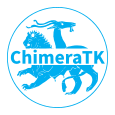 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
36 void addAccessor(
const boost::shared_ptr<TransferElement>& accessor);
101 void runPostReads(
const std::set<boost::shared_ptr<TransferElement>>& elements,
102 const std::exception_ptr& firstDetectedRuntimeError) noexcept;
std::set< boost::shared_ptr< TransferElement > > _copyDecorators
List of all CopyRegisterDecorators in the group.
void write(VersionNumber versionNumber={})
Trigger write transfer for all accessors in the group.
void addAccessor(TransferElementAbstractor &accessor)
Add a register accessor to the group.
bool isReadable()
Check if transfer group is readable.
bool isReadOnly()
Check if transfer group is read-only.
Group multiple data accessors to efficiently trigger data transfers on the whole group.
bool isWriteable()
Check if transfer group is writeable.
void read()
Trigger read transfer for all accessors in the group.
Base class for register accessors abstractors independent of the UserType.
std::map< boost::shared_ptr< TransferElement >, bool > _lowLevelElementsAndExceptionFlags
List of low-level TransferElements in this group, which are directly responsible for the hardware acc...
bool _cachedReadableWriteableIsValid
Flag whether to update the cached information.
std::set< boost::shared_ptr< DeviceBackend > > _exceptionBackends
List of all exception backends.
void updateIsReadableWriteable()
Helper function to update the cached state variables.
bool _isReadable
Cached value whether all elements are readable.
void runPostReads(const std::set< boost::shared_ptr< TransferElement >> &elements, const std::exception_ptr &firstDetectedRuntimeError) noexcept
Class for generating and holding version numbers without exposing a numeric representation.
bool _isWriteable
Cached value whether all elements are writeable.
void dump()
Print information about the accessors in this group to screen, which might help to understand which t...
std::set< boost::shared_ptr< TransferElement > > _highLevelElements
List of high-level TransferElements in this group which are directly used by the user.