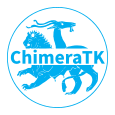 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
15 auto acc = acc_weak.lock();
16 if(!acc->_isActive)
continue;
31 for(
auto& acc_weak : pair.second) {
32 auto acc = acc_weak.lock();
33 if(acc->_isActive)
continue;
37 acc->_isActive =
true;
38 acc->_hasException =
false;
53 for(
auto& acc_weak : pair.second) {
54 auto acc = acc_weak.lock();
55 if(!acc->_isActive)
continue;
56 acc->_isActive =
false;
57 if(acc->_hasException)
continue;
58 acc->_hasException =
true;
71 auto adrPair = std::make_pair(info.bar, info.address);
79 auto adrPair = std::make_pair(info.bar, info.address);
101 [[maybe_unused]] std::string address, std::map<std::string, std::string> parameters) {
102 if(parameters[
"map"].empty()) {
105 return boost::shared_ptr<DeviceBackend>(
new ExceptionDummy(parameters[
"map"]));
146 auto itWriteOrder =
_writeOrderMap.find(std::make_pair(bar, address));
150 auto& orderNumberInMap = itWriteOrder->second;
154 while((current = orderNumberInMap.load()) < generatedOrderNumber) {
155 orderNumberInMap.compare_exchange_weak(current, generatedOrderNumber);
161 itWriteCounter->second++;
167 template<
typename UserType>
168 boost::shared_ptr<NDRegisterAccessor<UserType>> ExceptionDummy::getRegisterAccessor_impl(
170 auto path = registerPathName;
172 auto pathComponents = path.getComponents();
173 bool pushRead =
false;
174 if(pathComponents[pathComponents.size() - 1] ==
"PUSH_READ") {
183 DummyBackendBase, getRegisterAccessor_impl, UserType, path, numberOfWords, wordOffsetInRegister, flags);
187 auto decorator = boost::make_shared<ExceptionDummyPushDecorator<UserType>>(
188 acc, boost::dynamic_pointer_cast<ExceptionDummy>(this->shared_from_this()));
193 decorator->_isActive =
true;
194 decorator->trigger();
199 else if(acc->isReadable()) {
201 auto decorator = boost::make_shared<ExceptionDummyPollDecorator<UserType>>(
202 acc, boost::dynamic_pointer_cast<ExceptionDummy>(this->shared_from_this()));
207 if(pathComponents[pathComponents.size() - 1] !=
"DUMMY_WRITEABLE" &&
208 (pathComponents[0].find(
"DUMMY_INTERRUPT_") != 0)) {
210 auto adrPair = std::make_pair(info.bar, info.address);
217 acc->setExceptionBackend(shared_from_this());
size_t getWriteCount(const RegisterPath &path)
Function to obtain the number of writes of a register since the creation of the backend.
std::atomic< bool > throwExceptionWrite
DummyBackendBase(std::string const &mapFileName)
Base class for DummyBackends, provides common functionality.
void write(uint64_t bar, uint64_t address, int32_t const *data, size_t sizeInBytes) override
Write function to be implemented by backends.
std::atomic< size_t > throwExceptionCounter
void setExceptionImpl() noexcept override
Function to be (optionally) implemented by backends if additional actions are needed when switching t...
std::atomic< bool > throwExceptionOpen
static boost::shared_ptr< DeviceBackend > createInstance(std::string address, std::map< std::string, std::string > parameters)
std::atomic< size_t > _writeOrderCounter
Global counter for order numbers going into _writeOrderMap.
void remove(AccessMode flag)
Remove the given flag from the set.
std::mutex _pushDecoratorsMutex
Mutex to protect data structures for push decorators.
void activateAsyncRead() noexcept override
Activate asyncronous read for all transfer elements where AccessMode::wait_for_new_data is set.
std::map< std::pair< uint64_t, uint64_t >, std::atomic< size_t > > _writeCounterMap
Map used allow determining number of writes of a specific register by tests. Map key is pair of bar a...
void open() override
Open the device.
void setAltSeparator(const std::string &altSeparator)
set alternative separator.
Exception thrown when a runtime error has occured.
bool has(AccessMode flag) const
Check if a certain flag is in the set.
std::map< RegisterPath, std::list< boost::weak_ptr< ExceptionDummyPushDecoratorBase > > > _pushDecorators
Map of active ExceptionDummyPushDecorator. Protected by _pushDecoratorMutex.
#define CALL_BASE_FUNCTION_TEMPLATE(BaseClass, functionName, templateArgument,...)
Execute the virtual function template call to the base implementation of the function.
ExceptionDummy(std::string const &mapFileName)
void activateAsyncRead() noexcept override
Activate asyncronous read for all transfer elements where AccessMode::wait_for_new_data is set.
@ wait_for_new_data
Make any read blocking until new data has arrived since the last read.
void read(uint64_t bar, uint64_t address, int32_t *data, size_t sizeInBytes) override
Read function to be implemented by backends.
void setException(const std::string &message) noexcept final
Set the backend into an exception state.
#define OVERRIDE_VIRTUAL_FUNCTION_TEMPLATE(BaseClass, functionName)
Save the old vtable to be accessible by CALL_BASE_FUNCTION_TEMPLATE and overwrite it with the new imp...
std::map< std::pair< uint64_t, uint64_t >, std::atomic< size_t > > _writeOrderMap
Map used allow determining order of writes by tests. Map key is pair of bar and address.
void read(uint64_t bar, uint64_t address, int32_t *data, size_t sizeInBytes) override
Read function to be implemented by backends.
size_t getWriteOrder(const RegisterPath &path)
Function to obtain the write order number of a register.
void open() override
Open the device.
bool _activateNewPushAccessors
Flag is toggled by activateAsyncRead (true), setException (false) and close (false).
NumericAddressedRegisterInfo getRegisterInfo(const RegisterPath ®isterPathName)
getRegisterInfo returns a NumericAddressedRegisterInfo object for the given register.
Class to store a register path name.
void triggerPush(RegisterPath path, VersionNumber v={})
Function to trigger sending values for push-type variables.
Class for generating and holding version numbers without exposing a numeric representation.
bool asyncReadActivated()
Function to test whether async read transfers are activated.
The dummy device opens a mapping file instead of a device, and implements all registers defined in th...
void setExceptionImpl() noexcept override
Turn off the internal variable which remembers that async is active.
void write(uint64_t bar, uint64_t address, int32_t const *data, size_t sizeInBytes) override
Write function to be implemented by backends.
Set of AccessMode flags with additional functionality for an easier handling.
void closeImpl() override
This closes the device, clears all internal registers, read-only settings and callback functions.
std::atomic< bool > throwExceptionRead
std::map< RegisterPath, VersionNumber > _pushVersions
Map of version numbers to use in push decorators. Protected by _pushDecoratorMutex.
void closeImpl() override
This closes the device, clears all internal registers, read-only settings and callback functions.
Exception thrown when a logic error has occured.