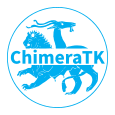 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
4 #define BOOST_TEST_DYN_LINK
5 #define BOOST_TEST_MODULE SubdeviceBackendUnifiedTest
6 #include <boost/test/unit_test.hpp>
7 using namespace boost::unit_test_framework;
11 #include <boost/thread/barrier.hpp>
17 BOOST_AUTO_TEST_SUITE(DoubleBufferingBackendTestSuite)
40 unsigned short goodStep = 100;
41 bool writeCorruptData =
true;
42 unsigned dataCorruptionCount = 0;
45 for(
unsigned t = 0; t < tries; t++) {
46 accessorA.readLatest();
50 int16_t previousVal = 0;
51 for(int16_t val : accessorA) {
53 if(previousVal != 0 && val != (int16_t)(previousVal + goodStep)) {
54 std::cout <<
"found data corruption at index " << i <<
": step from " << previousVal <<
" to " << val
55 <<
" while DAQ fifoStatus=" << fifoStatus << std::endl;
56 if(writeCorruptData) {
58 snprintf(fname,
sizeof(fname),
"corruptData%03i.dat", i);
59 std::cout <<
"writing corrupt data to " << fname << std::endl;
60 std::ofstream f(fname);
61 for(int16_t val1 : accessorA) {
62 f << val1 << std::endl;
66 dataCorruptionCount++;
67 jumpHist[(int16_t)(val - previousVal)]++;
73 boost::this_thread::sleep_for(boost::chrono::milliseconds(std::rand() % 100));
75 return dataCorruptionCount;
78 doubleBufferingEnabled = ena;
79 doubleBufferingEnabled.
write();
83 return writingBufferNum;
86 std::cout <<
"histogram of wrong count-up values in data:" << std::endl;
87 for(
auto p : jumpHist) {
88 std::cout <<
" distance=" << p.first <<
" : " << p.second <<
" times" << std::endl;
102 enableDoubleBuf(
false);
103 unsigned bufferNo = getActiveBufferNo();
105 unsigned dataCorruptionCount = checkDataCorruption(bufferNo == 0 ?
"channel10buf0" :
"channel10buf1", 200);
107 BOOST_CHECK(dataCorruptionCount > 0);
121 unsigned dataCorruptionCount = checkDataCorruption(
"channel10", 1000);
123 BOOST_CHECK(dataCorruptionCount == 0);
128 BOOST_AUTO_TEST_SUITE_END()
bool readLatest()
Read the latest value, discarding any other update since the last read if present.
void enableDoubleBuf(bool ena)
OneDRegisterAccessor< UserType > getOneDRegisterAccessor(const RegisterPath ®isterPathName, size_t numberOfWords=0, size_t wordOffsetInRegister=0, const AccessModeFlags &flags=AccessModeFlags({})) const
Get a OneDRegisterAccessor object for the given register.
unsigned checkDataCorruption(std::string reg, unsigned tries)
unsigned getActiveBufferNo()
void replace(const NDRegisterAccessorAbstractor< UserType > &newAccessor)
Assign a new accessor to this NDRegisterAccessorAbstractor.
ScalarRegisterAccessor< uint32_t > writingBufferNum
Class allows to read/write registers from device.
void open(std::string const &aliasName)
Open a device by the given alias name from the DMAP file.
ScalarRegisterAccessor< UserType > getScalarRegisterAccessor(const RegisterPath ®isterPathName, size_t wordOffsetInRegister=0, const AccessModeFlags &flags=AccessModeFlags({})) const
Get a ScalarRegisterObject object for the given register.
std::map< int16_t, unsigned > jumpHist
BOOST_FIXTURE_TEST_CASE(testWithHardware0, DeviceFixture_HW)
bool write(ChimeraTK::VersionNumber versionNumber={})
Write the data to device.
void setDMapFilePath(std::string dmapFilePath)
Set the location of the dmap file.