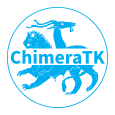 |
ChimeraTK-ControlSystemAdapter-OPCUAAdapter
04.00.01
|
Go to the documentation of this file.
23 #include <libxml2/libxml/tree.h>
24 #include <libxml2/libxml/xpath.h>
25 #include <libxml2/libxml/xpathInternals.h>
27 #include <boost/tokenizer.hpp>
58 bool createDoc(
const std::string& filePath);
72 xmlXPathObjectPtr
getNodeSet(
const std::string& xPathString);
81 static std::vector<xmlNodePtr>
getNodesByName(xmlNodePtr startNode,
const std::string& nodeName);
91 const std::string& variablePath,
const std::string& seperator =
"/");
xmlXPathObjectPtr getNodeSet(const std::string &xPathString)
This methode return a pointer of a xPath element depending of the given xPathString.
~xml_file_handler()
Destructor of the class, frees the document and clean the parser.
This class support any file interaction with a xml file.
static std::vector< std::string > parseVariablePath(const std::string &variablePath, const std::string &seperator="/")
This methode splitt a given string bey the given seperators.
static std::vector< xmlNodePtr > getNodesByName(xmlNodePtr startNode, const std::string &nodeName)
This methode return a list of all nodes with the given name nodeName starting by the given startNode.
static std::string getContentFromNode(xmlNode *node)
This methode returns the value between a xml tag.
xml_file_handler(const std::string &filePath)
The constructor of the class creates a doc pointer depending on the file path.
static std::string getAttributeValueFromNode(xmlNode *node, const std::string &attributeName)
This methode returns a value of the given attribute from the given node you want to know.
bool isDocSetted()
This Methode check if a document is currently setted.
bool createDoc(const std::string &filePath)
This methode set a document pointer to the file it ist given by the file path.