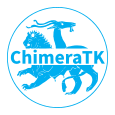 |
ChimeraTK-DeviceAccess
03.18.00
|
TransferElementID & operator=(const TransferElementID &other)=default
Assign ID from another.
bool isValid() const
Check whether the ID is valid.
void makeUnique()
Assign an ID to this instance.
bool operator==(const TransferElementID &other) const
Compare ID with another.
size_t _id
The actual ID value.
TransferElementID()=default
Default constructor constructs an invalid ID, which may be assigned with another ID.
Base class for register accessors which can be part of a TransferGroup.
std::ostream & operator<<(std::ostream &stream, const DataDescriptor::FundamentalType &fundamentalType)
friend std::ostream & operator<<(std::ostream &os, const TransferElementID &me)
Streaming operator to stream the TransferElementID e.g.
bool operator!=(const TransferElementID &other) const
Simple class holding a unique ID for a TransferElement.