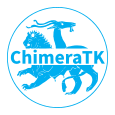 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
8 #include <boost/asio.hpp>
14 namespace ip = boost::asio::ip;
29 unsigned int protocolVersion, ip::tcp::socket socket, std::shared_ptr<DummyBackend> regsiterSpace);
39 static const uint32_t
PONG = 1005;
46 static const uint32_t
HELLO = 4;
47 static const uint32_t
PING = 5;
79 void write(std::vector<uint32_t> data);
84 RebotDummyServer(
unsigned int portNumber, std::string mapFile,
unsigned int protocolVersion);
89 unsigned int port()
const {
return _connectionAcceptor.local_endpoint().port(); }
91 boost::asio::io_service&
service() {
return _io; }
92 std::shared_ptr<RebotDummySession>
session() {
return _currentSession.lock(); }
96 unsigned int _protocolVersion;
97 boost::asio::io_service _io;
98 ip::tcp::acceptor _connectionAcceptor;
99 std::weak_ptr<RebotDummySession> _currentSession;
100 ip::tcp::socket _socket;
101 std::shared_ptr<DummyBackend> _registerSpace;
virtual ~RebotDummySession()
std::unique_ptr< DummyProtocolImplementor > _protocolImplementor
void acceptHandler(const boost::system::error_code &error)
static const int BUFFER_SIZE_IN_WORDS
std::shared_ptr< DummyBackend > _registerSpace
std::atomic< uint32_t > _heartbeatCount
static const uint32_t MULTI_WORD_READ
std::atomic< bool > stop_rebot_server
static const int32_t TOO_MUCH_DATA_REQUESTED
void sendSingleWord(int32_t response)
unsigned int port() const
static const uint32_t PING
static const uint32_t HELLO
static const uint32_t INSIDE_MULTI_WORD_WRITE
static const int32_t READ_SUCCESS_INDICATION
static const uint32_t PONG
std::atomic< bool > _dont_answer
static const uint32_t SINGLE_WORD_WRITE
static const uint32_t MULTI_WORD_WRITE
unsigned int _protocolVersion
boost::asio::io_service & service()
static const int32_t UNKNOWN_INSTRUCTION
ip::tcp::socket _currentClientConnection
RebotDummyServer(unsigned int portNumber, std::string mapFile, unsigned int protocolVersion)
static const int32_t WRITE_SUCCESS_INDICATION
void processReceivedPackage(std::vector< uint32_t > &buffer)
static const uint32_t ACCEPT_NEW_COMMAND
void writeWordToRequestedAddress(std::vector< uint32_t > &buffer)
void write(std::vector< uint32_t > data)
RebotDummySession(unsigned int protocolVersion, ip::tcp::socket socket, std::shared_ptr< DummyBackend > regsiterSpace)
std::shared_ptr< RebotDummySession > session()
std::vector< uint32_t > _dataBuffer
void readRegisterAndSendData(std::vector< uint32_t > &buffer)
std::atomic< uint32_t > _helloCount
static const uint32_t REBOT_MAGIC_WORD