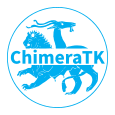 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
7 #include <boost/algorithm/string.hpp>
8 #include <boost/array.hpp>
9 #include <boost/asio.hpp>
10 #include <boost/chrono.hpp>
11 #include <boost/make_shared.hpp>
12 #include <boost/shared_ptr.hpp>
13 #include <boost/thread.hpp>
59 RebotBackend(std::string boardAddr, std::string port,
const std::string& mapFileName =
"",
65 void read(uint8_t bar, uint32_t addressInBytes, int32_t* data,
size_t sizeInBytes)
override;
66 void write(uint8_t bar, uint32_t addressInBytes, int32_t
const* data,
size_t sizeInBytes)
override;
70 std::string address, std::map<std::string, std::string> parameters);
75 void heartbeatLoop(
const boost::shared_ptr<ThreadInformerMutex>& threadInformerMutex);
void open() override
The function opens the connection to the device.
void write(uint8_t bar, uint32_t addressInBytes, int32_t const *data, size_t sizeInBytes) override
void read(uint8_t bar, uint32_t addressInBytes, int32_t *data, size_t sizeInBytes) override
boost::shared_ptr< ThreadInformerMutex > _threadInformerMutex
Handles the communication over TCP protocol with RebotDevice-based devices.
Base class for address-based device backends (e.g.
boost::thread _heartbeatThread
size_t minimumTransferAlignment([[maybe_unused]] uint64_t bar) const override
Determines the supported minimum alignment for any read/write requests.
RebotBackend(std::string boardAddr, std::string port, const std::string &mapFileName="", uint32_t connectionTimeout_sec=DEFAULT_CONNECTION_TIMEOUT_sec)
void heartbeatLoop(const boost::shared_ptr< ThreadInformerMutex > &threadInformerMutex)
void closeImpl() override
All backends derrived from NumericAddressedBackend must implement closeImpl() instead of close.
std::string readDeviceInfo() override
Return a device information string containing hardware details like the firmware version number or th...
This is the base class of the code which implements the ReboT protocol.
boost::chrono::steady_clock::time_point _lastSendTime
The time when the last command (read/write/heartbeat) was send.
boost::shared_ptr< Rebot::Connection > _connection
static boost::shared_ptr< DeviceBackend > createInstance(std::string address, std::map< std::string, std::string > parameters)
const static uint32_t DEFAULT_CONNECTION_TIMEOUT_sec
unsigned int _connectionTimeout
std::unique_ptr< RebotProtocolImplementor > _protocolImplementor