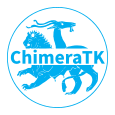 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
5 #include "DeviceAccessVersion.h"
9 #include <boost/function.hpp>
18 #define TEST_DMAP_FILE_PATH "./dummies.dmap" // FIXME remove
19 #define ENV_VAR_DMAP_FILE "DMAP_PATH_ENV" // FIXME remove
56 boost::shared_ptr<DeviceBackend> (*creatorFunction)(
57 std::string address, std::map<std::string, std::string> parameters),
58 const std::vector<std::string>& sdmParameterNames = {},
59 const std::string& deviceAccessVersion = CHIMERATK_DEVICEACCESS_VERSION);
65 [[deprecated]]
void registerBackendType(
const std::string& interface,
const std::string& protocol,
66 boost::shared_ptr<DeviceBackend> (*creatorFunction)(
67 std::string host, std::string instance, std::list<std::string> parameters, std::string mapFileName),
68 const std::string& version);
74 boost::shared_ptr<DeviceBackend>
createBackend(
const std::string& aliasOrUri);
89 boost::function<boost::shared_ptr<DeviceBackend>(
90 std::string address, std::map<std::string, std::string> parameters)>>
95 std::map<std::pair<std::string, std::string>,
96 boost::function<boost::shared_ptr<DeviceBackend>(
97 std::string host, std::string instance, std::list<std::string> parameters, std::string mapFileName)>>
101 std::string
aliasLookUp(std::string aliasName, std::string dmapFilePath);
119 std::list<std::string> parameters, std::string mapFileName, std::string exception_what);
boost::shared_ptr< DeviceBackend > createBackend(const std::string &aliasOrUri)
Create a new backend and return the instance as a shared pointer.
void loadPluginLibrary(const std::string &soFile)
Load a shared library (.so file) with a Backend at run time.
static BackendFactory & getInstance()
Static function to get an instance of factory.
std::mutex _mutex
A mutex to protect backend creation and returning to keep the maps consistent.
std::map< std::string, boost::weak_ptr< DeviceBackend > > _existingBackends
A map of all created backends.
void setDMapFilePath(std::string dMapFilePath)
This function sets the _DMapFilePath.
std::string getDMapFilePath()
Returns the _DMapFilePath.
boost::shared_ptr< DeviceBackend > createBackendInternal(const DeviceInfoMap::DeviceInfo &deviceInfo)
Internal function to return a DeviceBackend.
BackendFactory is a the factory class to create devices.
void loadAllPluginsFromDMapFile()
Load all shared libraries specified in the dmap file.
bool called_registerBackendType
Flag whether the function registerBackendType() was called.
static boost::shared_ptr< DeviceBackend > failedRegistrationThrowerFunction(std::string host, std::string instance, std::list< std::string > parameters, std::string mapFileName, std::string exception_what)
Stores information about one device.
std::map< std::string, boost::function< boost::shared_ptr< DeviceBackend > std::string address, std::map< std::string, std::string > parameters)> > creatorMap
Holds device type and function pointer to the createInstance function of plugin.
std::string aliasLookUp(std::string aliasName, std::string dmapFilePath)
Look for the alias and if found return a uri.
std::string _dMapFile
The dmap file set at run time.
void registerBackendType(const std::string &backendType, boost::shared_ptr< DeviceBackend >(*creatorFunction)(std::string address, std::map< std::string, std::string > parameters), const std::vector< std::string > &sdmParameterNames={}, const std::string &deviceAccessVersion=CHIMERATK_DEVICEACCESS_VERSION)
Register a backend by the name backendType with the given creatorFunction.
std::map< std::pair< std::string, std::string >, boost::function< boost::shared_ptr< DeviceBackend > std::string host, std::string instance, std::list< std::string > parameters, std::string mapFileName)> > creatorMap_compat
Compatibility creatorMap for old-style backends which just take a plain list of parameters.