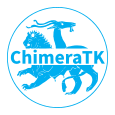 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
5 #include <boost/shared_ptr.hpp>
60 using iterator = std::vector<DeviceInfo>::iterator;
118 friend std::ostream&
operator<<(std::ostream& os,
const TYPE& me);
friend std::ostream & operator<<(std::ostream &os, const DeviceInfo &deviceInfo)
std::vector< DeviceInfo >::const_iterator const_iterator
DeviceInfo()
Default class constructor.
DeviceInfoMap::DeviceInfo _errorDevice1
Detailed information about first device that generate error or warning.
DeviceInfoMap::DeviceInfo _errorDevice2
Detailed information about second device that generate error or warning.
std::vector< DeviceInfo >::iterator iterator
std::vector< std::string > _pluginLibraries
Names of the so files with the plugins.
TYPE _type
Class of detected problem - ERROR or WARNING.
std::string deviceName
logical name of the device
std::string mapFileName
name of the MAP file storing information about PCIe registers mapping
@ WARNING
Non-critical error was detected.
iterator begin()
Return iterator to first device described in DMAP file.
iterator end()
Return iterator to element after last one in DMAP file.
size_t getSize()
Returns number of records in DMAP file.
ErrorElem(TYPE infoType, DMAP_FILE_ERR errorType, const DeviceInfoMap::DeviceInfo &device1, const DeviceInfoMap::DeviceInfo &device2)
Creates obiect that describe one detected error or warning.
std::string dmapFileName
name of the DMAP file
TYPE
Defines available classes of detected problems.
Stores information about errors and warnings.
boost::shared_ptr< DeviceInfoMap > DeviceInfoMapPointer
uint32_t dmapFileLineNumber
line number in DMAP file storing listed above information
Stores detailed information about one error or warning.
DMAP_FILE_ERR
Defines available types of detected problems.
bool check(ErrorList &err, ErrorList::ErrorElem::TYPE level)
Checks logical correctness of DMAP file.
std::string _dmapFileName
name of DMAP file
DeviceInfoMap(std::string fileName)
Constructor.
DMAP_FILE_ERR _errorType
Type of detected problem.
void addPluginLibrary(const std::string &soFile)
Add the name of a library to the list.
std::vector< DeviceInfo > _deviceInfoElements
vector storing parsed contents of DMAP file
Stores information about one device.
friend std::ostream & operator<<(std::ostream &os, const ErrorList &me)
friend std::ostream & operator<<(std::ostream &os, const TYPE &me)
friend std::ostream & operator<<(std::ostream &os, const DeviceInfoMap &deviceInfoMap)
std::list< ErrorElem > _errors
Lists of errors or warnings detected during MAP file correctness checking.
std::string uri
uri which describes the device (or name of the device file in /dev in backward compatibility mode)
std::pair< std::string, std::string > getDeviceFileAndMapFileName() const
Convenience function to extract the device file name and the map file name as one object (a pair).
void insert(const DeviceInfo &elem)
Insert new element read from DMAP file.
void getDeviceInfo(const std::string &deviceName, DeviceInfo &value)
Returns information about specified device.
@ NONUNIQUE_DEVICE_NAME
Names of two devices are the same - treated as critical error.
std::vector< std::string > getPluginLibraries()
You can define shared libraries with Backend plugins in the DMAP file.
@ ERROR
Critical error was detected.