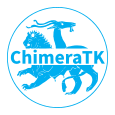 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
24 std::map<uint64_t, size_t> barSizesInBytes;
26 if(info.elementPitchBits % 8 != 0) {
29 barSizesInBytes[info.bar] = std::max(
30 barSizesInBytes[info.bar],
static_cast<size_t>(info.address + info.nElements * info.elementPitchBits / 8));
32 return barSizesInBytes;
36 if(sizeInBytes %
sizeof(int32_t)) {
41 template<
typename UserType>
46 if(registerPathName.
startsWith(
"DUMMY_INTERRUPT_")) {
51 assert(dummyCatalogue);
52 std::tie(interruptFound, interrupt) = dummyCatalogue->extractControllerInterrupt(registerPathName);
60 shared_from_this(), [
this, interrupt]() {
return triggerInterrupt(interrupt); }, registerPathName,
61 numberOfWords, wordOffsetInRegister, flags);
63 return boost::shared_ptr<NDRegisterAccessor<UserType>>(d);
68 numberOfWords, wordOffsetInRegister, flags);
DummyBackendBase(std::string const &mapFileName)
bool barIndexValid([[maybe_unused]] uint64_t bar) override
All bars are valid in dummies.
std::map< uint64_t, size_t > getBarSizesInBytesFromRegisterMapping() const
Determines the size of each bar because the DummyBackends allocate memory per bar.
virtual VersionNumber triggerInterrupt(uint32_t interruptNumber)=0
Simulate the arrival of an interrupt.
boost::shared_ptr< NDRegisterAccessor< UserType > > getRegisterAccessor_impl(const RegisterPath ®isterPathName, size_t numberOfWords, size_t wordOffsetInRegister, AccessModeFlags flags)
Specific override which allows to create "DUMMY_INTEERRUPT_X" accessors.
The DummyInterruptTriggerAccessor class.
static void checkSizeIsMultipleOfWordSize(size_t sizeInBytes)
#define CALL_BASE_FUNCTION_TEMPLATE(BaseClass, functionName, templateArgument,...)
Execute the virtual function template call to the base implementation of the function.
NumericAddressedRegisterCatalogue & _registerMap
Base class for address-based device backends (e.g.
#define OVERRIDE_VIRTUAL_FUNCTION_TEMPLATE(BaseClass, functionName)
Save the old vtable to be accessible by CALL_BASE_FUNCTION_TEMPLATE and overwrite it with the new imp...
bool startsWith(const RegisterPath &compare) const
check if the register path starts with the given path
std::unique_ptr< NumericAddressedRegisterCatalogue > _registerMapPointer
Class to store a register path name.
size_t minimumTransferAlignment([[maybe_unused]] uint64_t bar) const override
Determines the supported minimum alignment for any read/write requests.
Set of AccessMode flags with additional functionality for an easier handling.
Exception thrown when a logic error has occured.