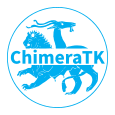 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
7 #include <boost/make_shared.hpp>
14 class MathPluginFormulaHelper;
24 template<
typename UserType,
typename TargetType>
26 boost::shared_ptr<LogicalNameMappingBackend>& backend,
29 void openHook(
const boost::shared_ptr<LogicalNameMappingBackend>& backend)
override;
30 void postParsingHook(
const boost::shared_ptr<const LogicalNameMappingBackend>& backend)
override;
36 boost::shared_ptr<MathPluginFormulaHelper>
getFormulaHelper(boost::shared_ptr<LogicalNameMappingBackend> backend);
65 boost::weak_ptr<MathPluginFormulaHelper> _h;
@ float64
Double precision float.
std::map< std::string, std::string > _parameters
Base class for plugins that modify the behaviour of accessors in the logical name mapping backend.
void doRegisterInfoUpdate() override
Implementation of the plugin specific register information update.
bool _allParametersWrittenAfterOpen
Math Plugin: Apply mathematical formula to register's data.
boost::shared_ptr< NDRegisterAccessor< UserType > > decorateAccessor(boost::shared_ptr< LogicalNameMappingBackend > &backend, boost::shared_ptr< NDRegisterAccessor< TargetType >> &target, const UndecoratedParams &accessorParams)
Helper struct to hold extra parameters needed by some plugins, used in decorateAccessor()
void closeHook() override
Hook called when the backend is closed, at the beginning of the close() function when the device is s...
boost::shared_ptr< MathPluginFormulaHelper > getFormulaHelper(boost::shared_ptr< LogicalNameMappingBackend > backend)
if not yet existing, creates the instance and returns it if already existing, backend ptr may be empt...
bool _creatingFormulaHelper
DataValidity
The current state of the data.
static thread_local int64_t _writeLockCounter
RegisterInfo structure for the LogicalNameMappingBackend.
LNMBackendRegisterInfo * info()
LNMBackendRegisterInfo _info
RegisterInfo describing the the target register for which this plugin instance should work.
std::vector< double > _lastMainValue
std::recursive_mutex _writeMutex
A class to describe which of the supported data types is used.
MathPlugin(const LNMBackendRegisterInfo &info, size_t pluginIndex, std::map< std::string, std::string > parameters)
ChimeraTK::DataValidity _lastMainValidity
void openHook(const boost::shared_ptr< LogicalNameMappingBackend > &backend) override
Hook called when the backend is opened, at the end of the open() function after all backend work has ...
void exceptionHook() override
Hook called when an exception is reported to the the backend via setException(), after the backend ha...
bool _enablePushParameters
bool _mainValueWrittenAfterOpen
void postParsingHook(const boost::shared_ptr< const LogicalNameMappingBackend > &backend) override
DataType getTargetDataType(DataType) const override
Return the data type for which the target accessor shall be obtained.
N-dimensional register accessor.