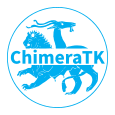 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
32 _impl = std::move(other._impl);
44 return _impl->getRegister(registerPathName);
50 return _impl->hasRegister(registerPathName);
56 return _impl->getNumberOfRegisters();
74 : _impl(std::move(it)) {}
109 return *(
_impl->get());
Const iterator for iterating through the registers in the catalogue.
const BackendRegisterInfoBase * operator->()
std::unique_ptr< BackendRegisterCatalogueBase > _impl
bool operator!=(const const_iterator &rightHandSide) const
bool hasRegister(const RegisterPath ®isterPathName) const
Check if register with the given path name exists.
const_iterator begin() const
Return iterators for iterating through the registers in the catalogue.
const_iterator(std::unique_ptr< const_RegisterCatalogueImplIterator > it)
const_iterator end() const
RegisterCatalogue(std::unique_ptr< BackendRegisterCatalogueBase > &&impl)
Catalogue of register information.
const_iterator & operator--()
size_t getNumberOfRegisters() const
Get number of registers in the catalogue.
RegisterInfo getRegister(const RegisterPath ®isterPathName) const
Get register information for a given full path name.
std::unique_ptr< const_RegisterCatalogueImplIterator > _impl
RegisterCatalogue & operator=(const RegisterCatalogue &other)
const_iterator & operator++()
bool operator==(const const_iterator &rightHandSide) const
const BackendRegisterInfoBase & operator*()
const BackendRegisterCatalogueBase & getImpl() const
Return a const reference to the implementation object.
Class to store a register path name.
DeviceBackend-independent register description.
Pure virtual implementation base class for the register catalogue.