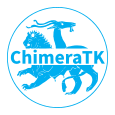 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
8 #include <boost/shared_ptr.hpp>
14 class BackendRegisterCatalogueBase;
15 class const_RegisterCatalogueImplIterator;
53 explicit const_iterator(std::unique_ptr<const_RegisterCatalogueImplIterator> it);
71 std::unique_ptr<const_RegisterCatalogueImplIterator>
_impl;
79 std::unique_ptr<BackendRegisterCatalogueBase>
_impl;
98 [[nodiscard]]
virtual bool isEqual(
99 const std::unique_ptr<const_RegisterCatalogueImplIterator>& rightHandSide)
const = 0;
105 [[nodiscard]]
virtual std::unique_ptr<const_RegisterCatalogueImplIterator>
clone()
const = 0;
Const iterator for iterating through the registers in the catalogue.
virtual void decrement()=0
const BackendRegisterInfoBase * operator->()
std::unique_ptr< BackendRegisterCatalogueBase > _impl
bool operator!=(const const_iterator &rightHandSide) const
virtual const BackendRegisterInfoBase * get()=0
virtual bool isEqual(const std::unique_ptr< const_RegisterCatalogueImplIterator > &rightHandSide) const =0
bool hasRegister(const RegisterPath ®isterPathName) const
Check if register with the given path name exists.
const_iterator begin() const
Return iterators for iterating through the registers in the catalogue.
const_iterator(std::unique_ptr< const_RegisterCatalogueImplIterator > it)
virtual std::unique_ptr< const_RegisterCatalogueImplIterator > clone() const =0
Create copy of the iterator.
const_iterator end() const
RegisterCatalogue(std::unique_ptr< BackendRegisterCatalogueBase > &&impl)
Catalogue of register information.
const_iterator & operator--()
size_t getNumberOfRegisters() const
Get number of registers in the catalogue.
Virtual base class for the catalogue const iterator.
RegisterInfo getRegister(const RegisterPath ®isterPathName) const
Get register information for a given full path name.
std::unique_ptr< const_RegisterCatalogueImplIterator > _impl
const_iterator & operator=(const const_iterator &other)
RegisterCatalogue & operator=(const RegisterCatalogue &other)
virtual ~const_RegisterCatalogueImplIterator()=default
const_iterator & operator++()
bool operator==(const const_iterator &rightHandSide) const
const BackendRegisterInfoBase & operator*()
virtual void increment()=0
const BackendRegisterCatalogueBase & getImpl() const
Return a const reference to the implementation object.
Class to store a register path name.
DeviceBackend-independent register description.
Pure virtual implementation base class for the register catalogue.