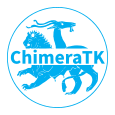 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
51 std::string path_alt =
path.substr(1);
52 while((pos = path_alt.find(std::string(
separator))) != std::string::npos) {
67 path += rightHandSide;
88 if(found_alt != std::string::npos) found = std::max(found, found_alt);
90 if(found != std::string::npos && found > 0) {
103 std::size_t found =
path.find_first_of(std::string(
separator), 1);
107 if(found != std::string::npos) {
138 [[nodiscard]]
size_t length()
const {
return path.length(); }
145 return pathConverted.substr(0, compare.
length()) == otherPathConverted;
153 if(pathConverted.size() < compare.
length())
return false;
154 return pathConverted.substr(pathConverted.size() - compare.
length()) == otherPathConverted;
159 std::vector<std::string> components;
160 if(
path.length() <= 1)
return components;
162 while(pos != std::string::npos) {
166 if(npos_alt != std::string::npos && npos_alt < npos) npos = npos_alt;
168 components.push_back(
path.substr(pos + 1, npos - pos - 1));
199 while((pos =
string.find(std::string(sep) + sep)) != std::string::npos) {
200 string.erase(pos, 1);
202 if(
string.
length() > 1 &&
string.substr(
string.
length() - 1, 1) == sep)
string.erase(
string.
length() - 1, 1);
210 if(otherSeparator.length() == 0)
return path;
212 std::string path_other =
path;
214 while((pos = path_other.find(otherSeparator)) != std::string::npos) {
215 path_other.replace(pos, 1, std::string(
separator));
227 if(sepalt.length() == 0) {
233 "when both have different alternative "
245 RegisterPath
operator/(
const RegisterPath& leftHandSide,
const RegisterPath& rightHandSide);
248 std::string
operator+(
const std::string& leftHandSide,
const RegisterPath& rightHandSide);
249 RegisterPath
operator+(
const RegisterPath& leftHandSide,
const std::string& rightHandSide);
252 RegisterPath
operator/(
const RegisterPath& leftHandSide,
int rightHandSide);
253 RegisterPath
operator*(
const RegisterPath& leftHandSide,
int rightHandSide);
256 std::ostream&
operator<<(std::ostream& os,
const RegisterPath& me);
void removeExtraSeparators()
Search for duplicate separators (e.g.
std::string getWithOtherSeparatorReplaced(const std::string &otherSeparator) const
Return the path after replacing the given otherSeparator with the standard separator "/".
RegisterPath & operator--()
Cut-left operator, e.g. –registerPath.
RegisterPath & operator--(int)
Cut-right operator, e.g. registerPath–.
bool operator==(const std::string &rightHandSide) const
comparison with std::string
friend RegisterPath operator+(const RegisterPath &leftHandSide, const std::string &rightHandSide)
RegisterPath & operator/=(const std::string &rightHandSide)
/= operator: modify this object by adding a new element to this path
friend RegisterPath operator*(const RegisterPath &leftHandSide, int rightHandSide)
void setAltSeparator(const std::string &altSeparator)
set alternative separator.
std::string getWithAltSeparator() const
obtain path with alternative separator character instead of "/".
RegisterPath operator*(const RegisterPath &leftHandSide, int rightHandSide)
std::string operator+(const std::string &leftHandSide, const RegisterPath &rightHandSide)
non-member + operator for RegisterPath: concatenate with normal strings.
bool endsWith(const RegisterPath &compare) const
check if the register path ends with the given path component(s)
RegisterPath & operator=(const RegisterPath &_path)=default
bool operator==(const char *rightHandSide) const
comparison with char*
RegisterPath operator/(const RegisterPath &leftHandSide, const RegisterPath &rightHandSide)
non-member / operator: add a new element to the path.
RegisterPath(const std::string &_path)
bool operator!=(const char *rightHandSide) const
comparison with char*
friend std::ostream & operator<<(std::ostream &os, const RegisterPath &me)
streaming operator
static const char separator[]
separator character to separate the elements in the path
friend RegisterPath operator/(const RegisterPath &leftHandSide, const RegisterPath &rightHandSide)
non-member / operator: add a new element to the path.
std::string getCommonAltSeparator(const RegisterPath &otherPath) const
return common alternative separator for this RegisterPath and the specified other RegisterPath object...
bool startsWith(const RegisterPath &compare) const
check if the register path starts with the given path
bool operator<(const RegisterPath &rightHandSide) const
< operator: comparison used for sorting e.g. in std::map
size_t length() const
return the length of the path (including leading slash)
bool operator==(const RegisterPath &rightHandSide) const
comparison with other RegisterPath
RegisterPath(const char *_path)
RegisterPath(const RegisterPath &_path)
bool operator!=(const std::string &rightHandSide) const
comparison with std::string
Class to store a register path name.
std::vector< std::string > getComponents() const
split path into components
std::ostream & operator<<(std::ostream &stream, const DataDescriptor::FundamentalType &fundamentalType)
bool operator!=(const RegisterPath &rightHandSide) const
comparison with other RegisterPath
static std::string removeExtraSeparators(std::string string, const std::string &sep=separator)
Search for duplicate separators (e.g.
std::string separator_alt
altenative separator character
std::string path
path in standardised notation
RegisterPath & operator+=(const std::string &rightHandSide)
+= operator: just concatenate-assign like normal strings
Exception thrown when a logic error has occured.