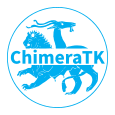 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
22 virtual void send() = 0;
33 virtual const std::string&
getUnit() = 0;
71 explicit AsyncAccessorManager(boost::shared_ptr<DeviceBackend> backend, boost::shared_ptr<Domain> asyncDomain)
79 template<
typename UserType>
80 boost::shared_ptr<AsyncNDRegisterAccessor<UserType>>
subscribe(
134 template<
typename SourceType>
165 template<
typename UserType>
178 boost::weak_ptr<AsyncNDRegisterAccessor<UserType>> _asyncAccessor;
187 template<
typename UserType>
189 auto subscriber = _asyncAccessor.lock();
190 if(subscriber.get() !=
nullptr) {
191 subscriber->sendException(e);
196 template<
typename UserType>
198 return _sendBuffer.value.size();
202 template<
typename UserType>
204 if(_sendBuffer.value.size() > 0) {
205 return _sendBuffer.value[0].size();
211 template<
typename UserType>
213 : _sendBuffer(nChannels, nElements) {}
216 template<
typename UserType>
218 auto subscriber = _asyncAccessor.lock();
219 if(subscriber.get() !=
nullptr) {
220 subscriber->sendDestructively(_sendBuffer);
225 template<
typename UserType>
234 auto newSubscriber = boost::make_shared<AsyncNDRegisterAccessor<UserType>>(
_backend, shared_from_this(),
235 _asyncDomain, name, asyncVariable->getNumberOfChannels(), asyncVariable->getNumberOfSamples(), flags,
236 asyncVariable->getUnit(), asyncVariable->getDescription());
239 newSubscriber->setExceptionBackend(
_backend);
241 asyncVariable->_asyncAccessor = newSubscriber;
245 asyncVariable->send();
248 _asyncVariables[newSubscriber->getId()] = std::move(untypedAsyncVariable);
251 return newSubscriber;
254 template<
typename SourceType>
256 if(!_asyncDomain->unsafeGetIsActive()) {
260 _sourceBuffer = data;
263 if(prepareIntermediateBuffers()) {
264 assert(_delayedUnsubscriptions.empty());
265 for(
auto& var : _asyncVariables) {
266 var.second->fillSendBuffer();
267 _isHoldingDomainLock =
this;
269 _isHoldingDomainLock =
nullptr;
271 for(
auto id : _delayedUnsubscriptions) {
274 _delayedUnsubscriptions.clear();
void distribute(SourceType, VersionNumber version)
virtual unsigned int getNumberOfChannels()=0
Helper functions for the creation of an AsyncNDRegisterAccessor.
The AsyncAccessorManager has three main functionalities:
void unsubscribe(TransferElementID id)
This function must only be called from the destructor of the AsyncNDRegisterAccessor which is created...
size_t wordOffsetInRegister
std::map< TransferElementID, std::unique_ptr< AsyncVariable > > _asyncVariables
AsyncVariableImpl contains a weak pointer to an AsyncNDRegisterAccessor<UserType> and a send buffer N...
virtual void send()=0
Send the value from the _sendBuffer of the AsyncVariableImpl.
virtual void sendException(std::exception_ptr e)=0
Send an exception to all subscribers.
void sendException(const std::exception_ptr &e)
Send an exception to all accessors.
DEFINE_VIRTUAL_FUNCTION_TEMPLATE_VTABLE(createAsyncVariable, std::unique_ptr< AsyncVariable >(AccessorInstanceDescriptor const &))
AsyncVariableImpl(size_t nChannels, size_t nElements)
Helper class to have a complete descriton to create an Accessor.
unsigned int getNumberOfSamples() override
static thread_local AsyncAccessorManager * _isHoldingDomainLock
We have to remember that we are holding the domain lock before we lock a weak pointer to an AsyncNDRe...
virtual ~AsyncAccessorManager()=default
boost::shared_ptr< DeviceBackend > _backend
boost::shared_ptr< Domain > _asyncDomain
void send() final
Send the value from the _sendBuffer of the AsyncVariableImpl.
NDRegisterAccessor< UserType >::Buffer _sendBuffer
virtual const std::string & getDescription()=0
unsigned int getNumberOfChannels() override
Helper functions for the creation of an AsyncNDRegisterAccessor.
virtual bool prepareIntermediateBuffers()
Implement this function in case there is a step between setting the source buffer and filling the use...
virtual ~AsyncVariable()=default
Class to store a register path name.
Class for generating and holding version numbers without exposing a numeric representation.
virtual void fillSendBuffer()=0
Fill the send buffer with data and version number.
virtual unsigned int getNumberOfSamples()=0
#define CALL_VIRTUAL_FUNCTION_TEMPLATE(functionName, templateArgument,...)
Execute the virtual function template call using the vtable defined with the DEFINE_VIRTUAL_FUNCTION_...
Set of AccessMode flags with additional functionality for an easier handling.
Data type to create individual buffers.
Simple class holding a unique ID for a TransferElement.
void unsubscribeImpl(TransferElementID id)
Internal helper function to avoid code duplication.
void sendException(std::exception_ptr e) final
Send an exception to all subscribers.
AsyncAccessorManager(boost::shared_ptr< DeviceBackend > backend, boost::shared_ptr< Domain > asyncDomain)
virtual void asyncVariableMapChanged(TransferElementID)
This virtual function lets derived classes react on subscribe / unsubscribe.
std::list< TransferElementID > _delayedUnsubscriptions
If an unsubscription request is coming in while iterating the _asyncVariables container,...
virtual const std::string & getUnit()=0
boost::shared_ptr< AsyncNDRegisterAccessor< UserType > > subscribe(RegisterPath name, size_t numberOfWords, size_t wordOffsetInRegister, AccessModeFlags flags)
Request a new subscription.