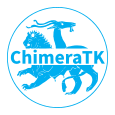 |
ChimeraTK-DeviceAccess
03.18.00
|
Go to the documentation of this file.
10 template<
typename UserType,
typename DataConverterType,
bool isRaw>
11 class NumericAddressedBackendRegisterAccessor;
13 class NumericAddressedBackendASCIIAccessor;
16 template<
typename UserType,
typename DataConverterType,
bool isRaw>
17 struct NumericAddressedPrePostActionsImplementor;
33 const boost::shared_ptr<NumericAddressedBackend>& dev,
size_t bar,
size_t startAddress,
size_t numberOfBytes)
36 if(!dev->barIndexValid(bar)) {
37 std::stringstream errorMessage;
38 errorMessage <<
"Invalid bar number: " << bar << std::endl;
87 bool isMergeable(
const boost::shared_ptr<TransferElement const>& other)
const {
88 if(!
_dev->canMergeRequests())
return false;
91 auto rhsCasted = boost::dynamic_pointer_cast<const NumericAddressedLowLevelTransferElement>(other);
92 if(!rhsCasted)
return false;
93 if(
_dev != rhsCasted->_dev)
return false;
94 if(
_bar != rhsCasted->_bar)
return false;
98 if(
_startAddress > rhsCasted->_startAddress + rhsCasted->_numberOfBytes)
return false;
102 bool mayReplaceOther(
const boost::shared_ptr<TransferElement const>&)
const override {
109 return typeid(int32_t);
136 "is not implemented");
149 auto alignment =
_dev->minimumTransferAlignment(
_bar);
156 if(end_padding != alignment) {
169 boost::shared_ptr<NumericAddressedBackend>
_dev;
194 return {boost::enable_shared_from_this<TransferElement>::shared_from_this()};
201 template<
typename UserType,
typename DataConverterType,
bool isRaw>
204 template<
typename UserType,
typename DataConverterType,
bool isRaw>
friend struct detail::NumericAddressedPrePostActionsImplementor
@ raw
Raw access: disable any possible conversion from the original hardware data type into the given UserT...
~NumericAddressedLowLevelTransferElement() override=default
boost::shared_ptr< NumericAddressedBackend > _dev
the backend to use for the actual hardware access
Implementation of the NDRegisterAccessor for NumericAddressedBackends for scalar and 1D registers.
std::list< boost::shared_ptr< TransferElement > > getInternalElements() override
Obtain the full list of TransferElements internally used by this TransferElement.
void doPostWrite(TransferType, VersionNumber) override
Backend specific implementation of postWrite().
bool _isUnaligned
flag whether access is unaligned
std::vector< boost::shared_ptr< TransferElement > > getHardwareAccessingElements() override
Obtain the underlying TransferElements with actual hardware access.
bool doWriteTransfer(ChimeraTK::VersionNumber) override
Implementation version of writeTransfer().
bool isReadable() const override
Check if transfer element is readable.
uint64_t _startAddress
start address w.r.t.
size_t _numberOfBytes
number of bytes to access
std::string _name
Identifier uniquely identifying the TransferElement.
const std::type_info & getValueType() const override
Returns the std::type_info for the value type of this transfer element.
void setAddress(size_t startAddress, size_t numberOfBytes)
Set the start address (inside the bar given in the constructor) and number of words of this accessor.
uint8_t * begin(size_t addressInBar)
Return pointer to the begin of the raw buffer matching the given address.
void doPreWrite(TransferType, VersionNumber) override
Backend specific implementation of preWrite().
TransferType
Used to indicate the applicable operation on a Transferelement.
bool isWriteable() const override
Check if transfer element is writeable.
std::unique_lock< std::mutex > _unalignedAccess
Lock to protect unaligned access (with mutex from backend)
std::vector< uint8_t > rawDataBuffer
raw buffer
Implementation of the NDRegisterAccessor for NumericAddressedBackends, responsible for the underlying...
Base class for register accessors which can be part of a TransferGroup.
bool mayReplaceOther(const boost::shared_ptr< TransferElement const > &) const override
Check whether the TransferElement can be used in places where the TransferElement "other" is currentl...
void doPostRead(TransferType, bool hasNewData) override
Backend specific implementation of postRead().
void doReadTransferSynchronously() override
Implementation version of readTransfer() for synchronous reads.
Class for generating and holding version numbers without exposing a numeric representation.
void replaceTransferElement(boost::shared_ptr< TransferElement >) override
NumericAddressedLowLevelTransferElement(const boost::shared_ptr< NumericAddressedBackend > &dev, size_t bar, size_t startAddress, size_t numberOfBytes)
boost::shared_ptr< TransferElement > makeCopyRegisterDecorator() override
Create a CopyRegisterDecorator of the right type decorating this TransferElement.
void changeAddress(size_t startAddress, size_t numberOfWords)
Change the start address (inside the bar given in the constructor) and number of words of this access...
std::string to_string(Boolean &value)
bool isShared
flag if changeAddress() has been called, which is this low-level transfer element is shared between m...
Implementation of the NDRegisterAccessor for NumericAddressedBackends for ASCII data.
uint64_t _bar
start address w.r.t.
bool isMergeable(const boost::shared_ptr< TransferElement const > &other) const
Check if the address areas are adjacent and/or overlapping.
bool isReadOnly() const override
Check if transfer element is read only, i.e.
Exception thrown when a logic error has occured.
VersionNumber _versionNumber
The version number of the last successful transfer.