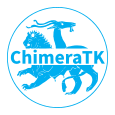 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
17 auto path = registerPathName;
38 auto path = registerPathName;
55 static const std::string DUMMY_INTERRUPT_REGISTER_NAME{
"^/DUMMY_INTERRUPT_([0-9]+)$"};
57 const std::string regPathNameStr{registerPathName};
58 const std::regex dummyInterruptRegex{DUMMY_INTERRUPT_REGISTER_NAME};
60 std::regex_search(regPathNameStr, match, dummyInterruptRegex);
62 if(not match.empty()) {
66 auto primaryInterrupt =
static_cast<unsigned int>(std::stoul(match[1].str()));
68 if(interruptID.front() == primaryInterrupt) {
69 return {
true, primaryInterrupt};
83 std::unique_ptr<BackendRegisterCatalogueBase> c = std::make_unique<DummyBackendRegisterCatalogue>();
NumericAddressedRegisterInfo getBackendRegister(const RegisterPath ®isterPathName) const override
Note: Override this function if backend has "hidden" registers which are not added to the map and hen...
bool hasRegister(const RegisterPath ®isterPathName) const override
Check if register with the given path name exists.
void setAltSeparator(const std::string &altSeparator)
set alternative separator.
bool startsWith(const RegisterPath &compare) const
check if the register path starts with the given path
constexpr auto DUMMY_READABLE_SUFFIX
void fillFromThis(BackendRegisterCatalogue< NumericAddressedRegisterInfo > *target) const
Helper function for clone functions.
std::unique_ptr< BackendRegisterCatalogueBase > clone() const override
Create deep copy of the catalogue.
NumericAddressedRegisterInfo getBackendRegister(const RegisterPath ®isterPathName) const override
Note: Override this function if backend has "hidden" registers which are not added to the map and hen...
Class to store a register path name.
constexpr auto DUMMY_WRITEABLE_SUFFIX
std::pair< bool, int > extractControllerInterrupt(const RegisterPath ®isterPathName) const
bool hasRegister(const RegisterPath ®isterPathName) const override
Check if register with the given path name exists.
std::map< RegisterPath, std::vector< size_t > > _canonicalInterrupts
A canonical interrupt path consists of an exclamation mark, followed by a numeric interrupt and a col...
std::set< std::vector< size_t > > _listOfInterrupts
set of interrupt IDs.