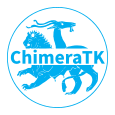 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
12 class SubdeviceRegisterAccessor;
63 void close()
override;
70 std::string address, std::map<std::string, std::string> parameters);
122 size_t wordOffsetInRegister,
bool enforceAlignment);
124 template<
typename UserType>
130 template<
typename UserType>
135 template<
typename UserType>
137 const RegisterPath& registerPathName,
size_t numberOfWords,
size_t wordOffsetInRegister,
152 size_t numberOfWords,
size_t wordOffsetInRegister,
AccessModeFlags flags);
size_t timeout
timeout (in milliseconds), used in threeRegisters to throw a runtime_error if status register stuck a...
static boost::shared_ptr< DeviceBackend > createInstance(std::string address, std::map< std::string, std::string > parameters)
Backend for subdevices which are passed through some register or area of another device (subsequently...
void setExceptionImpl() noexcept override
Function to be (optionally) implemented by backends if additional actions are needed when switching t...
void activateAsyncRead() noexcept override
Activate asyncronous read for all transfer elements where AccessMode::wait_for_new_data is set.
std::string targetAddress
for type == threeRegisters or twoRegisters: the name of the target registers
std::string targetAlias
the target device name
void close() override
Close the device.
std::string targetControl
MetadataCatalogue getMetadataCatalogue() const override
Return the device metadata catalogue.
void obtainTargetBackend()
obtain the target backend if not yet done
std::string readDeviceInfo() override
Return a device information string containing hardware details like the firmware version number or th...
static void verifyRegisterAccessorSize(const NumericAddressedRegisterInfo &info, size_t &numberOfWords, size_t wordOffsetInRegister, bool enforceAlignment)
Check consistency of the passed sizes and offsets against the information in the map file Will adjust...
NumericAddressedRegisterCatalogue _registerMap
map from register names to addresses
Catalogue of register information.
MetadataCatalogue _metadataCatalogue
boost::shared_ptr< NDRegisterAccessor< UserType > > getRegisterAccessor_area(const RegisterPath ®isterPathName, size_t numberOfWords, size_t wordOffsetInRegister, AccessModeFlags flags)
getRegisterAccessor implemenation for area types
boost::shared_ptr< NDRegisterAccessor< UserType > > getRegisterAccessor_synchronized(const RegisterPath ®isterPathName, size_t numberOfWords, size_t wordOffsetInRegister, const AccessModeFlags &flags)
getRegisterAccessor implemenation for threeRegisters types
RegisterCatalogue getRegisterCatalogue() const override
Return the register catalogue with detailed information on all registers.
size_t sleepTime
for type == threeRegisters or twoRegisters: sleep time of polling loop resp. between operations,...
Type type
type of the subdeivce
DeviceBackendImpl implements some basic functionality which should be available for all backends.
DEFINE_VIRTUAL_FUNCTION_TEMPLATE_VTABLE_FILLER(SubdeviceBackend, getRegisterAccessor_impl, 4)
boost::shared_ptr< SubdeviceRegisterAccessor > getRegisterAccessor_helper(const NumericAddressedRegisterInfo &info, size_t numberOfWords, size_t wordOffsetInRegister, AccessModeFlags flags)
Class to store a register path name.
SubdeviceBackend(std::map< std::string, std::string > parameters)
std::mutex mutex
Mutex to deal with concurrent access to the device.
Set of AccessMode flags with additional functionality for an easier handling.
boost::shared_ptr< NDRegisterAccessor< UserType > > getRegisterAccessor_impl(const RegisterPath ®isterPathName, size_t numberOfWords, size_t wordOffsetInRegister, AccessModeFlags flags)
boost::shared_ptr< ChimeraTK::DeviceBackend > targetDevice
The target device backend itself.
void open() override
Open the device.
std::string targetArea
for type == area: the name of the target register
size_t addressToDataDelay
for type == threeRegisters or twoRegisters: sleep time between address and data write