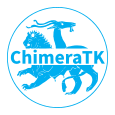 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
15 #define TRY_REGISTER_ACCESS(COMMAND) \
19 catch(std::out_of_range & outOfRangeException) { \
20 std::stringstream errorMessage; \
21 errorMessage << "Invalid address offset " << address << " in bar " << bar << "." \
22 << "Caught out_of_range exception: " << outOfRangeException.what(); \
23 std::cout << errorMessage.str() << std::endl; \
24 throw ChimeraTK::logic_error(errorMessage.str()); \
55 void read([[maybe_unused]] uint8_t bar, [[maybe_unused]] uint32_t address, [[maybe_unused]] int32_t* data,
56 [[maybe_unused]]
size_t sizeInBytes)
final {
63 void write([[maybe_unused]] uint8_t bar, [[maybe_unused]] uint32_t address, [[maybe_unused]] int32_t
const* data,
64 [[maybe_unused]]
size_t sizeInBytes)
final {
82 template<
typename UserType>
101 template<
typename T,
typename... Args>
103 [[maybe_unused]]
const std::string& instanceId, Args&&... arguments) {
104 return boost::make_shared<T>(std::forward<Args>(arguments)...);
void read([[maybe_unused]] uint8_t bar, [[maybe_unused]] uint32_t address, [[maybe_unused]] int32_t *data, [[maybe_unused]] size_t sizeInBytes) final
You cannot override the read version with 32 bit address any more.
std::atomic< bool > throwExceptionWrite
DEFINE_VIRTUAL_FUNCTION_OVERRIDE_VTABLE(NumericAddressedBackend, getRegisterAccessor_impl, boost::shared_ptr< NDRegisterAccessor< T >>(const RegisterPath &, size_t, size_t, AccessModeFlags))
~DummyBackendBase() override=default
DummyBackendBase(std::string const &mapFileName)
static boost::shared_ptr< DeviceBackend > returnInstance([[maybe_unused]] const std::string &instanceId, Args &&... arguments)
Backward compatibility: Leftover from the time when the dummy managed it's own map to return the same...
bool barIndexValid([[maybe_unused]] uint64_t bar) override
All bars are valid in dummies.
std::map< uint64_t, size_t > getBarSizesInBytesFromRegisterMapping() const
Determines the size of each bar because the DummyBackends allocate memory per bar.
Base class for DummyBackends, provides common functionality.
std::atomic< size_t > throwExceptionCounter
std::atomic< bool > throwExceptionOpen
virtual VersionNumber triggerInterrupt(uint32_t interruptNumber)=0
Simulate the arrival of an interrupt.
boost::shared_ptr< NDRegisterAccessor< UserType > > getRegisterAccessor_impl(const RegisterPath ®isterPathName, size_t numberOfWords, size_t wordOffsetInRegister, AccessModeFlags flags)
Specific override which allows to create "DUMMY_INTEERRUPT_X" accessors.
static void checkSizeIsMultipleOfWordSize(size_t sizeInBytes)
void write([[maybe_unused]] uint8_t bar, [[maybe_unused]] uint32_t address, [[maybe_unused]] int32_t const *data, [[maybe_unused]] size_t sizeInBytes) final
You cannot override the write version with 32 bit address any more.
Base class for address-based device backends (e.g.
Class to store a register path name.
Class for generating and holding version numbers without exposing a numeric representation.
size_t minimumTransferAlignment([[maybe_unused]] uint64_t bar) const override
Determines the supported minimum alignment for any read/write requests.
Set of AccessMode flags with additional functionality for an easier handling.
std::atomic< bool > throwExceptionRead