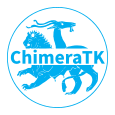 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
23 template<
typename UserType,
typename TAG = std::
nullptr_t>
45 operator UserType&() {
return get()->accessData(0, 0); }
49 operator const UserType&()
const {
return get()->accessData(0, 0); }
55 template<
typename OTHER_TAG>
63 template<
typename OTHER_TAG>
89 template<
typename COOKED_TYPE>
97 template<
typename COOKED_TYPE>
112 void setAndWrite(UserType newValue, VersionNumber versionNumber = {});
136 operator std::string&();
145 template<
typename COOKED_TYPE>
153 template<
typename COOKED_TYPE>
195 operator const bool&()
const {
return get()->accessData(0, 0); }
201 operator bool&() {
return get()->accessData(0, 0); }
207 template<
typename UserType,
typename TAG>
210 static_assert(!std::is_same<UserType, Void>::value,
211 "You cannot create ScalarRegisterAccessor<ChimeraTK::Void>! Use VoidRegisterAccessor instead.");
216 template<
typename UserType,
typename TAG>
218 static_assert(!std::is_same<UserType, Void>::value,
219 "You cannot create ScalarRegisterAccessor<ChimeraTK::Void>! Use VoidRegisterAccessor instead.");
224 template<
typename UserType,
typename TAG>
225 template<
typename OTHER_TAG>
233 template<
typename UserType,
typename TAG>
235 get()->accessData(0, 0) = rightHandSide;
241 template<
typename UserType,
typename TAG>
243 UserType v = get()->accessData(0, 0);
250 template<
typename UserType,
typename TAG>
252 UserType v = get()->accessData(0, 0);
259 template<
typename UserType,
typename TAG>
260 template<
typename COOKED_TYPE>
262 return get()->template getAsCooked<COOKED_TYPE>(0, 0);
267 template<
typename UserType,
typename TAG>
268 template<
typename COOKED_TYPE>
270 return get()->template setAsCooked<COOKED_TYPE>(0, 0, value);
275 template<
typename UserType,
typename TAG>
278 if(get()->accessData(0, 0) != newValue || this->getVersionNumber() ==
VersionNumber(
nullptr) ||
279 this->dataValidity() != validity) {
284 this->setDataValidity(validity);
285 this->
write(versionNumber);
291 template<
typename UserType,
typename TAG>
294 this->
write(versionNumber);
299 template<
typename UserType,
typename TAG>
302 return get()->accessData(0, 0);
315 return boost::static_pointer_cast<NDRegisterAccessor<std::string>>(_impl)->accessData(0, 0);
321 std::string rightHandSide) {
322 boost::static_pointer_cast<NDRegisterAccessor<std::string>>(
_impl)->accessData(0, 0) = std::move(rightHandSide);
328 template<
typename COOKED_TYPE>
330 return get()->template getAsCooked<COOKED_TYPE>(0, 0);
335 template<
typename COOKED_TYPE>
337 return get()->template setAsCooked<COOKED_TYPE>(0, 0, value);
347 if(versionNumber ==
VersionNumber{
nullptr}) versionNumber = {};
349 this->
write(versionNumber);
358 this->
write(versionNumber);
365 return get()->accessData(0, 0);
void setAsCooked(COOKED_TYPE value)
Set the cooked values in case the accessor is a raw accessor (which does not do data conversion).
DataValidity dataValidity() const
Return current validity of the data.
COOKED_TYPE getAsCooked()
Get the cooked values in case the accessor is a raw accessor (which does not do data conversion).
ScalarRegisterAccessor & operator++()
Pre-increment operator for the first element.
void setAndWrite(UserType newValue, VersionNumber versionNumber={})
Convenience function to set and write new value.
void writeIfDifferent(UserType newValue, VersionNumber versionNumber=VersionNumber{nullptr}, DataValidity validity=DataValidity::ok)
Convenience function to set and write new value if it differes from the current value.
UserType readAndGet()
Convenience function to read and return a value of UserType.
void setDataValidity(DataValidity valid=DataValidity::ok)
Set the current DataValidity for this TransferElement.
Accessor class to read and write scalar registers transparently by using the accessor object like a v...
Group multiple data accessors to efficiently trigger data transfers on the whole group.
ScalarRegisterAccessor & operator=(UserType rightHandSide)
Assignment operator, assigns the first element.
DataValidity
The current state of the data.
ScalarRegisterAccessor()
Placeholder constructor, to allow late initialisation of the accessor, e.g.
Base class for the register accessor abstractors (ScalarRegisterAccessor, OneDRegisterAccessor and Tw...
ScalarRegisterAccessor & operator--()
Pre-decrement operator for the first element.
NDRegisterAccessor< UserType > * get()
Class for generating and holding version numbers without exposing a numeric representation.
boost::shared_ptr< TransferElement > _impl
Untyped pointer to implementation.
bool write(ChimeraTK::VersionNumber versionNumber={})
Write the data to device.
ChimeraTK::VersionNumber getVersionNumber() const
Returns the version number that is associated with the last transfer (i.e.
void read()
Read the data from the device.
Wrapper Class to avoid vector<bool> problems.
N-dimensional register accessor.