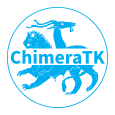 |
ChimeraTK-ControlSystemAdapter-OPCUAAdapter
04.00.01
|
Go to the documentation of this file.
4 #include <boost/test/included/unit_test.hpp>
12 using namespace boost::unit_test_framework;
17 static void testWithoutPVSet();
19 static void testWithPVSet();
23 cout <<
"Enter CSAOPCUATest without any pv" << std::endl;
42 std::cout <<
"Enter CSAOPCUATest with ExampleSet" << std::endl;
This class provide the two parts of the OPCUA Adapter. First of all the OPCUA server starts with a po...
void stop()
Stop all objects in single threads for this case only the opc ua server.
The boost test suite which executes the ProcessVariableTest.
bool isRunning()
Checks if the opcua server is still running and return the suitable bool value.
boost::shared_ptr< ControlSystemPVManager > csManager
static void testWithoutPVSet()
boost::shared_ptr< ControlSystemPVManager > csManager
std::shared_ptr< ua_uaadapter > getUAAdapter()
Return the uaadapter, hence the OPC UA server.
csa_opcua_adapter * csaOPCUA
void start()
Start all objects in single threads for this case only the opc ua server.
static void testWithPVSet()
boost::shared_ptr< ControlSystemPVManager > const & getControlSystemManager() const
Return the ControlsystemPVManager of the class.
test_suite * init_unit_test_suite(int, char **)