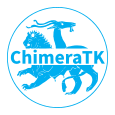 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
12 template<
typename UserType>
18 template<
typename UserType>
31 std::string address, std::map<std::string, std::string> parameters);
37 void read(uint64_t bar, uint64_t address, int32_t* data,
size_t sizeInBytes)
override;
39 void write(uint64_t bar, uint64_t address, int32_t
const* data,
size_t sizeInBytes)
override;
66 std::map<RegisterPath, std::list<boost::weak_ptr<ExceptionDummyPushDecoratorBase>>>
_pushDecorators;
103 template<
typename UserType>
104 boost::shared_ptr<NDRegisterAccessor<UserType>> getRegisterAccessor_impl(
119 template<
typename UserType>
120 struct ExceptionDummyPushDecorator : NDRegisterAccessorDecorator<UserType>, ExceptionDummyPushDecoratorBase {
122 boost::shared_ptr<ExceptionDummy> backend)
124 assert(_target->isReadable());
126 this->_accessModeFlags = _target->getAccessModeFlags();
132 _path = _target->getName();
134 _path /=
"PUSH_READ";
138 std::unique_lock<std::mutex> lk(
_backend->_pushDecoratorsMutex);
141 for(
auto it = list.begin(); it != list.end(); ++it) {
142 if(it->lock().get() ==
nullptr) {
148 catch(std::exception& e) {
153 std::cout <<
"~ExceptionDummyPushDecorator(): Unexpected exception: " << e.what() << std::endl;
155 std::cout <<
"~ExceptionDummyPushDecorator(): Could not unlist instance!" << std::endl;
171 std::vector<std::vector<UserType>>
_data;
181 _hasException =
false;
184 b.
_data = _target->accessChannels();
192 if(!_hasException)
_myReadQueue.push_overwrite_exception(std::current_exception());
193 _hasException =
true;
206 if(updateDataBuffer) {
232 template<
typename UserType>
235 boost::shared_ptr<ExceptionDummy> backend)
237 assert(_target->isReadable());
239 _path = _target->getName();
size_t getWriteCount(const RegisterPath &path)
Function to obtain the number of writes of a register since the creation of the backend.
std::map< RegisterPath, DataValidity > _registerValidities
This map is used for setting individual (poll-type) variables to DataValidity=faulty.
boost::shared_ptr< ExceptionDummy > _backend
ExceptionDummyPushDecorator(const boost::shared_ptr< ChimeraTK::NDRegisterAccessor< UserType >> &target, boost::shared_ptr< ExceptionDummy > backend)
Base class for DummyBackends, provides common functionality.
@ faulty
The data is considered valid.
void setExceptionImpl() noexcept override
Function to be (optionally) implemented by backends if additional actions are needed when switching t...
VersionNumber _versionNumber
The version number of the last successful transfer.
static boost::shared_ptr< DeviceBackend > createInstance(std::string address, std::map< std::string, std::string > parameters)
ExceptionDummyPollDecorator(const boost::shared_ptr< ChimeraTK::NDRegisterAccessor< UserType >> &target, boost::shared_ptr< ExceptionDummy > backend)
std::atomic< size_t > _writeOrderCounter
Global counter for order numbers going into _writeOrderMap.
DataValidity _dataValidity
The validity of the data in the application buffer.
std::mutex _pushDecoratorsMutex
Mutex to protect data structures for push decorators.
void activateAsyncRead() noexcept override
Activate asyncronous read for all transfer elements where AccessMode::wait_for_new_data is set.
std::map< std::pair< uint64_t, uint64_t >, std::atomic< size_t > > _writeCounterMap
Map used allow determining number of writes of a specific register by tests. Map key is pair of bar a...
void setAltSeparator(const std::string &altSeparator)
set alternative separator.
void setExceptionBackend(boost::shared_ptr< DeviceBackend > exceptionBackend) override
~ExceptionDummyPushDecorator() override
Exception thrown when a runtime error has occured.
DataValidity getValidity(RegisterPath path)
Query map for overwritten data validities.
std::map< RegisterPath, std::list< boost::weak_ptr< ExceptionDummyPushDecoratorBase > > > _pushDecorators
Map of active ExceptionDummyPushDecorator. Protected by _pushDecoratorMutex.
void doPreRead(TransferType) override
cppext::future_queue< Buffer > _myReadQueue
ExceptionDummy(std::string const &mapFileName)
void interrupt() override
DataValidity
The current state of the data.
DataValidity _dataValidity
The validity of the data in the application buffer.
void doPostRead(TransferType, bool updateDataBuffer) override
@ wait_for_new_data
Make any read blocking until new data has arrived since the last read.
virtual void setExceptionBackend(boost::shared_ptr< DeviceBackend > exceptionBackend)
Set the backend to which the exception has to be reported.
void read(uint64_t bar, uint64_t address, int32_t *data, size_t sizeInBytes) override
Read function to be implemented by backends.
DataValidity _dataValidity
The validity of the data in the application buffer.
std::map< std::pair< uint64_t, uint64_t >, std::atomic< size_t > > _writeOrderMap
Map used allow determining order of writes by tests. Map key is pair of bar and address.
A decorator that returns invalid data for polled variables.
TransferType
Used to indicate the applicable operation on a Transferelement.
size_t getWriteOrder(const RegisterPath &path)
Function to obtain the write order number of a register.
void open() override
Open the device.
bool _activateNewPushAccessors
Flag is toggled by activateAsyncRead (true), setException (false) and close (false).
Base class for decorators of the NDRegisterAccessor.
void setValidity(RegisterPath path, DataValidity val)
Use decorator to overwrite returned data validity of individual (poll-type) variables.
DEFINE_VIRTUAL_FUNCTION_OVERRIDE_VTABLE(DummyBackendBase, getRegisterAccessor_impl, boost::shared_ptr< NDRegisterAccessor< T >>(const RegisterPath &, size_t, size_t, AccessModeFlags))
std::vector< std::vector< UserType > > _data
Class to store a register path name.
cppext::future_queue< void > _readQueue
The queue for asynchronous read transfers.
boost::shared_ptr< ExceptionDummy > _backend
void triggerPush(RegisterPath path, VersionNumber v={})
Function to trigger sending values for push-type variables.
std::mutex _registerValiditiesMutex
mutex to protect map _registerValidities
Class for generating and holding version numbers without exposing a numeric representation.
virtual ~ExceptionDummyPollDecoratorBase()=default
cppext::future_queue< void > _readQueue
The queue for asynchronous read transfers.
bool asyncReadActivated()
Function to test whether async read transfers are activated.
The dummy device opens a mapping file instead of a device, and implements all registers defined in th...
void write(uint64_t bar, uint64_t address, int32_t const *data, size_t sizeInBytes) override
Write function to be implemented by backends.
Set of AccessMode flags with additional functionality for an easier handling.
virtual ~ExceptionDummyPushDecoratorBase()=default
void closeImpl() override
This closes the device, clears all internal registers, read-only settings and callback functions.
std::map< RegisterPath, VersionNumber > _pushVersions
Map of version numbers to use in push decorators. Protected by _pushDecoratorMutex.
void doPostRead(TransferType type, bool updateDataBuffer) override
Exception thrown when a logic error has occured.
VersionNumber _versionNumber
The version number of the last successful transfer.