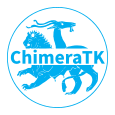 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
9 #include <boost/algorithm/string.hpp>
10 #ifdef CHIMERATK_HAVE_PCIE_BACKEND
13 #ifdef CHIMERATK_HAVE_XDMA_BACKEND
16 #ifdef CHIMERATK_HAVE_UIO_BACKEND
22 #include "DeviceAccessVersion.h"
31 #include <boost/bind/bind.hpp>
32 #include <boost/function.hpp>
46 boost::shared_ptr<DeviceBackend> (*creatorFunction)(
47 std::string address, std::map<std::string, std::string> parameters),
48 const std::vector<std::string>& sdmParameterNames,
const std::string& version) {
50 std::cout <<
"adding:" << backendType << std::endl << std::flush;
55 throw ChimeraTK::logic_error(
"A backend with the type name '" + backendType +
"' has already been registered.");
58 if(version != CHIMERATK_DEVICEACCESS_VERSION) {
64 std::stringstream errorMessage;
65 errorMessage <<
"Backend plugin '" << backendType <<
"' compiled with wrong DeviceAccess version " << version
66 <<
". Please recompile with version " << CHIMERATK_DEVICEACCESS_VERSION;
67 std::string errorMessageString = errorMessage.str();
70 creatorMap_compat[make_pair(backendType,
"")] = [errorMessageString](std::string host, std::string instance,
71 std::list<std::string> parameters, std::string mapFileName) {
73 host, instance, parameters, mapFileName, errorMessageString);
75 creatorMap[backendType] = [errorMessageString](std::string,
76 std::map<std::string, std::string>) -> boost::shared_ptr<ChimeraTK::DeviceBackend> {
85 creatorMap_compat[make_pair(backendType,
"")] = [creatorFunction, sdmParameterNames](std::string,
86 std::string instance, std::list<std::string> parameters,
87 std::string mapFileName) {
89 std::map<std::string, std::string> pars;
91 for(
auto& p : parameters) {
92 if(i >= sdmParameterNames.size())
break;
93 pars[sdmParameterNames[i]] = p;
96 if(!mapFileName.empty()) {
97 if(pars[
"map"].empty()) {
98 pars[
"map"] = mapFileName;
101 std::cout <<
"WARNING: You have specified the map file name twice, in "
102 "the parameter list and in the 3rd "
103 "column of the DMAP file."
105 std::cout <<
"Please only specify the map file name in the parameter list!" << std::endl;
108 return creatorFunction(std::move(instance), pars);
115 boost::shared_ptr<DeviceBackend> (*creatorFunction)(
116 std::string host, std::string instance, std::list<std::string> parameters, std::string mapFileName),
117 const std::string& version) {
119 std::cout <<
"adding:" <<
interface << std::endl << std::flush;
122 if(version != CHIMERATK_DEVICEACCESS_VERSION) {
128 std::stringstream errorMessage;
129 errorMessage <<
"Backend plugin '" <<
interface << "' compiled with wrong DeviceAccess version " << version
130 << ". Please recompile with version " << CHIMERATK_DEVICEACCESS_VERSION;
131 std::string errorMessageString = errorMessage.str();
134 creatorMap_compat[make_pair(interface, protocol)] = [errorMessageString](std::string host, std::string instance,
135 std::list<std::string> parameters,
136 std::string mapFileName) {
138 host, instance, parameters, mapFileName, errorMessageString);
140 creatorMap[interface] = [errorMessageString](std::string,
141 std::map<std::string, std::string>) -> boost::shared_ptr<ChimeraTK::DeviceBackend> {
150 creatorMap[interface] = [interface](std::string,
151 std::map<std::string, std::string>) -> boost::shared_ptr<ChimeraTK::DeviceBackend> {
154 "' does not yet support ChimeraTK device "
155 "descriptors! Please update the backend!");
172 BackendFactory::BackendFactory() {
173 #ifdef CHIMERATK_HAVE_PCIE_BACKEND
176 #ifdef CHIMERATK_HAVE_XDMA_BACKEND
179 #ifdef CHIMERATK_HAVE_UIO_BACKEND
193 std::cout <<
"getInstance" << std::endl << std::flush;
196 return factoryInstance;
202 std::lock_guard<std::mutex> lock(
_mutex);
208 deviceInfo.
uri = aliasOrUri;
224 std::cout <<
"uri to parse" << deviceInfo.
uri << std::endl;
225 std::cout <<
"Entries" <<
creatorMap.size() << std::endl << std::flush;
231 auto strongPtr = iterator->second.lock();
245 catch(std::out_of_range&) {
256 std::cout <<
"Using the SDM descriptor is deprecated. Please change to CDD (ChimeraTK device descriptor)."
262 <<
"Using the device node in a dmap file is deprecated. Please change to CDD (ChimeraTK device descriptor)."
266 if((iter.first.first == sdm.
interface)) {
268 boost::weak_ptr<DeviceBackend> weakBackend = backend;
287 void* hndl = dlopen(soFile.c_str(), RTLD_LAZY);
288 if(hndl ==
nullptr) {
296 "'" + soFile +
"' is not a valid DeviceAccess plugin, it does not register any backends!");
309 for(
const auto& lib : dmap->getPluginLibraries()) {
317 std::cerr <<
"Error: Caught exception loading plugin '" << lib <<
"' specified in dmap file: " << e.
what()
319 std::cerr <<
"Some backends will not be available!" << std::endl;
329 std::string , std::list<std::string> , std::string ,
330 std::string exception_what) {
boost::shared_ptr< DeviceBackend > createBackend(const std::string &aliasOrUri)
Create a new backend and return the instance as a shared pointer.
static boost::shared_ptr< DeviceBackend > createInstance(std::string address, std::map< std::string, std::string > parameters)
static DeviceInfoMapPointer parse(const std::string &file_name)
Performs parsing of specified DMAP file.
DeviceDescriptor parseDeviceDesciptor(std::string cddString)
Parse a ChimeraTK device descriptor (CDD) and return the information in the DeviceDescriptor struct.
void loadPluginLibrary(const std::string &soFile)
Load a shared library (.so file) with a Backend at run time.
static BackendFactory & getInstance()
Static function to get an instance of factory.
std::string mapFileName
name of the MAP file storing information about PCIe registers mapping
std::mutex _mutex
A mutex to protect backend creation and returning to keep the maps consistent.
static boost::shared_ptr< DeviceBackend > createInstance(std::string address, std::map< std::string, std::string > parameters)
This structure holds the information of an SDM.
std::map< std::string, boost::weak_ptr< DeviceBackend > > _existingBackends
A map of all created backends.
void setDMapFilePath(std::string dMapFilePath)
This function sets the _DMapFilePath.
std::string dmapFileName
name of the DMAP file
std::string getDMapFilePath()
Returns the _DMapFilePath.
uint32_t dmapFileLineNumber
line number in DMAP file storing listed above information
boost::shared_ptr< DeviceBackend > createBackendInternal(const DeviceInfoMap::DeviceInfo &deviceInfo)
Internal function to return a DeviceBackend.
BackendFactory is a the factory class to create devices.
Sdm parseDeviceString(const std::string &deviceString)
Parse an old-style device string (either path to device node or map file name for dummies)
static boost::shared_ptr< DeviceBackend > createInstance(std::string address, std::map< std::string, std::string > parameters)
bool isSdm(const std::string &theString)
Check wehter the given string seems to be an SDM.
void loadAllPluginsFromDMapFile()
Load all shared libraries specified in the dmap file.
static boost::shared_ptr< DeviceBackend > createInstance(std::string address, std::map< std::string, std::string > parameters)
bool called_registerBackendType
Flag whether the function registerBackendType() was called.
Sdm parseSdm(const std::string &sdmString)
Parse an SDM URI and return the device information in the Sdm struct.
static boost::shared_ptr< DeviceBackend > failedRegistrationThrowerFunction(std::string host, std::string instance, std::list< std::string > parameters, std::string mapFileName, std::string exception_what)
Stores information about one device.
const char * what() const noexcept override
Return the message describing what exactly went wrong.
std::list< std::string > parameters
bool isDeviceDescriptor(std::string theString)
Check whether the given string seems to be a CDD.
static boost::shared_ptr< DeviceBackend > createInstance(std::string address, std::map< std::string, std::string > parameters)
std::map< std::string, boost::function< boost::shared_ptr< DeviceBackend > std::string address, std::map< std::string, std::string > parameters)> > creatorMap
Holds device type and function pointer to the createInstance function of plugin.
static boost::shared_ptr< DeviceBackend > createInstance(std::string address, std::map< std::string, std::string > parameters)
DeviceInfoMap::DeviceInfo aliasLookUp(const std::string &aliasName, const std::string &dmapFilePath)
Search for an alias in a given DMap file and return the DeviceInfo entry.
std::string _dMapFile
The dmap file set at run time.
std::string uri
uri which describes the device (or name of the device file in /dev in backward compatibility mode)
void registerBackendType(const std::string &backendType, boost::shared_ptr< DeviceBackend >(*creatorFunction)(std::string address, std::map< std::string, std::string > parameters), const std::vector< std::string > &sdmParameterNames={}, const std::string &deviceAccessVersion=CHIMERATK_DEVICEACCESS_VERSION)
Register a backend by the name backendType with the given creatorFunction.
static boost::shared_ptr< DeviceBackend > createInstance(std::string address, std::map< std::string, std::string > parameters)
std::string to_string(Boolean &value)
static boost::shared_ptr< DeviceBackend > createInstance(std::string address, std::map< std::string, std::string > parameters)
static boost::shared_ptr< DeviceBackend > createInstance(std::string address, std::map< std::string, std::string > parameters)
Exception thrown when a logic error has occured.
std::map< std::pair< std::string, std::string >, boost::function< boost::shared_ptr< DeviceBackend > std::string host, std::string instance, std::list< std::string > parameters, std::string mapFileName)> > creatorMap_compat
Compatibility creatorMap for old-style backends which just take a plain list of parameters.