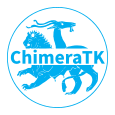 |
ChimeraTK-DeviceAccess
03.18.00
|
void unsubscribe(TransferElementID id)
This function must only be called from the destructor of the AsyncNDRegisterAccessor which is created...
std::map< TransferElementID, std::unique_ptr< AsyncVariable > > _asyncVariables
void sendException(const std::exception_ptr &e)
Send an exception to all accessors.
static thread_local AsyncAccessorManager * _isHoldingDomainLock
We have to remember that we are holding the domain lock before we lock a weak pointer to an AsyncNDRe...
boost::shared_ptr< Domain > _asyncDomain
Simple class holding a unique ID for a TransferElement.
void unsubscribeImpl(TransferElementID id)
Internal helper function to avoid code duplication.
virtual void asyncVariableMapChanged(TransferElementID)
This virtual function lets derived classes react on subscribe / unsubscribe.
std::list< TransferElementID > _delayedUnsubscriptions
If an unsubscription request is coming in while iterating the _asyncVariables container,...