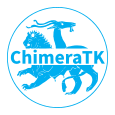 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
58 uint64_t address_ = 0, uint32_t nBytes_ = 0, uint64_t bar_ = 0, uint32_t width_ = 32,
66 uint32_t elementPitchBits_, std::vector<ChannelInfo> channelInfo_,
Access dataAccess_,
67 std::vector<size_t> interruptId_);
124 [[nodiscard]] std::unique_ptr<BackendRegisterInfoBase>
clone()
const override {
131 void computeDataDescriptor();
145 [[nodiscard]] std::unique_ptr<BackendRegisterCatalogueBase>
clone()
const override;
bool operator!=(const ChannelInfo &rhs) const
std::vector< size_t > interruptId
uint64_t address
Lower part of the address relative to BAR, in bytes.
void addRegister(const NumericAddressedRegisterInfo ®isterInfo)
@ raw
Raw access: disable any possible conversion from the original hardware data type into the given UserT...
int32_t nFractionalBits
Number of fractional bits.
uint32_t width
Number of significant bits in the register.
unsigned int getNumberOfElements() const override
Return number of elements per channel.
NumericAddressedRegisterInfo getBackendRegister(const RegisterPath ®isterPathName) const override
Note: Override this function if backend has "hidden" registers which are not added to the map and hen...
uint32_t nElements
Number of elements in register.
bool operator==(const ChimeraTK::NumericAddressedRegisterInfo &rhs) const
void add(AccessMode flag)
Add the given flag to the set.
Interface for backends to the register catalogue.
Class describing the actual payload data format of a register in an abstract manner.
Type dataType
Data type (fixpoint, floating point)
bool signedFlag
Signed/Unsigned flag.
const DataDescriptor & getDataDescriptor() const override
Return desciption of the actual payload data for this register.
const std::set< std::vector< size_t > > & getListOfInterrupts() const
@ wait_for_new_data
Make any read blocking until new data has arrived since the last read.
uint32_t bitOffset
Offset in bits w.r.t.
RegisterPath getRegisterName() const override
Return full path name of the register (including modules)
uint64_t bar
Upper part of the address (name originally from PCIe, meaning now generalised)
std::unique_ptr< BackendRegisterCatalogueBase > clone() const override
Create deep copy of the catalogue.
DataDescriptor dataDescriptor
A class to describe which of the supported data types is used.
NumericAddressedRegisterInfo & operator=(const NumericAddressedRegisterInfo &other)=default
std::vector< ChannelInfo > channels
Define per-channel information (bit interpretation etc.), 1D/scalars have exactly one entry.
unsigned int getNumberOfChannels() const override
Return number of channels in register.
bool isWriteable() const override
Return whether the register is writeable.
Type
Enum descibing the data interpretation:
uint32_t elementPitchBits
Distance in bits (!) between two elements (of the same channel)
bool operator==(const ChannelInfo &rhs) const
Access
Enum describing the access mode of the register:
std::vector< size_t > getQualifiedAsyncId() const override
Return the fully qualified async::SubDomain ID.
Class to store a register path name.
NumericAddressedRegisterInfo(RegisterPath const &pathName_={}, uint32_t nElements_=0, uint64_t address_=0, uint32_t nBytes_=0, uint64_t bar_=0, uint32_t width_=32, int32_t nFractionalBits_=0, bool signedFlag_=true, Access dataAccess_=Access::READ_WRITE, Type dataType_=Type::FIXED_POINT, std::vector< size_t > interruptId_={})
Constructor to set all data members for scalar/1D registers.
Access registerAccess
Data access direction: Read, write, read and write or interrupt.
Set of AccessMode flags with additional functionality for an easier handling.
bool hasRegister(const RegisterPath ®isterPathName) const override
Check if register with the given path name exists.
bool isReadable() const override
Return whether the register is readable.
bool operator!=(const ChimeraTK::NumericAddressedRegisterInfo &rhs) const
AccessModeFlags getSupportedAccessModes() const override
Return all supported AccessModes for this register.
DeviceBackend-independent register description.
std::map< RegisterPath, std::vector< size_t > > _canonicalInterrupts
A canonical interrupt path consists of an exclamation mark, followed by a numeric interrupt and a col...
std::set< std::vector< size_t > > _listOfInterrupts
set of interrupt IDs.
std::unique_ptr< BackendRegisterInfoBase > clone() const override
Create copy of the object.
DataType getRawType() const
Return raw type matching the given width.