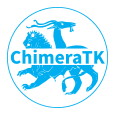 |
ChimeraTK-ApplicationCore
04.01.00
|
Go to the documentation of this file.
18 #include <ChimeraTK/ApplicationCore/ApplicationCore.h>
19 #include <ChimeraTK/ApplicationCore/ConfigReader.h>
20 #include <ChimeraTK/ApplicationCore/PeriodicTrigger.h>
21 #include <ChimeraTK/ApplicationCore/ScriptedInitialisationHandler.h>
22 #include <ChimeraTK/ApplicationCore/VersionInfoProvider.h>
70 Controller controller{
this,
"Controller",
"The temperature controller"};
75 AverageCurrent averageCurrent{
this,
"AverageCurrent",
"Provide averaged heater current"};
78 ControlUnit controlUnit{
this,
"ControlUnit",
"Unit for controlling the oven temperature"};
83 SetpointRamp(
this,
"SetpointRamp",
"Slow ramping of temperator setpoint") :
This module can be added to applications to provide version information from the CMakeLists....
const T & get(const std::string &variableName) const
Get value for given configuration variable.
[Snippet: Class Definition]
~ExampleApp() override
[Snippet: Destructor]
Initialisation handler which calls an external application (usually a script), captures its output (b...
[Snippet: Class Definition Start]
ModuleGroup()=default
Default constructor to allow late initialisation of module groups.
ConfigReader & getConfigReader()
[Snippet: Class Definition]
Helper class to set the DMAP file path.
InvalidityTracer application module.
const std::string & getName() const
Get the name of the module instance.
Simple periodic trigger that fires a variable once per second.