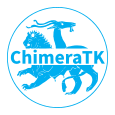 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
5 #include "../DeviceBackend.h"
6 #include "../NDRegisterAccessor.h"
9 #include <ChimeraTK/cppext/finally.hpp>
10 #include <ChimeraTK/cppext/future_queue.hpp>
14 class AsyncAccessorManager;
22 template<
typename UserType>
24 static constexpr
size_t _queueSize{3};
31 AsyncNDRegisterAccessor(boost::shared_ptr<DeviceBackend> backend, boost::shared_ptr<AsyncAccessorManager> manager,
32 boost::shared_ptr<Domain> asyncDomain, std::string
const& name,
size_t nChannels,
size_t nElements,
34 std::string
const& description = std::string());
83 [[nodiscard]]
bool isReadable()
const override {
return true; }
84 [[nodiscard]]
bool isWriteable()
const override {
return false; }
87 this->_exceptionBackend = exceptionBackend;
91 return {boost::enable_shared_from_this<TransferElement>::shared_from_this()};
void doReadTransferSynchronously() override
std::vector< boost::shared_ptr< TransferElement > > getHardwareAccessingElements() override
The AsyncNDRegisterAccessor implements a data transport queue with typed data as continuation of the ...
bool isWriteable() const override
std::list< boost::shared_ptr< TransferElement > > getInternalElements() override
AsyncNDRegisterAccessor(boost::shared_ptr< DeviceBackend > backend, boost::shared_ptr< AsyncAccessorManager > manager, boost::shared_ptr< Domain > asyncDomain, std::string const &name, size_t nChannels, size_t nElements, AccessModeFlags accessModeFlags, std::string const &unit=std::string(TransferElement::unitNotSet), std::string const &description=std::string())
In addition to the arguments of the NDRegisterAccessor constructor, you need an AsyncAccessorManager ...
void replaceTransferElement(boost::shared_ptr< TransferElement >) override
DECLARE_TEMPLATE_FOR_CHIMERATK_USER_TYPES(AsyncNDRegisterAccessor)
~AsyncNDRegisterAccessor() override
boost::shared_ptr< AsyncAccessorManager > _accessorManager
void sendException(std::exception_ptr &e)
Pushes the exception to the queue.
void doPreRead([[maybe_unused]] TransferType type) override
boost::shared_ptr< Domain > _asyncDomain
static constexpr char unitNotSet[]
Constant string to be used as a unit when the unit is not provided or known.
cppext::future_queue< Buffer, cppext::SWAP_DATA > _dataTransportQueue
void sendDestructively(typename NDRegisterAccessor< UserType >::Buffer &data)
You can only send destructively.
void interrupt() override
boost::shared_ptr< DeviceBackend > _backend
bool doWriteTransferDestructively([[maybe_unused]] ChimeraTK::VersionNumber versionNumber) override
TransferType
Used to indicate the applicable operation on a Transferelement.
bool isReadOnly() const override
void setExceptionBackend(boost::shared_ptr< DeviceBackend > exceptionBackend) override
bool doWriteTransfer([[maybe_unused]] ChimeraTK::VersionNumber versionNumber) override
Class for generating and holding version numbers without exposing a numeric representation.
Set of AccessMode flags with additional functionality for an easier handling.
void doPreWrite([[maybe_unused]] TransferType type, [[maybe_unused]] VersionNumber versionNumber) override
bool isReadable() const override
Data type to create individual buffers.
void doPostRead([[maybe_unused]] TransferType type, bool updateDataBuffer) override
N-dimensional register accessor.
Exception thrown when a logic error has occured.