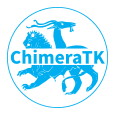 |
ChimeraTK-ApplicationCore
04.01.00
|
Go to the documentation of this file.
10 #include <ChimeraTK/Utilities.h>
20 const std::unordered_set<std::string>& tags)
23 throw ChimeraTK::logic_error(
"ApplicationModule owner cannot be nullptr");
36 assert(!_moduleThread.joinable());
46 model.informMove(*
this);
67 while(!
_moduleThread.try_join_for(boost::chrono::milliseconds(10))) {
70 auto el{var.getAppAccessorNoType().getHighLevelImplElement()};
111 assert(!variable.getAppAccessorNoType().getHighLevelImplElement()->getAccessModeFlags().has(
112 AccessMode::wait_for_new_data));
115 variable.getAppAccessorNoType().read();
134 variable.getAppAccessorNoType().read();
169 if(std::count(startList.begin(), startList.end(),
this)) {
183 startList.push_back(
this);
184 std::list<EntityOwner*> returnList{
193 auto proxy = accessor.getModel().visit(Model::returnApplicationModule, Model::keepApplicationModules,
195 if(!proxy.isValid()) {
198 auto& feedingModule = proxy.getApplicationModule();
200 auto thisInputsRecursiveModuleList = feedingModule.getInputModulesRecursively(startList);
202 assert(startList.size() <= thisInputsRecursiveModuleList.size());
203 auto copyStartIter = thisInputsRecursiveModuleList.begin();
204 std::advance(copyStartIter, startList.size());
206 returnList.insert(returnList.end(), copyStartIter, thisInputsRecursiveModuleList.end());
221 throw ChimeraTK::logic_error(
222 "Error: setCircularNetworkHash() called with different values for EntityOwner \"" +
_name +
"\" ");
225 throw ChimeraTK::logic_error(
"Error: setCircularNetworkHash() called after initialisation.");
234 return DataValidity::ok;
241 return DataValidity::ok;
245 return DataValidity::faulty;
std::list< VariableNetworkNode > getAccessorListRecursive() const
Obtain the list of accessors/variables associated with this instance and any submodules.
void remove(VariableGroup &module)
static void registerThread(const std::string &name)
Register the thread in the application system and give it a name.
std::atomic< size_t > _dataFaultCounter
Number of inputs which report DataValidity::faulty.
VersionNumber _currentVersionNumber
Version number of last push-type read operation - will be passed on to any write operations.
void setCurrentVersionNumber(VersionNumber versionNumber) override
Set the current version number.
detail::CircularDependencyDetector _circularDependencyDetector
static Application & getInstance()
Obtain instance of the application.
void terminate() override
Terminate the module.
detail::CircularDependencyDetectionRecursionStopper _recursionStopper
Helper needed to stop the recursion when detecting circular dependency networks.
void setCircularNetworkHash(size_t circularNetworkHash)
Set the ID of the circular dependency network.
ModuleGroupProxy add(ModuleGroup &module)
ApplicationModule & operator=(ApplicationModule &&other) noexcept
Move assignment.
virtual void mainLoop()=0
To be implemented by the user: function called in a separate thread executing the main loop of the mo...
VariableGroup & operator=(VariableGroup &&other) noexcept
Move assignment.
void decrementDataFaultCounter() override
Decrement the fault counter and set the data validity flag to ok if the counter has reached 0.
void unregisterModule(Module *module) override
Unregister another module as a sub-module.
ChimeraTK::Model::ModuleGroupProxy getModel()
Return the application model proxy representing this module.
void unregisterModule(Module *module) override
Unregister another module as a sub-module.
bool isValid() const
Check if the model is valid.
ChimeraTK::Model::ApplicationModuleProxy _model
ChimeraTK::Model::VariableGroupProxy _model
void run() override
Execute the module.
boost::thread _moduleThread
The thread executing mainLoop()
@ initialisation
Initialisation phase including ApplicationModule::prepare().
size_t _circularNetworkHash
Unique ID for the circular dependency network.
~ApplicationModule() override
Destructor.
DataValidity getDataValidity() const override
Return the data validity flag.
std::string _name
The name of this instance.
void incrementDataFaultCounter() override
Set the data validity flag to fault and increment the fault counter.
ApplicationModule()=default
Default constructor: Allows late initialisation of modules (e.g.
InvalidityTracer application module.
std::string className()
Name of the module class, used for logging and debugging purposes.
detail::TestableMode & getTestableMode()
Get the TestableMode control object of this application.
std::atomic< bool > _testableModeReached
Flag used by the testable mode to identify whether a thread within the EntityOwner has reached the po...
Base class for ApplicationModule and DeviceModule, to have a common interface for these module types.
std::list< EntityOwner * > getInputModulesRecursively(std::list< EntityOwner * > startList) override
Use pointer to the module as unique identifier.
size_t getCircularNetworkHash() const override
Get the ID of the circular dependency network (0 if none).
void mainLoopWrapper()
Wrapper around mainLoop(), to execute additional tasks in the thread before entering the main loop.