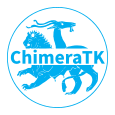 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
10 size_t nFractionalDigits_,
DataType rawDataType_,
DataType transportLayerDataType_)
11 : _fundamentalType(fundamentalType_), _rawDataType(rawDataType_), _transportLayerDataType(transportLayerDataType_),
12 _isIntegral(isIntegral_), _isSigned(isSigned_), _nDigits(nDigits_), _nFractionalDigits(nFractionalDigits_) {}
28 _nFractionalDigits = 0;
56 _nFractionalDigits = 45;
60 _nFractionalDigits = 325;
73 : _fundamentalType(
FundamentalType::undefined), _isIntegral(false), _isSigned(false), _nDigits(0),
74 _nFractionalDigits(0) {}
79 return _fundamentalType;
107 return _nFractionalDigits;
123 return _transportLayerDataType;
129 return _fundamentalType == other._fundamentalType && _rawDataType == other._rawDataType &&
130 _transportLayerDataType == other._transportLayerDataType && _isIntegral == other._isIntegral &&
131 _isSigned == other._isSigned && _nDigits == other._nDigits && _nFractionalDigits == other._nFractionalDigits;
147 return typeid(int64_t);
150 return typeid(int32_t);
153 return typeid(int16_t);
155 return typeid(int8_t);
158 return typeid(uint64_t);
161 return typeid(uint32_t);
164 return typeid(uint16_t);
166 return typeid(uint8_t);
172 size_t floatMaxDigits = 4 +
174 std::log10(std::numeric_limits<float>::max()), -std::log10(std::numeric_limits<float>::denorm_min())));
175 if(
nDigits() > floatMaxDigits) {
176 return typeid(double);
178 return typeid(float);
184 return typeid(std::string);
209 stream <<
"undefined";
@ uint8
Unsigned 8 bit integer.
bool isIntegral() const
Return whether the raw data type is an integer.
@ float64
Double precision float.
bool isSigned() const
Return whether the raw data type is signed.
@ float32
Single precision float.
bool operator!=(const DataDescriptor &other) const
@ int32
Signed 32 bit integer.
void setRawDataType(const DataType &d)
Set the raw data type.
DataType transportLayerDataType() const
Get the data type on the transport layer.
DataType minimumDataType() const
Get the minimum data type required to represent the described data type in the host CPU.
@ uint32
Unsigned 32 bit integer.
Class describing the actual payload data format of a register in an abstract manner.
FundamentalType fundamentalType() const
Get the fundamental data type.
size_t nFractionalDigits() const
Approximate maximum number of digits after decimal dot (of base 10) needed to represent the value (ex...
bool isSigned() const
Return whether the data is signed or not.
bool operator==(const DataDescriptor &other) const
@ uint16
Unsigned 16 bit integer.
bool isNumeric() const
Returns whether the data type is numeric.
A class to describe which of the supported data types is used.
DataType rawDataType() const
Get the raw data type.
@ int64
Signed 64 bit integer.
FundamentalType
Enum for the fundamental data types.
@ int8
Signed 8 bit integer.
std::ostream & operator<<(std::ostream &stream, const DataDescriptor::FundamentalType &fundamentalType)
bool isIntegral() const
Return whether the data is integral or not (e.g.
size_t nDigits() const
Return the approximate maximum number of digits (of base 10) needed to represent the value (including...
DataDescriptor()
Default constructor sets fundamental type to "undefined".
@ int16
Signed 16 bit integer.
Wrapper Class to avoid vector<bool> problems.
@ uint64
Unsigned 64 bit integer.