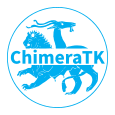 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
9 #include <unordered_set>
25 void close()
override;
30 std::string address, std::map<std::string, std::string> parameters);
38 template<typename UserType>
40 size_t numberOfWords,
size_t wordOffsetInRegister,
AccessModeFlags flags,
size_t omitPlugins = 0);
42 template<typename UserType>
70 template<
typename UserType>
72 boost::weak_ptr<NDRegisterAccessor<UserType>>
accessor;
83 template<
typename UserType>
std::pair< DeviceBackend *, RegisterPath > AccessorKey
Map of target accessors which are potentially shared across our accessors.
std::map< AccessorKey, SharedAccessor< UserType > > SharedAccessorMap
friend class LNMBackendRegisterAccessor
void activateAsyncRead() noexcept override
Activate asyncronous read for all transfer elements where AccessMode::wait_for_new_data is set.
boost::shared_ptr< NDRegisterAccessor< UserType > > getRegisterAccessor_impl(const RegisterPath ®isterPathName, size_t numberOfWords, size_t wordOffsetInRegister, AccessModeFlags flags, size_t omitPlugins=0)
std::recursive_mutex mutex
std::string _lmapFileName
name of the logical map file
LogicalNameMappingBackend(std::string lmapFileName="")
std::atomic< bool > _asyncReadActive
Flag storing whether asynchronous read has been activated.
std::string readDeviceInfo() override
Return a device information string containing hardware details like the firmware version number or th...
boost::weak_ptr< NDRegisterAccessor< UserType > > accessor
bool catalogueCompleted
Flag whether the catalogue has already been filled with extra information from the target backends.
boost::shared_ptr< NDRegisterAccessor< UserType > > getRegisterAccessor_internal(const RegisterPath ®isterPathName, size_t numberOfWords, size_t wordOffsetInRegister, AccessModeFlags flags)
void setExceptionImpl() noexcept override
Function to be (optionally) implemented by backends if additional actions are needed when switching t...
RegisterCatalogue getRegisterCatalogue() const override
Return the register catalogue with detailed information on all registers.
Catalogue of register information.
The base class for backends providing IO functionality for the Device class.
Interface for backends to the register catalogue.
void parse() const
parse the logical map file, if not yet done
std::map< std::string, LNMVariable > _variables
Map of variables and constants.
std::map< std::string, boost::shared_ptr< DeviceBackend > > _devices
map of target devices
BackendRegisterCatalogue< LNMBackendRegisterInfo > _catalogue_mutable
We need to make the catalogue mutable, since we fill it within getRegisterCatalogue()
RegisterInfo structure for the LogicalNameMappingBackend.
void open() override
Open the device.
ChimeraTK::VersionNumber getVersionOnOpen() const
TemplateUserTypeMap< SharedAccessorMap > sharedAccessorMap
Backend to map logical register names onto real hardware registers.
std::unordered_set< std::string > getTargetDevices() const
Obtain list of all target devices referenced in the catalogue.
DeviceBackendImpl implements some basic functionality which should be available for all backends.
bool hasParsed
flag if already parsed
std::mutex sharedAccessorMap_mutex
a mutex to be locked when sharedAccessorMap (the container) is changed
Class to store a register path name.
static boost::shared_ptr< DeviceBackend > createInstance(std::string address, std::map< std::string, std::string > parameters)
Class for generating and holding version numbers without exposing a numeric representation.
Struct holding shared accessors together with a mutex for thread safety.
void close() override
Close the device.
Set of AccessMode flags with additional functionality for an easier handling.
std::map< std::string, std::string > _parameters
map of parameters passed through the CDD
N-dimensional register accessor.