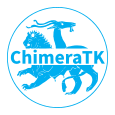 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
9 #include <boost/bind/bind.hpp>
10 #include <boost/enable_shared_from_this.hpp>
11 #include <boost/shared_ptr.hpp>
12 #include <boost/thread.hpp>
13 #include <boost/thread/future.hpp>
23 class PersistentDataStorage;
37 [[nodiscard]]
const std::string&
getName()
const {
return _impl->getName(); }
41 [[nodiscard]]
const std::string&
getUnit()
const {
return _impl->getUnit(); }
166 void replace(boost::shared_ptr<TransferElement> newImpl) {
_impl = std::move(newImpl); }
225 boost::shared_ptr<TransferElement>
_impl;
231 return _impl->writeDestructively(versionNumber);
TransferElementID getId() const
Obtain unique ID for the actual implementation of this TransferElement.
AccessModeFlags getAccessModeFlags() const
Return the AccessModeFlags for this TransferElement.
bool readLatest()
Read the latest value, discarding any other update since the last read if present.
bool writeDestructively(ChimeraTK::VersionNumber versionNumber={})
Just like write(), but allows the implementation to destroy the content of the user buffer in the pro...
bool isReadOnly() const
Check if transfer element is read only, i.e.
bool isWriteable() const
Check if transfer element is writeable.
DataValidity dataValidity() const
Return current validity of the data.
void replace(const TransferElementAbstractor &newAccessor)
Assign a new accessor to this TransferElementAbstractor.
std::vector< boost::shared_ptr< TransferElement > > getHardwareAccessingElements()
Obtain the underlying TransferElements with actual hardware access.
TransferElementAbstractor(boost::shared_ptr< TransferElement > impl)
Construct from TransferElement implementation.
bool readNonBlocking()
Read the next value, if available in the input buffer.
bool isInitialised() const
Return if the accessor is properly initialised.
void setDataValidity(DataValidity valid=DataValidity::ok)
Set the current DataValidity for this TransferElement.
void setPersistentDataStorage(boost::shared_ptr< ChimeraTK::PersistentDataStorage > storage)
Associate a persistent data storage object to be updated on each write operation of this ProcessArray...
const std::string & getDescription() const
Returns the description of this variable/register.
Base class for register accessors abstractors independent of the UserType.
bool isReadable() const
Check if transfer element is readable.
DataValidity
The current state of the data.
TransferElementAbstractor()=default
Create an uninitialised abstractor - just for late initialisation.
const std::string & getUnit() const
Returns the engineering unit.
void replaceTransferElement(const boost::shared_ptr< TransferElement > &newElement)
Search for all underlying TransferElements which are considered identical (see mayReplaceOther()) wit...
std::list< boost::shared_ptr< TransferElement > > getInternalElements()
Obtain the full list of TransferElements internally used by this TransferElement.
const boost::shared_ptr< TransferElement > & getHighLevelImplElement()
Obtain the highest level implementation TransferElement.
void replace(boost::shared_ptr< TransferElement > newImpl)
Alternative signature of relace() with the same functionality, used when a pointer to the implementat...
const std::string & getName() const
Returns the name that identifies the process variable.
void interrupt()
Return from a blocking read immediately and throw boost::thread_interrupted.
Class for generating and holding version numbers without exposing a numeric representation.
const std::type_info & getValueType() const
Returns the std::type_info for the value type of this transfer element.
Set of AccessMode flags with additional functionality for an easier handling.
boost::shared_ptr< TransferElement > _impl
Untyped pointer to implementation.
bool write(ChimeraTK::VersionNumber versionNumber={})
Write the data to device.
Simple class holding a unique ID for a TransferElement.
ChimeraTK::VersionNumber getVersionNumber() const
Returns the version number that is associated with the last transfer (i.e.
void read()
Read the data from the device.