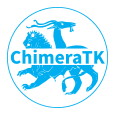 |
ChimeraTK-ApplicationCore
04.01.00
|
Go to the documentation of this file.
11 #include <unordered_map>
12 #include <unordered_set>
14 #include <ChimeraTK/NDRegisterAccessorAbstractor.h>
16 #include <boost/shared_ptr.hpp>
27 struct VariableNetworkNode_data;
48 const std::string& description,
const std::type_info* valueType,
49 const std::unordered_set<std::string>& tags = {});
52 VariableNetworkNode(
const std::string& name,
const std::string& devAlias,
const std::string& regName,
54 size_t nElements = 0);
58 const std::type_info& valTyp =
typeid(
AnyType),
size_t nElements = 0);
79 void setMetaData(
const std::string& name,
const std::string& unit,
const std::string& description)
const;
80 void setMetaData(
const std::string& name,
const std::string& unit,
const std::string& description,
81 const std::unordered_set<std::string>& tags)
const;
103 void dump(std::ostream& stream = std::cout)
const;
109 void addTag(
const std::string& tag)
const;
128 [[nodiscard]]
const std::type_info&
getValueType()
const;
129 [[nodiscard]] std::string
getName()
const;
131 [[nodiscard]]
const std::string&
getUnit()
const;
137 [[nodiscard]]
const std::unordered_set<std::string>&
getTags()
const;
148 template<
typename UserType>
149 ChimeraTK::NDRegisterAccessorAbstractor<UserType>&
getAppAccessor()
const;
151 template<
typename UserType>
169 template<
typename UserType>
172 template<
typename UserType>
177 boost::shared_ptr<VariableNetworkNode_data>
pdata;
209 std::string
unit{ChimeraTK::TransferElement::unitNotSet};
215 ChimeraTK::TransferElementAbstractor*
appNode{
nullptr};
240 std::unordered_set<std::string>
tags;
263 template<
typename UserType>
267 auto accessor =
static_cast<ChimeraTK::NDRegisterAccessorAbstractor<UserType>*
>(
pdata->appNode);
268 assert(accessor !=
nullptr);
274 template<
typename UserType>
279 decorated->disableDataValidityPropagation();
282 getAppAccessor<UserType>().replace(decorated);
283 auto flagProvider = boost::dynamic_pointer_cast<MetaDataPropagationFlagProvider>(decorated);
284 assert(flagProvider);
289 template<
typename UserType>
292 boost::fusion::at_key<UserType>(
pdata->constantValue) = value;
297 template<
typename UserType>
300 return boost::fusion::at_key<UserType>(
pdata->constantValue);
Class describing a node of a variable network.
Struct to define the direction of variables.
std::string name
Accessor name if type == Application.
boost::shared_ptr< VariableNetworkNode_data > pdata
EntityOwner * getOwningModule() const
void setConstantValue(UserType value)
NodeType getType() const
Getter for the properties.
std::string getQualifiedName() const
std::unordered_set< std::string > tags
Set of tags if type == Application.
Base class for owners of other EntityOwners (e.g.
std::string publicName
Public name if type == ControlSystem.
std::string unit
Engineering unit.
const std::string & getUnit() const
const std::type_info & getValueType() const
void setAppAccessorImplementation(boost::shared_ptr< ChimeraTK::NDRegisterAccessor< UserType >> impl) const
void accept(Visitor< VariableNetworkNode > &visitor) const
ChimeraTK::NDRegisterAccessorAbstractor< UserType > & getAppAccessor() const
void clearOwner()
Clear the owner network of this node.
size_t nElements
Number of elements in the variable.
UpdateMode
Enum to define the update mode of variables.
const std::string & getDeviceAlias() const
const std::string & getDescription() const
Pseudo type to identify nodes which can have arbitrary types.
UserType getConstantValue() const
UpdateMode mode
Update mode: poll or push.
Model::ProcessVariableProxy getModel() const
bool hasImplementation() const
Function checking if the node requires a fixed implementation.
std::string qualifiedName
void setDirection(VariableDirection newDirection) const
Set the direction for this node.
size_t getCircularNetworkHash() const
Get the unique ID of the circular network.
VariableNetworkNode()
Default constructor for an invalid node.
ChimeraTK::TransferElementAbstractor * appNode
Pointer to implementation if type == Application.
const std::string & getRegisterName() const
const std::unordered_set< std::string > & getTags() const
const std::type_info * valueType
Value type of this node.
EntityOwner * owningModule
Pointer to the module owning this node.
VariableNetworkNode nodeToTrigger
Pointer to network which should be triggered by this node.
std::string deviceAlias
Device information if type == Device.
size_t circularNetworkHash
Hash which idientifies a circular network.
std::map< VariableNetworkNode, VariableNetworkNode > nodeWithTrigger
Map to store triggered versions of this node.
std::string description
Description.
const void * getUniqueId() const
Return the unique ID of this node (will change every time the application is started).
bool operator<(const VariableNetworkNode &other) const
const std::string & getPublicName() const
VariableDirection direction
Node direction: feeding or consuming.
constexpr auto explicitDataValidityTag
Special tag to designate that a not should not automatically take over DataValidity of its owning mod...
bool operator!=(const VariableNetworkNode &other) const
void addTag(const std::string &tag) const
Add a tag.
void setAppAccessorPointer(ChimeraTK::TransferElementAbstractor *accessor) const
Change pointer to the accessor.
UpdateMode getMode() const
size_t getNumberOfElements() const
void setAppAccessorConstImplementation(const VariableNetworkNode &feeder) const
NodeType
Enum to define types of VariableNetworkNode.
VariableNetworkNode getNodeToTrigger() const
void setModel(const Model::ProcessVariableProxy &model) const
NodeType type
Type of the node (Application, Device, ControlSystem, Trigger)
bool isCircularInput() const
Returns true if a circular dependency has been detected and the node is a consumer.
Proxy class which does not keep the ownership of the model.
VariableNetworkNode externalTrigger
Pointer to the network providing the external trigger.
Model::NonOwningProxy< Model::ProcessVariableProxy > model
Model representation of this variable.
VariableNetworkNode & operator=(const VariableNetworkNode &rightHandSide)
Copy by assignment operator: Just copy the pointer to the data storage object.
std::string getName() const
bool operator==(const VariableNetworkNode &other) const
Compare two nodes.
void setOwningModule(EntityOwner *newOwner) const
VariableDirection getDirection() const
userTypeMap constantValue
Value in case of a constant.
void setNumberOfElements(size_t nElements) const
void setValueType(const std::type_info &newType) const
Set the value type for this node.
We use a pimpl pattern so copied instances of VariableNetworkNode refer to the same instance of the d...
InvalidityTracer application module.
VariableNetworkNode_data()=default
ChimeraTK::TransferElementAbstractor & getAppAccessorNoType() const
void setPublicName(const std::string &name) const
void dump(std::ostream &stream=std::cout) const
Print node information to specified stream.
void setMetaData(const std::string &name, const std::string &unit, const std::string &description) const
Change meta data (name, unit, description and optionally tags).
std::list< EntityOwner * > scanForCircularDepencency() const
Scan the networks and set the isCircularInput() flags if circular dependencies are detected.