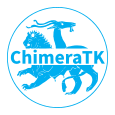 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
4 #define BOOST_TEST_DYN_LINK
6 #define BOOST_TEST_MODULE AsyncVarAndHierarchicalInterruptsUnified
8 #include <boost/test/unit_test.hpp>
9 using namespace boost::unit_test_framework;
23 using ExceptionDummy::ExceptionDummy;
25 static boost::shared_ptr<DeviceBackend>
createInstance(std::string, std::map<std::string, std::string> parameters) {
26 return boost::make_shared<DummyForCleanupCheck>(parameters[
"map"]);
29 std::cout <<
"~DummyForCleanupCheck()" << std::endl;
57 BOOST_AUTO_TEST_SUITE(AsyncVarAndHierarchicalInterruptsUnifiedTestSuite)
61 static std::string
cdd(
"(DummyForCleanupCheck:1?map=testHierarchicalInterrupts.map)");
63 boost::dynamic_pointer_cast<ExceptionDummy>(BackendFactory::getInstance().createBackend(
cdd));
65 template<
class WITHPATH, u
int32_t INTERRUPT>
81 .disableSwitchReadOnly()
82 .disableSwitchWriteOnly()
83 .disableTestWriteNeverLosesData()
84 .enableTestRawTransfer();
88 template<
typename Type>
89 std::vector<std::vector<Type>>
generateValue([[maybe_unused]]
bool raw =
false) {
90 return {{acc +
static_cast<int32_t
>(INTERRUPT)}};
93 template<
typename UserType>
94 std::vector<std::vector<UserType>>
getRemoteValue([[maybe_unused]]
bool raw =
false) {
99 acc = generateValue<minimumUserType>()[0][0];
100 if(!WITHPATH::activeInterruptsPath().empty()) {
102 activeInterrupts = WITHPATH::activeInterruptsValue();
124 static std::string
path() {
return "/datafrom6"; }
130 static std::string
path() {
return "/datafrom5_9"; }
136 static std::string
path() {
return "/datafrom4_8_2"; }
142 activeParentInterrupts = 1U << 8U;
147 static std::string
path() {
return "/datafrom4_8_3"; }
153 activeParentInterrupts = 1U << 8U;
160 template<
class WITHPATH, u
int32_t INTERRUPT>
174 .disableSwitchReadOnly()
175 .disableSwitchWriteOnly()
176 .disableTestWriteNeverLosesData()
177 .disableTestRawTransfer();
179 template<
typename Type>
180 std::vector<std::vector<Type>>
generateValue([[maybe_unused]]
bool raw =
false) {
184 template<
typename UserType>
185 std::vector<std::vector<UserType>>
getRemoteValue([[maybe_unused]]
bool raw =
false) {
190 if(!WITHPATH::activeInterruptsPath().empty()) {
192 activeInterrupts = WITHPATH::activeInterruptsValue();
214 static std::string
path() {
return "/interrupt6"; }
225 static std::string
path() {
return "/!6"; }
232 static std::string
path() {
return "/interrupt5_9"; }
238 static std::string
path() {
return "/!5"; }
245 static std::string
path() {
return "/!5:9"; }
251 static std::string
path() {
return "/interrupt4_8_2"; }
257 static std::string
path() {
return "/!4"; }
264 static std::string
path() {
return "/!4:8"; }
270 static std::string
path() {
return "/!4:8:2"; }
276 static std::string
path() {
return "/interrupt4_8_3"; }
282 static std::string
path() {
return "/!4"; }
289 static std::string
path() {
return "/!4:8"; }
295 static std::string
path() {
return "/!4:8:3"; }
304 static std::string path() {
return "/!4:8"; }
305 static std::string activeInterruptsPath() {
return "/int_ctrls/controller4_8/active_ints"; }
306 static uint32_t activeInterruptsValue() {
return 1U << 3U; }
309 std::cout <<
"*** testRegisterAccessor *** " << std::endl;
313 .addRegister<datafrom5_9>()
315 .addRegister<datafrom4_8_3>()
317 .addRegister<canonicalInterrupt6>()
319 .addRegister<canonicalInterrupt5>()
321 .addRegister<interrupt4_8_2>()
323 .addRegister<canonicalInterrupt4_8a>()
325 .addRegister<interrupt4_8_3>()
327 .addRegister<canonicalInterrupt4_8b>()
338 BOOST_AUTO_TEST_SUITE_END()
static uint32_t activeInterruptsValue()
size_t nRuntimeErrorCases()
static std::string activeInterruptsPath()
void setForceRuntimeError(bool enable, size_t)
static std::string activeInterruptsPath()
size_t nElementsPerChannel()
@ raw
Raw access: disable any possible conversion from the original hardware data type into the given UserT...
std::vector< std::vector< UserType > > getRemoteValue([[maybe_unused]] bool raw=false)
static uint32_t activeInterruptsValue()
static uint32_t activeInterruptsValue()
size_t nRuntimeErrorCases()
~DummyForCleanupCheck() override
static uint32_t activeInterruptsValue()
static BackendFactory & getInstance()
Static function to get an instance of factory.
BOOST_AUTO_TEST_CASE(testRegisterAccessor)
static uint32_t activeInterruptsValue()
static uint32_t activeInterruptsValue()
static uint32_t activeInterruptsValue()
static std::string activeInterruptsPath()
static std::string path()
size_t nRuntimeErrorCases()
static std::string activeInterruptsPath()
static uint32_t activeInterruptsValue()
static std::string activeInterruptsPath()
static uint32_t activeInterruptsValue()
static std::string activeInterruptsPath()
static std::string path()
size_t writeQueueLength()
static std::string activeInterruptsPath()
ChimeraTK::AccessModeFlags supportedFlags()
Descriptor for the test capabilities for each register.
static std::string path()
static std::string path()
static std::string activeInterruptsPath()
void setForceRuntimeError(bool enable, size_t)
static std::string activeInterruptsPath()
static std::string activeInterruptsPath()
static std::string path()
size_t nRuntimeErrorCases()
static std::string path()
static std::string path()
static std::atomic_bool cleanupCalled
static std::string path()
void forceAsyncReadInconsistency()
static uint32_t activeInterruptsValue()
size_t nRuntimeErrorCases()
static boost::shared_ptr< DeviceBackend > createInstance(std::string, std::map< std::string, std::string > parameters)
static std::string activeInterruptsPath()
@ wait_for_new_data
Make any read blocking until new data has arrived since the last read.
minimumUserType rawUserType
static uint32_t activeInterruptsValue()
std::vector< std::vector< UserType > > getRemoteValue([[maybe_unused]] bool raw=false)
static std::string path()
static uint32_t activeInterruptsValue()
static uint32_t activeInterruptsValue()
ChimeraTK::Boolean minimumUserType
static std::string activeInterruptsPath()
static std::string path()
static std::string path()
Class to test any backend for correct behaviour.
size_t nRuntimeErrorCases()
static std::string path()
static std::string activeInterruptsPath()
static std::string path()
constexpr TestCapabilities< _syncRead, TestCapability::disabled, _asyncReadInconsistency, _switchReadOnly, _switchWriteOnly, _writeNeverLosesData, _testWriteOnly, _testReadOnly, _testRawTransfer, _testCatalogue, _setRemoteValueIncrementsVersion > disableForceDataLossWrite() const
static uint32_t activeInterruptsValue()
ChimeraTK::Void rawUserType
static std::string path()
static std::string activeInterruptsPath()
size_t writeQueueLength()
static uint32_t activeInterruptsValue()
std::vector< std::vector< Type > > generateValue([[maybe_unused]] bool raw=false)
void forceAsyncReadInconsistency()
static std::string activeInterruptsPath()
ChimeraTK::AccessModeFlags supportedFlags()
static std::string path()
Set of AccessMode flags with additional functionality for an easier handling.
static std::string path()
void registerBackendType(const std::string &backendType, boost::shared_ptr< DeviceBackend >(*creatorFunction)(std::string address, std::map< std::string, std::string > parameters), const std::vector< std::string > &sdmParameterNames={}, const std::string &deviceAccessVersion=CHIMERATK_DEVICEACCESS_VERSION)
Register a backend by the name backendType with the given creatorFunction.
static uint32_t activeInterruptsValue()
static uint32_t activeInterruptsValue()
static std::string activeInterruptsPath()
static std::string activeInterruptsPath()
Wrapper Class to avoid vector<bool> problems.
std::vector< std::vector< Type > > generateValue([[maybe_unused]] bool raw=false)
size_t nRuntimeErrorCases()
size_t nElementsPerChannel()
UnifiedBackendTest< typename boost::mpl::push_back< VECTOR_OF_REGISTERS_T, REG_T >::type > addRegister()
Add a register to be used by the test.
static std::string path()