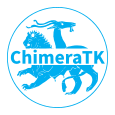 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
27 return leftHandSide + ((std::string)rightHandSide);
34 ret.
path += rightHandSide;
57 return os << std::string(me);
void removeExtraSeparators()
Search for duplicate separators (e.g.
void setAltSeparator(const std::string &altSeparator)
set alternative separator.
RegisterPath operator*(const RegisterPath &leftHandSide, int rightHandSide)
std::string operator+(const std::string &leftHandSide, const RegisterPath &rightHandSide)
non-member + operator for RegisterPath: concatenate with normal strings.
RegisterPath operator/(const RegisterPath &leftHandSide, const RegisterPath &rightHandSide)
non-member / operator: add a new element to the path.
static const char separator[]
separator character to separate the elements in the path
std::string getCommonAltSeparator(const RegisterPath &otherPath) const
return common alternative separator for this RegisterPath and the specified other RegisterPath object...
Class to store a register path name.
std::ostream & operator<<(std::ostream &stream, const DataDescriptor::FundamentalType &fundamentalType)
std::string separator_alt
altenative separator character
std::string to_string(Boolean &value)
std::string path
path in standardised notation