®isterPathName) const final
Note: Implementation internally uses getBackendRegister(), so no need to override.
const_BackendRegisterCatalogueImplIterator< BackendRegisterInfo > begin() const
Return const begin iterators for iterating through the registers in the catalogue.
std::unique_ptr< const_RegisterCatalogueImplIterator > getConstIteratorBegin() const override
Return begin iterator for iterating through the registers in the catalogue.
const_BackendRegisterCatalogueImplIterator< BackendRegisterInfo > cend() const
Return const end iterators for iterating through the registers in the catalogue.
virtual std::unique_ptr< BackendRegisterCatalogueBase > clone() const =0
Create deep copy of the catalogue.
void fillFromThis(BackendRegisterCatalogue< BackendRegisterInfo > *target) const
Helper function for clone functions.
std::unique_ptr< const_RegisterCatalogueImplIterator > clone() const override
virtual ~BackendRegisterCatalogueBase()=default
void removeRegister(const RegisterPath &name)
Remove register as identified by the given name from the catalogue.
Class to store a register path name.
bool hasRegister(const RegisterPath ®isterPathName) const override
Check if register with the given path name exists.
virtual BackendRegisterInfo getBackendRegister(const RegisterPath ®isterPathName) const
Note: Override this function if backend has "hidden" registers which are not added to the map and hen...
void increment() override
Implementation of the catalogue const iterator which is templated to the actual BackendRegisterInfo i...
DeviceBackend-independent register description.
const_BackendRegisterCatalogueImplIterator & operator--()
bool isEqual(const std::unique_ptr< const_RegisterCatalogueImplIterator > &rightHandSide) const override
virtual std::unique_ptr< const_RegisterCatalogueImplIterator > getConstIteratorBegin() const =0
Return begin iterator for iterating through the registers in the catalogue.
size_t getNumberOfRegisters() const override
Get number of registers in the catalogue.
const BackendRegisterInfoBase * get() override
Exception thrown when a logic error has occured.
Pure virtual implementation base class for the register catalogue.
BackendRegisterCatalogueImplIterator & operator++()
BackendRegisterCatalogueImplIterator< BackendRegisterInfo > begin()
Return begin iterator for iterating through the registers in the catalogue.
bool operator!=(const BackendRegisterCatalogueImplIterator &rightHandSide) const