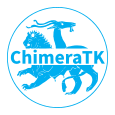 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
28 inline operator T()
const {
return fpcptr->template scalarToCooked<T>(*
buffer); }
91 auto* basePtr =
reinterpret_cast<std::byte*
>(
buffer);
92 auto* startAddress = basePtr +
static_cast<size_t>(
pitch) * sample;
139 :
_dev(dev),
_path(module +
"/" + name),
fpc(module +
"/" + name) {
221 class DummyMultiplexedRegisterAccessor {
230 :
_dev(dev),
_path(module +
"/" + name) {
238 assert(c.bitOffset % 8 == 0);
240 nbytes.push_back((c.width - 1) / 8 + 1);
276 auto* seq =
reinterpret_cast<int32_t*
>(basePtr +
offsets[sequence]);
299 std::vector<FixedPointConverter>
fpc;
332 boost::shared_ptr<DeviceBackend>
const& backend, std::string
const& module, std::string
const& name)
DummyBackend & getBackend() const
Return the backend.
unsigned int getNumberOfElements() const
return number of elements
uint64_t address
Lower part of the address relative to BAR, in bytes.
int nbytes
number of bytes per word
unsigned int getNumberOfElements()
return number of elements per sequence
T operator++(int)
post-increment operator
void operator=(const DummyRegisterSequence &rightHandSide) const =delete
remove assignment operator since it will be confusing */
int32_t * getElement(unsigned int index)
return element
proxies::DummyRegisterElement< T > getProxy(int index)
return a proxy object
@ raw
Raw access: disable any possible conversion from the original hardware data type into the given UserT...
RegisterPath _path
path of the register
NumericAddressedRegisterInfo getBackendRegister(const RegisterPath ®isterPathName) const override
Note: Override this function if backend has "hidden" registers which are not added to the map and hen...
void operator=(const DummyMultiplexedRegisterAccessor &rightHandSide) const =delete
remove assignment operator since it will be confusing
boost::shared_ptr< DummyBackend > _backend
pointer to dummy backend
Temporary proxy class for sequences, used in the DummyMultiplexedRegister class.
int32_t * buffer
raw buffer of this accessor
Register accessor for accessing single word or 1D array registers internally of a DummyBackend implem...
NumericAddressedRegisterInfo registerInfo
register map information
uint32_t nElements
Number of elements in register.
Register accessor for accessing multiplexed 2D array registers internally of a DummyBackend implement...
DummyBackend & getBackend() const
Return the backend.
NumericAddressedRegisterInfo registerInfo
register map information
void setWriteCallbackFunction(AddressRange addressRange, boost::function< void(void)> const &writeCallbackFunction)
T operator--(int)
post-decrement operator
FixedPointConverter * fpcptr
fixed point converter to be used for this element
int32_t & operator[](unsigned int index)
Get or set register content by [] operator.
DummyRegisterRawAccessor & operator=(int32_t rhs)
RegisterPath _path
path of the register
void operator=(const DummyRegisterAccessor &rightHandSide) const =delete
remove assignment operator since it will be confusing */
DummyRegisterElement(FixedPointConverter *_fpc, int _nbytes, int32_t *_buffer)
unsigned int nElements
number of elements per sequence
std::unique_lock< std::mutex > getBufferLock()
Get a lock to safely modify the buffer in a multi-treaded environment.
std::vector< uint32_t > nbytes
number of bytes per word for sequences
DummyRegisterElement()
constructor when used as a base class in DummyRegister
int nbytes
number of bytes per word
FixedPointConverter * fpcptr
fixed point converter to be used for this sequence
NumericAddressedRegisterCatalogue & _registerMap
proxies::DummyRegisterSequence< T > operator[](unsigned int sequence)
Get or set register content by [] operators.
const NumericAddressedRegisterInfo & getRegisterInfo()
unsigned int getNumberOfSequences()
return number of sequences
void operator=(const DummyRegisterRawAccessor &rightHandSide) const =delete
remove assignment operator since it will be confusing
proxies::DummyRegisterElement< T > operator[](unsigned int index)
Get or set register content by [] operator.
uint64_t bar
Upper part of the address (name originally from PCIe, meaning now generalised)
DummyRegisterRawAccessor(boost::shared_ptr< DeviceBackend > const &backend, std::string const &module, std::string const &name)
int32_t * buffer
reference to the raw buffer (first word of the sequence)
std::map< uint64_t, std::vector< int32_t > > _barContents
DummyRegisterElement< T > operator++()
pre-increment operator
int pitch
pitch in bytes (distance between samples of the same sequence)
const RegisterPath & getRegisterPath() const
Return the register path.
Accessor for raw 32 bit integer access to the underlying memory space.
unsigned int getNumberOfElements()
return number of elements
DummyRegisterElement< T > operator[](unsigned int sample)
Get or set register content by [] operator.
DummyRegisterAccessor(DummyBackend *dev, std::string const &module, std::string const &name)
Constructor should normally be called in the constructor of the DummyBackend implementation.
std::vector< ChannelInfo > channels
Define per-channel information (bit interpretation etc.), 1D/scalars have exactly one entry.
Temporary proxy class for use in the DummyRegister and DummyMultiplexedRegister classes.
const NumericAddressedRegisterInfo & getRegisterInfo()
NumericAddressedRegisterInfo registerInfo
register map information
std::vector< uint32_t > offsets
offsets in bytes for sequences
uint32_t elementPitchBits
Distance in bits (!) between two elements (of the same channel)
std::vector< FixedPointConverter > fpc
pointer to fixed point converter
Class to store a register path name.
DummyMultiplexedRegisterAccessor(DummyBackend *dev, std::string const &module, std::string const &name)
Constructor should normally be called in the constructor of the DummyBackend implementation.
DummyRegisterSequence(FixedPointConverter *_fpc, int _nbytes, int _pitch, int32_t *_buffer)
The fixed point converter provides conversion functions between a user type and up to 32 bit fixed po...
int pitch
pitch in bytes (distance between samples of the same sequence)
The dummy device opens a mapping file instead of a device, and implements all registers defined in th...
DummyRegisterElement< T > operator--()
pre-decrement operator
uint32_t toRaw(UserType cookedValue) const
Conversion function from type T to fixed point.
const RegisterPath & getRegisterPath() const
Return the register path.
DummyBackend * _dev
pointer to VirtualDevice
FixedPointConverter fpc
fixed point converter
void setWriteCallback(const std::function< void()> &writeCallback)
Set callback function which is called when the register is written to (through the normal Device inte...
DummyBackend * _dev
pointer to VirtualDevice
DummyRegisterElement< T > & operator=(T rhs)
assignment operator
Exception thrown when a logic error has occured.
std::unique_lock< std::mutex > getBufferLock()
Get a lock to safely modify the buffer.
int32_t * buffer
raw buffer of this element