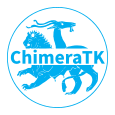 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
10 #include <boost/container/static_vector.hpp>
11 #include <boost/make_shared.hpp>
19 template<
typename UserType>
20 class NDRegisterAccessor :
public TransferElement {
26 std::string
const& description = std::string())
43 UserType&
accessData(
unsigned int channel,
unsigned int sample) {
return buffer_2D[channel][sample]; }
44 const UserType&
accessData(
unsigned int channel,
unsigned int sample)
const {
return buffer_2D[channel][sample]; }
62 const std::type_info&
getValueType()
const override {
return typeid(UserType); }
64 template<
typename COOKED_TYPE>
65 COOKED_TYPE
getAsCooked(
unsigned int channel,
unsigned int sample)
const;
67 template<
typename COOKED_TYPE>
68 COOKED_TYPE
getAsCooked_impl(
unsigned int channel,
unsigned int sample)
const;
70 template<
typename COOKED_TYPE>
71 void setAsCooked(
unsigned int channel,
unsigned int sample, COOKED_TYPE value);
73 template<
typename COOKED_TYPE>
74 void setAsCooked_impl(
unsigned int channel,
unsigned int sample, COOKED_TYPE value);
87 Buffer(
size_t nChannels,
size_t nElements) :
value(nChannels) {
88 for(
auto& channel :
value) channel.resize(nElements);
97 value = std::move(other.value);
104 std::vector<std::vector<UserType>>
value;
134 template<
typename UserType>
135 template<
typename COOKED_TYPE>
140 template<
typename UserType>
141 template<
typename COOKED_TYPE>
146 template<
typename UserType>
147 template<
typename COOKED_TYPE>
152 template<
typename UserType>
153 template<
typename COOKED_TYPE>
155 unsigned int ,
unsigned int , COOKED_TYPE ) {
const std::vector< UserType > & accessChannel(unsigned int channel) const
unsigned int getNumberOfChannels() const
Return number of channels.
ChimeraTK::VersionNumber versionNumber
Version number of this data.
Buffer(Buffer &&other) noexcept
DEFINE_VIRTUAL_FUNCTION_TEMPLATE_VTABLE(getAsCooked_impl, T const(unsigned int, unsigned int))
void setAsCooked_impl(unsigned int channel, unsigned int sample, COOKED_TYPE value)
DEFINE_VIRTUAL_FUNCTION_TEMPLATE_VTABLE_FILLER(NDRegisterAccessor< UserType >, getAsCooked_impl, 2)
COOKED_TYPE getAsCooked(unsigned int channel, unsigned int sample) const
unsigned int getNumberOfSamples() const
Return number of elements per channel.
const std::vector< std::vector< UserType > > & accessChannels() const
#define FILL_VIRTUAL_FUNCTION_TEMPLATE_VTABLE(functionName)
Fill the vtable of a virtual function template defined with DEFINE_VIRTUAL_FUNCTION_TEMPLATE.
std::vector< std::vector< UserType > > buffer_2D
Buffer of converted data elements.
NDRegisterAccessor(std::string const &name, AccessModeFlags accessModeFlags, std::string const &unit=std::string(TransferElement::unitNotSet), std::string const &description=std::string())
Creates an NDRegisterAccessor with the specified name (passed on to the transfer element).
const UserType & accessData(size_t sample) const
UserType & accessData(unsigned int channel, unsigned int sample)
Get or set register accessor's buffer content (2D version).
const UserType & accessData(unsigned int channel, unsigned int sample) const
std::vector< std::vector< UserType > > & accessChannels()
Get or set register accessor's 2D channel vector.
static constexpr char unitNotSet[]
Constant string to be used as a unit when the unit is not provided or known.
void setAsCooked(unsigned int channel, unsigned int sample, COOKED_TYPE value)
Buffer & operator=(Buffer &&other) noexcept
DataValidity
The current state of the data.
boost::shared_ptr< TransferElement > makeCopyRegisterDecorator() override
friend class RegisterAccessor
const std::type_info & getValueType() const override
void makeUniqueId()
Allow generating a unique ID from derived classes.
Base class for register accessors which can be part of a TransferGroup.
COOKED_TYPE getAsCooked_impl(unsigned int channel, unsigned int sample) const
Class for generating and holding version numbers without exposing a numeric representation.
std::vector< std::vector< UserType > > value
The actual data contained in this buffer.
#define CALL_VIRTUAL_FUNCTION_TEMPLATE(functionName, templateArgument,...)
Execute the virtual function template call using the vtable defined with the DEFINE_VIRTUAL_FUNCTION_...
Set of AccessMode flags with additional functionality for an easier handling.
Data type to create individual buffers.
Buffer(size_t nChannels, size_t nElements)
DECLARE_TEMPLATE_FOR_CHIMERATK_USER_TYPES(DummyInterruptTriggerAccessor)
ChimeraTK::DataValidity dataValidity
Whether or not the data in the buffer is considered valid.
N-dimensional register accessor.
std::vector< UserType > & accessChannel(unsigned int channel)
Get or set register accessor's channel vector.
Exception thrown when a logic error has occured.
UserType & accessData(size_t sample)
Get or set register accessor's buffer content (1D version).