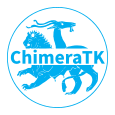 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
61 [[nodiscard]]
size_t nDigits()
const;
146 size_t _nFractionalDigits;
bool operator!=(const DataDescriptor &other) const
void setRawDataType(const DataType &d)
Set the raw data type.
DataType transportLayerDataType() const
Get the data type on the transport layer.
DataType minimumDataType() const
Get the minimum data type required to represent the described data type in the host CPU.
Class describing the actual payload data format of a register in an abstract manner.
FundamentalType fundamentalType() const
Get the fundamental data type.
size_t nFractionalDigits() const
Approximate maximum number of digits after decimal dot (of base 10) needed to represent the value (ex...
bool isSigned() const
Return whether the data is signed or not.
bool operator==(const DataDescriptor &other) const
@ none
The data type/concept does not exist, e.g. there is no raw transfer (do not confuse with Void)
A class to describe which of the supported data types is used.
DataType rawDataType() const
Get the raw data type.
FundamentalType
Enum for the fundamental data types.
std::ostream & operator<<(std::ostream &stream, const DataDescriptor::FundamentalType &fundamentalType)
bool isIntegral() const
Return whether the data is integral or not (e.g.
size_t nDigits() const
Return the approximate maximum number of digits (of base 10) needed to represent the value (including...
DataDescriptor()
Default constructor sets fundamental type to "undefined".