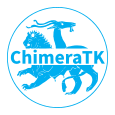 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
13 #include <boost/algorithm/string.hpp>
14 #include <boost/shared_ptr.hpp>
68 explicit Device(
const std::string& aliasName);
73 void open(std::string
const& aliasName);
100 template<
typename UserType>
127 template<
typename UserType>
129 size_t numberOfWords = 0,
size_t wordOffsetInRegister = 0,
145 template<
typename UserType>
147 size_t numberOfElements = 0,
size_t elementsOffset = 0,
168 [[nodiscard]]
bool isOpened()
const;
201 [[nodiscard]] boost::shared_ptr<DeviceBackend>
getBackend();
211 template<
typename UserType>
212 [[nodiscard]] UserType
read(
226 template<
typename UserType>
227 [[nodiscard]] std::vector<UserType>
read(
const RegisterPath& registerPathName,
size_t numberOfWords,
238 template<
typename UserType>
250 template<
typename UserType>
251 void write(
const RegisterPath& registerPathName,
const std::vector<UserType>& vector,
262 template<
typename UserType>
267 _deviceBackendPointer->getRegisterAccessor<UserType>(registerPathName, 1, wordOffsetInRegister, flags));
272 template<
typename UserType>
274 size_t numberOfWords,
size_t wordOffsetInRegister,
const AccessModeFlags& flags)
const {
277 registerPathName, numberOfWords, wordOffsetInRegister, flags));
282 template<
typename UserType>
284 size_t numberOfElements,
size_t elementsOffset,
const AccessModeFlags& flags)
const {
287 registerPathName, numberOfElements, elementsOffset, flags));
292 template<
typename UserType>
294 auto acc = getScalarRegisterAccessor<UserType>(registerPathName, 0, flags);
301 template<
typename UserType>
304 auto acc = getOneDRegisterAccessor<UserType>(registerPathName, numberOfWords, wordOffsetInRegister, flags);
306 std::vector<UserType> vector(acc.getNElements());
313 template<
typename UserType>
315 auto acc = getScalarRegisterAccessor<UserType>(registerPathName, 0, flags);
322 template<
typename UserType>
325 auto acc = getOneDRegisterAccessor<UserType>(registerPathName, vector.size(), wordOffsetInRegister, flags);
329 auto& mutable_vector =
const_cast<std::vector<UserType>&
>(vector);
330 acc.swap(mutable_vector);
332 acc.swap(mutable_vector);
Accessor class to read and write 2D registers.
void close()
Close the device.
OneDRegisterAccessor< UserType > getOneDRegisterAccessor(const RegisterPath ®isterPathName, size_t numberOfWords=0, size_t wordOffsetInRegister=0, const AccessModeFlags &flags=AccessModeFlags({})) const
Get a OneDRegisterAccessor object for the given register.
boost::shared_ptr< DeviceBackend > getBackend()
Obtain the backend.
VoidRegisterAccessor getVoidRegisterAccessor(const RegisterPath ®isterPathName, const AccessModeFlags &flags=AccessModeFlags({})) const
Get a VoidRegisterAccessor object for the given register.
UserType read(const RegisterPath ®isterPathName, const AccessModeFlags &flags=AccessModeFlags({})) const
Inefficient convenience function to read a single-word register without obtaining an accessor.
Device()=default
Create device instance without associating a backend yet.
Accessor class to read and write scalar registers transparently by using the accessor object like a v...
std::string readDeviceInfo() const
Return a device information string.
Catalogue of register information.
Accessor class to read and write registers transparently by using the accessor object like a vector o...
void open()
Re-open the device after previously closeing it by calling close(), or when it was constructed with a...
void activateAsyncRead() noexcept
Activate asyncronous read for all transfer elements where AccessMode::wait_for_new_data is set.
boost::shared_ptr< DeviceBackend > _deviceBackendPointer
Accessor class to read and write void-typed registers.
RegisterCatalogue getRegisterCatalogue() const
Return the register catalogue with detailed information on all registers.
Class allows to read/write registers from device.
Class to store a register path name.
TwoDRegisterAccessor< UserType > getTwoDRegisterAccessor(const RegisterPath ®isterPathName, size_t numberOfElements=0, size_t elementsOffset=0, const AccessModeFlags &flags=AccessModeFlags({})) const
Get a TwoDRegisterAccessor object for the given register.
ScalarRegisterAccessor< UserType > getScalarRegisterAccessor(const RegisterPath ®isterPathName, size_t wordOffsetInRegister=0, const AccessModeFlags &flags=AccessModeFlags({})) const
Get a ScalarRegisterObject object for the given register.
bool isOpened() const
Check if the device is currently opened.
void checkPointersAreNotNull() const
MetadataCatalogue getMetadataCatalogue() const
Return the register catalogue with detailed information on all registers.
Set of AccessMode flags with additional functionality for an easier handling.
void write(const RegisterPath ®isterPathName, UserType value, const AccessModeFlags &flags=AccessModeFlags({}))
Inefficient convenience function to write a single-word register without obtaining an accessor.
bool isFunctional() const
Return wether a device is working as intended, usually this means it is opened and does not have any ...
void setException(const std::string &message)
Set the device into an exception state.